How to Create a Date Object in Javascript?
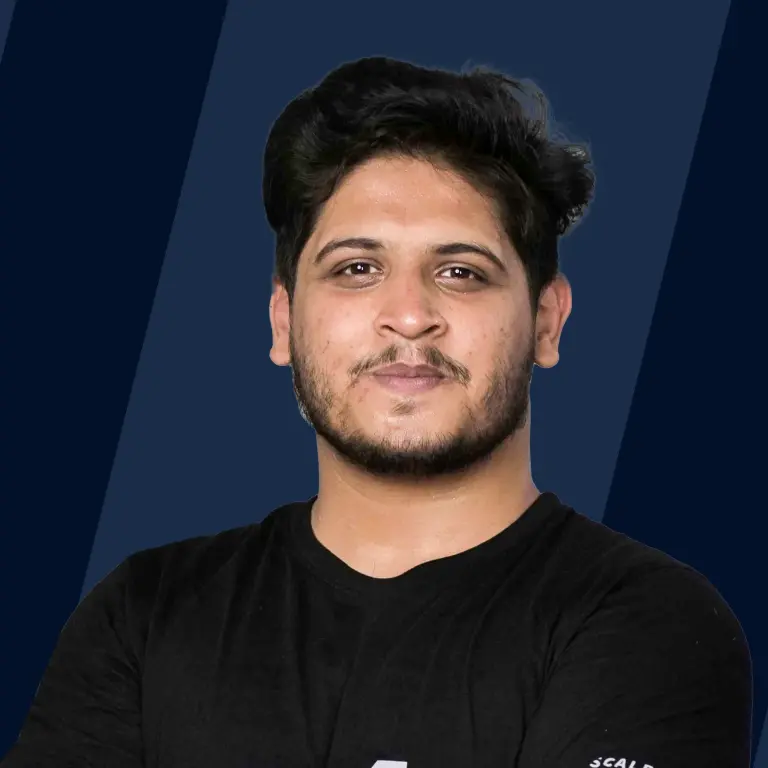
To create a date object in javascript, we have to call the Date constructor. As a result, it returns the date object having a current date(mm/dd/yyyy), and time(Hrs: min: sec).
As an example, we called the Date constructor to make a date object-
Output-
Above, we called the Date constructor and assigned the return object in the myDate variable, then logged myDate on the console. As a result, we printed "Mon Jul 18 2022 18:08:22 GMT+0530 (India Standard Time)" on the console, which is the date and time when the code is executed.
In the above example, we called the Date constructor without passing any argument, but we have other different ways.
4 Variants of Date Constructor to Create a Date Object
There are four ways to create a date object using the Date constructor in javascript.
Date()
Calling the Date constructor without any passing any parameter returns a new date object having values of current date and time-
Output-
Above, we call the Date constructor without passing any parameter and assigned the return value in a variable currentDate. Then, we logged the currentDate on the console by applying toDateString method, as a result, it prints "Fri Jul 22 2022 16:17:36 GMT+0530 (India Standard Time)" on the console.
Date(milliseconds)
Javascript stores the date in a number of milliseconds since January 01, 1970, 00:00:00 UTC, till now it's 1658230119890 milliseconds(constantly running) from the January 01, 1970.
When we call the Date constructor by passing milliseconds as a parameter, it returns the time and date considering January 01, 1970, 00:00:00 UTC starting value of time and date, ie- given value of millisecond will be converted in time and date and will be added after the January 01, 1970, 00:00:00 UTC.
As an example, we are calling the Date constructor by passing 86 400 000 milliseconds(24 hours) as a parameter to add 24 more hours-
Output-
Above, we called the Date constructor by passing the milliseconds as a parameter, then assigned the return object in myVal and logged it on the console. As a result, it prints "Fri Jan 02 1970 05:30:00 GMT+0530 (India Standard Time)" on the console because we passed the 24 hours(in milliseconds) as a parameter.
Note: One day (24 hours) = 86,400,000 milliseconds.
Date(dateString)
To make a date object have a custom date and time, we call the Date constructor by passing the date(month: date: year) and time(hrs: min: sec) as parameters.
As an example, we called the Date constructor by passing the dateString(date and time as string) as a parameter to create a custom date object-
Output-
Above, we called the Date constructor by passing the dateString as a parameter and assigned the return value in myVal variable, then logged the myVal on the console. As a result, it prints "Tue Mar 23 2010" on the console because we passed the custome date and time as a parameter.
Date(year, month, day, hours, minutes, seconds, milliseconds)
To make a date object have a custom and more specific date and time we call the Date constructor by passing the year, month, day, hours, minutes, seconds, and milliseconds as parameters(separated by a comma).
As an example, we called the Date constructor by passing the year, month, day, hours, minutes, seconds, and milliseconds to make a date object having custom and more specific date and time-
Output-
Above, we called the Date constructor by passing the parameters and assigned the return in myVal variable, then logged myVal on the console. As a result, we printed "Fri Nov 23 2012 10:25:40 GMT+0530 (India Standard Time)" on the console.
JavaScript Date Methods
We use the Date constructor to make date objects, but there are several methods defined inside the Date constructor, each time a date object is created inherits the predefined methods into the Date constructor.
These methods are used to perform various operations upon date object, for example- we use the getFullYear to get the year as a four-digit number and setFullYear to set the year.
In the table below, we have given a list of the following methods:
Methods | Description |
---|---|
toString() | Convert the date into a string. |
toUTCString() | Convert the date into UTC. It's a standard to display date. |
toDateString() | Convert the date into a more readable format. |
toISOString() | Convert the date object into a string using the ISO standard formate |
getFullYear() | Used to get the year as a four-digit number (0000) |
getMonth() | Used to get the month as a number (0-11) |
getDate() | Used to get the day as a number (1-31) |
getHours() | Used to get the hour (0-23) |
getMinutes() | Used to get the minute (0-59) |
getSeconds() | Used to get the second (0-59) |
getMilliseconds() | Used to get the millisecond (0-999) |
getDay() | Used to get the weekday as a number (0-6) |
getTime() | Get the time (milliseconds since January 1, 1970) |
setDate() | Used to set the day as a number (1-31) |
setFullYear() | Used to set the year (month and day are optional) |
setHours() | Used to set the hour (0-23) |
setMilliseconds() | Used to set the milliseconds (0-999) |
setMinutes() | Used to set the minutes (0-59) |
setMonth() | Used to set the month (0-11) |
setSeconds() | Used to set the seconds (0-59) |
setTime() | Used to set the time (milliseconds since January 1, 1970) |
Examples
We are taking some examples to understand how to make a date object in javascript:
JavaScript Date Example
In this example, we will get the today date by calling the getDate method on a date object-
Output-
Above, we called the getDate method on the date object and logged it on the console. As a result, we print 20 (today's date) on the console.
JavaScript Current Time Example
In this example, we will get the current time by calling the getTime method on a date object-
Output-
Above, we called the getTime method on the time object and logged it on the console. As a result, we print 1658257721118 on the console. The reason behind this is getTime method gets the time which in Javascript stored in a number of milliseconds since January 01, 1970, 00:00:00 UTC, till now it's 1658257721118 milliseconds(constantly running) from the January 01, 1970.
JavaScript Digital Clock Example
In this example, we will make a digital clock by calling the getHours, getMinutes, and getSeconds methods on a date object-
Output-
Above, we called the getHours, getMinutes, and getSeconds methods on time objects using string literals and logged them on the console simultaneously. As a result, we print "0:54:27" on the console because getHours, getMinutes and getSeconds methods get the hours, minutes, and seconds from the date object.
Conclusion
- To create a date object in javascript, we have to call the Date constructor.
- There are four variants of the Date constructor to create a date object.
- Calling the Date constructor without any parameter returns a date object having a value of the current date and time.
- Calling the Date constructor by passing milliseconds as a parameter returns a date object having date and time, which is the result of adding a millisecond after January 01, 1970, 00:00:00 UTC .
- Calling the Date constructor by passing dateString returns a date object having a custom date and time.
- Calling the Date constructor by passing years, months...milliseconds as parameters returns a new date object having a custom and more specific date and time.