Data Structures in Java | Beginners Tutorial 2023
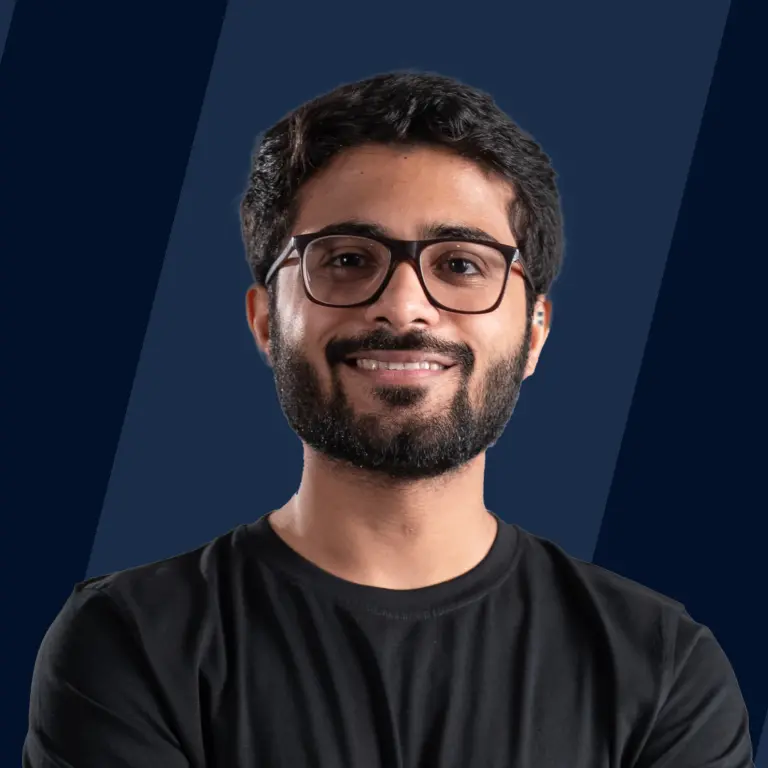
Overview
Data structure is a method of storing and organizing data to make it more useful. The data structure is not written in any programming language, such as C, C++, or Java. It is a set of methods that may be used to structure data in memory in any programming language.
What are Data Structures in Java?
The term data structure refers to a collection of data with well-defined operations, behavior, or properties. A data structure is a special manner of storing or organizing data in computer memory so that we can use it effectively.
A real-life example can be that data structure is used in a drive-in burger joint, to keep track of customer orders. (Customers continue to arrive, and they must obtain the correct meal at the payment/collection window.)
Data Structures are used extensively in practically all areas of computer science, including computer graphics, operating systems, artificial intelligence, compiler design, and many more.
Need for Data Structures in Java
The use of data structures is necessary to solve various problems generated because of the rapid increase in the amount of data, which makes the applications more complex.
- Reasonable Processor Speed: The faster a computer's CPU works, the more data it can handle. If data is not effectively structured into a data structure, the processor may not be able to keep up.
- Data Searchable: We've all glanced at a long list of statistics or an intimidating spreadsheet and wondered how we'll ever find what we're looking for. Search functions are incorporated straight into data structures, allowing us to find what we need fast and easily.
- Handle high levels of requests for data: Clients of a corporation may appear to request data all at once, and without data structures in place, this might cause a server to crash. Computers can handle these many requests more effectively thanks to data structures.
Don't miss out on this opportunity to become a Java DSA expert. Enroll in our free Java DSA course now & gain the skills to ace job interviews & coding challenges.
Advantages of Data Structures in java
Advantages of Data Structures in Java:
- Efficient organization and storage of data: Data structures in Java provide efficient ways to organize and store data. They enable the representation of complex data relationships and allow for easy access, retrieval, and modification of data elements using data structures in Java.
- Improved time and space complexity for operations: Data structures in Java are designed to optimize the performance of common operations such as searching, insertion, deletion, and sorting. By leveraging appropriate data structures in Java based on the specific requirements of your application, you can achieve improved time and space complexity, resulting in faster and more efficient operations with data structures in Java.
- Enhanced data manipulation capabilities: Data structures in Java offer a wide range of operations and methods for manipulating data. They provide functionalities such as sorting, filtering, merging, and searching, making it easier to perform complex data manipulations using data structures in Java.
- Flexibility and adaptability: Java provides a rich set of built-in data structures such as arrays, lists, queues, stacks, trees, and graphs. Additionally, Java allows for the creation of custom data structures through object-oriented programming principles.
This flexibility with data structures in Java enables developers to choose the most suitable data structure for their specific needs and adapt it as requirements change over time.
Data Structure Classification in Java
- Linear Data Structures: It is a single level data structure in which all elements are arranged in sequential order.
- Non-Linear Data Structures: These are multi-level data structures in which data is not arranged in sequential order.
1. Arrays
Arrays are collections of data elements of comparable types stored in contiguous memory locations. It's one of the simplest data structures, with each data element accessible at random using its index number.
Syntax in Java:
Implementation Example
Advantages of Arrays
- Arrays use a single name to represent numerous data elements of the same type.
- The elements in arrays can be accessed at random using the index number.
- For all of its elements, arrays allocate memory in contiguous memory regions. As a result, there is no probability of extra memory being allocated. This prevents memory overflow and shortages in arrays.
- Other data structures, such as linked lists, stacks, queues, trees, and graphs, can be implemented using arrays.
Disadvantages of Arrays
- The number of elements to be stored in an array should be known in advance.
- Once the size of the array is declared, it cannot be modified. Memory allocated cannot be increased or decreased. Thus array is a static structure.
- Allocating more memory than several elements leads to wastage of memory. Less memory allocation leads to a problem.
- As the items are stored in consecutive memory regions and the shifting process is expensive, insertion and deletion are difficult in an array.
To learn more about array data structure refer here
2. Linked Lists
The LinkedList class, just like the ArrayList, is a collection that can hold numerous items of the same type. Here the elements are not stored in contiguous locations and each element is a separate object with a data part and address part. Each element is known as a node and is linked using pointers and addresses.
Linked List is a part of the Collection framework present in java.util package.
Syntax
Implementation Example
Output
Advantages of Linked List
- The linked list is a dynamic data structure. We can allocate and deallocate the memory at run-time itself.
- The node can be easily inserted or deleted using the insertion and deletion function.
- The linked list makes good use of memory. Because we don't have to allocate memory ahead of time.
- It has an extremely rapid access time and can be accessed at a specific time without any memory overhead.
- Linear data structures like stack and the queue can be easily used linked list.
Disadvantages of Linked List
- As each node of the linked list points to a pointer, it requires more memory to store the elements than an array.
- Traversing the nodes of a linked list is quite tough. We won't be able to access any node at random in this case.(As we do in the array by index.)
- In a linked list, reverse traversing is harder since the pointer demands extra memory.
To learn more about linked list data structure refer here
3. Stack
A stack is a Last In First Out (LIFO) data structure that can be implemented physically as an array or a linked list. It simply has one pointer, top, which points to the stack's topmost element. When a new element is added to the stack, it is placed at the top, and the element can only be removed from the stack. To put it another way, a stack may be thought of as a container in which insertion and deletion can be done from one end.
Syntax
Implementation Example
Output
Advantages of Stack
- Stack manages the data in a LIFO method, which is not possible with a linked list and array.
- Local variables are stored in a stack when a function is called, and automatically get destroyed once returned.
- You can use Stack to manage how memory is allocated and deallocated.
- Stack is more secure and reliable since it does not easily get corrupted.
- Stack does not allow resizing of variables.
Disadvantages of Stack
- Stack overflow can occur if you create too many objects on the stack.
- When variable storage is overwritten, the function or programme can sometimes behave in an unpredictable manner.
- Stack memory is limited.
- Data cannot be randomly accessed in a stack.
To learn more about stack data structure refer here
4. Queue
A queue is a data structure that follows the FIFO (First-In-First-Out) principle, in which elements are added to the end of the list and removed from the beginning.
Syntax
Few implementations which can be used
Implementation Example
Output
Advantages of Queue
Queues have the benefit of being able to handle a variety of data types while also being flexible and quick. In addition, queues have the potential to be infinitely long, as opposed to fixed-length arrays.
Disadvantage of Queue
A major disadvantage of a classical queue is that a new element can only be inserted when all of the elements are deleted from the queue.
To learn more about queue data structure refer here
5. Graph
A graph is a data structure for storing connected data like a network of people on a social media platform. Vertices and edges make up a graph. The entity (for example, people) is represented by a vertex, and the relationship between entities (for example, a person's friendships) is represented by an edge.
Syntax
Implementation
Output
Advantage of Graph
The fundamental benefit of a graph data structure is that it allows you to use all graph-related computer science algorithms. You can use all of the power of graph algorithms to solve your problem once you've worked out how to represent your domain logic as a graph.
Disadvantage of Graph
The main disadvantage is its large memory complexity.
To learn more about graph data structures refer here
6. Set
A collection of unique elements is termed a set. Java offers a set interface that can be used to store distinct values. In the java.util package, there is an interface called to set. The Collection interface is extended by the Set interface. A collection interface is an unordered collection or list in which duplicates are not permitted. The mathematical set is created using the set interface. To prevent the insertion of the identical elements, the set interface makes use of the collection interface's methods. Two interfaces that extend the set implementation are SortedSet and NavigableSet.
Syntax
Implementation
Output
Advantages of Set
- Set guarantees there is no duplicate object in it.
- Sets typically guarantee access times of O(logN).
To learn more about set data structure refer here
7. Map
In Java, there is a map interface. A mapping between a key and a value is represented by the util package. The Collection interface is not a subclass of the Map interface. As a result, it operates differently than the other collection types. A map has its own set of keys.
Syntax
Implementation example
Output
Advantages of Map
- If all you care about is lookup look you know your keys (what you're using to look up your data) fall into a fairly small integer range, an array (or, better yet, a vector) is great. With minimal fuss, you can look something up using a key in real-time.
- map stores items in key order. So you can iterate over all the items in a map, from beginning to end, in sorted key order. You obviously can do this with an array/vector, how, ever as was said earlier, a map lets you have any arbitrary key type with any arbitrary ordering defined.
- To insert into the middle of an array/vector, all of the objects must be shifted to the left. If you have a dynamic array and a vector, you must enlarge the vector, which will cause the entire array to be copied to fresh memory.
Disadvantages of Map
- While hash-tables have constant time insertion, they will need to evolve their internal structure and re-bucket their entries periodically. This is an operation with a cost proportionate to the hash-current table's size. As a result, insertion time does not always follow a consistent pattern.
- Hash-tables, in general, do not preserve ordering, whether natural or insertion order.
Shape Your Coding Future! Join Our Data Structures Course and Navigate the World of Efficient Algorithms. Enroll Now!
Conclusion
- The term data structure refers to a collection of data with well-defined operations, behavior, or properties.
- Use of data structures is necessary to solve various problems generated because of the rapid increase in the amount of data, which makes the application more complex.
- Linear Data Structures are single-level data structures in which all elements are arranged in sequential order whereas non-linear data structures are multilevel data structures in which data is not arranged in sequential order.
- Arrays are collections of data elements of comparable types stored in contiguous memory locations.
- The LinkedList class, just like the ArrayList, is a collection that can hold numerous items of the same type. Here the elements are not stored in contiguous locations and each element is a separate object with a data part and address part.
- A stack is a Last In First Out (LIFO) data structure that can be implemented physically as an array or a linked list.
- A queue is a data structure that follows the FIFO (First-In-First-Out) principle, in which elements are added to the end of the list and removed from the beginning.
- A graph is a data structure for storing connected data like a network of people on a social media platform.
- A collection of unique elements is termed a set. Java offers a set interface that can be used to store distinct values.
- In Java, there is a map interface. The Collection interface is not a subclass of the Map interface. As a result, it operates differently than the other collection types. A map has its own set of keys.