What is Dangling Pointer?
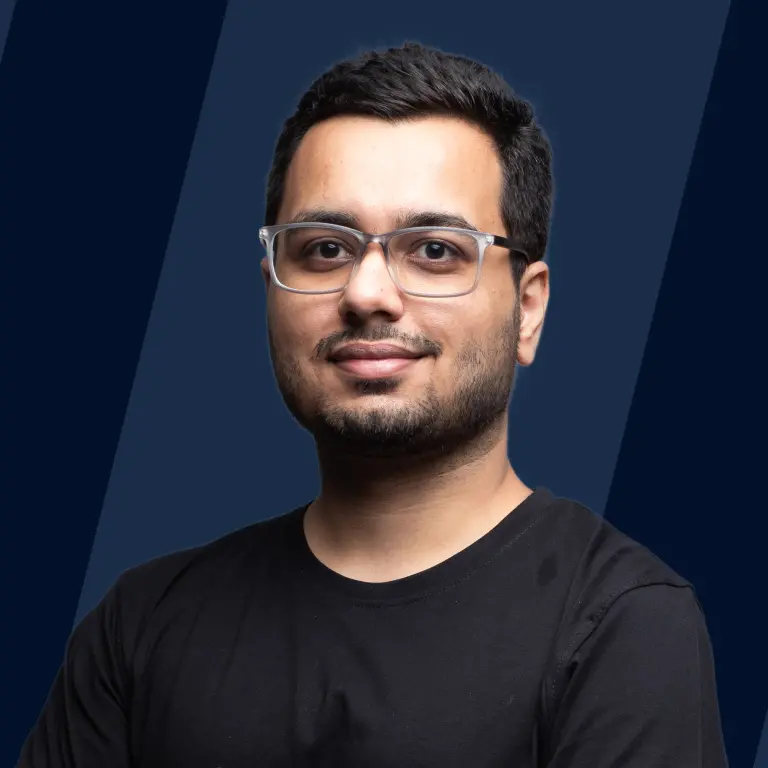
A dangling pointer is the most common pointer in C++. This type of pointer also causes a problem in the memory management of a system. When we create a program, we use a pointer to point to an object. But sometimes, we do not change the pointer's value, and we free the object from the memory block. In this situation, the pointer becomes a dangling pointer. A dangling pointer is a type of pointer that comes when we de-allocate the object but forget to modify the pointer's value. In this article, we will discuss the dangling pointer with details examples, explanations, and illustrations where ever necessary.
- A dangling pointer does not point to valid data or useless data. The dangling pointer happens when the user does not delete the memory allocated for an object in the past. The dangling pointer error is also responsible for crashing a program. This happens when the two pointers point to the same memory address, and any one of the two pointers tries to access them.
A dangling pointer is also known as Memory Leak. This is because the dangling pointer leaks memory from memory Heap. A heap is defined as the memory region that can be allocated and de-allocated, and the dynamic data resides in this region.
- Example :
Output :
Explanation :
In the above example, we can see a dynamic memory location allocated to the pointer variable and the value stored in it that is 80. After that, the dynamic memory is deleted using the delete operator. Here, the pointer points to the dynamic memory. And this is the Dangling Pointer.
What are the Different Ways a Pointer can Act as a Dangling Pointer in C++?
Three situations in which a pointer can act as a dangling pointer in c++ are given below. All three situations are described below using the implementation in c++ language and explaining each.
Deallocation of Memory
Explanation: In the above example, we can see there is deallocation of the memory block is done, and after that, the pointer becomes become the dangling pointer. First, the pointer is assigned to the int. In this, the malloc() function is used for reserving a block of memory. After that, a free call is made to the pointer so that the pointer becomes the dangling pointer. And again, the value of the pointer is set to NULL, after which the pointer again becomes a normal pointer.
Function Call
Output :
Explanation :
In the above example, a function named function_1 is created, and the pointer is assigned. Inside the function, a variable a is defined as an int type whose value is 6. Now, in the main function, an integer value is assigned to the function funct. And the output is printed using the cout. In this code, the local variable goes out of scope. In the main function, the pointer points to the element that is not valid.
So, the pointer does not become dangling if the variable a is the static variable. Now,
Output:
Explanation :
Inside the function local_funct, a static variable a is created, and the value 5 is assigned to it. In return, the address of a is assigned. Inside the main function, the pointer value is printed as output using the cout.
Variable Going Out of Scope
Explanation :
In the above example, the pointer ptr is assigned to the int inside the main function. As we can see, when the control will not present inside the inner scope, the variable will be unavailable for access, and hence, the variable becomes a dangling pointer.
Examples Related to Dangling Pointer in C++
Now let us see examples related to a dangling pointer in C++.
- Example 1. In this example, we will use dangling pointer to delete the variable.
Explanation : In the above program, there is the main function. Inside this, we have declared the pointer int type and assigned it to new int. After that, we used a pointer to assign the value 80 to the pointer. In the next line, we deleted the pointer using the delete function and finally print the output of the program.
- Example 2. In this example, we use typecasting for referencing the variables and manipulating the variables using a dangling pointer in C++.
Output :
Explanation :
In this example, we have initialized the x variable with an integer value. Then we also initialized a variable y whose data type is float. Then we declared a void pointer, namely p. After that, we did typecast for (int*)p. Then we assigned &y to p. Now, the pointer p becomes a float type. Again we do the same typecasting for (float*)p. And finally, we print the output.
How to Avoid Dangling Pointers?
Following are the methods by which we can avoid dangling pointers in c++.
Null Assignment After Memory Deallocation.
In some cases, we need to free the memory and reset the variable's value to NULL. If we set the unused pointer to the value NULL, it will be a defensive style because it protects against the bugs of dangling pointers. When we access the dangling pointer after it is freed, we can also read or overwrite the random memory. Initializing the pointers to the value NULL is better before they get a true value for the pointer.
Static Variables with Global Scope.
A static variable is a variable that can only be accessed when the variable is created in the file. This variable is also known for having file scope.
Smart Pointers
Smart pointers are a special type of pointer in C++ used to make sure that an object is deleted if it is no longer used. It is a wrapper class that wraps (encloses) a raw (or bare) C++ pointer to avoid any memory leak. Smart pointers are the pointers that make sure that the acquired memory is released if an object is no longer needed. Smart pointers are used to perform automatic memory management.
Types of Smart Pointers in C++ :
The smart pointers can be of three types in C++ :
-
unique_ptr: The unique pointers only store one value at a time. These pointers cannot be copied to another pointer. The unique_ptr prevents the copying of contained pointers.
-
shared_ptr: The shared pointers contain raw pointers. A shared_ptr can contain a copied object, or it is also possible that it may not contain any object. When we initialize a shared_ptr, we can copy and pass the value to another pointer. When we use shared_ptr, one object is pointed by more than one pointer simultaneously, and the shared_ptr will maintain a reference counter.
-
weak_ptr: The weak_ptr pointer is almost the same as shared_ptr, but one thing that makes it different is that it does not maintain any reference counter. This pointer gets in dilemma whether to hold a value or de-allocate it. This situation may form a Deadlock.
Learn More :
You can also click here to learn more about Dangling Pointers in C
Conclusion
- A dangling pointer in C++ is defined as when a user allocates the object but forgets to update the pointer's value, then the pointer becomes a Dangling Pointer.
- A Dangling Pointer is also known as Memory Leak.
- A heap is defined as the memory region that can be allocated and de-allocated, and the dynamic data resides in this region.
- A dangling pointer in c++ can be seen in three different conditions- Deallocation of memory during a Function Call and when the Variable goes out of scope.
- Dangling pointers in C++ can be avoided by the given methods: Null assignment after Memory Deallocation, Static variables with global scope, and using Smart pointers.
- The Smart pointers are a special type of pointer in C++ that is used to make sure that an object is deleted if it is no longer used.