Java Program to Convert Decimal to Binary
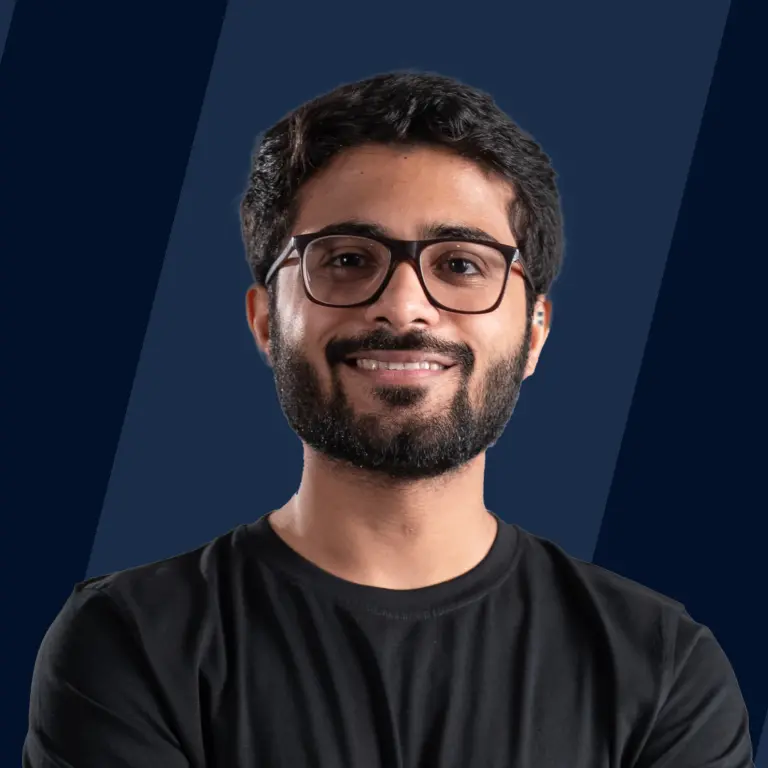
Overview
A number system is a structured way of representing quantities using symbols or digits. Different number systems are based on specific rules and conventions that dictate how numbers are formed, organized, and manipulated. The decimal (base-10) and binary (base-2) systems are the two most commonly used number systems. This article will discuss how to convert decimal to binary in Java. Let's dive right in!
Introduction to Binary and Decimal Number System
The decimal (base-10) and binary (base-2) systems are the two most commonly used number systems.
Decimal System: The decimal system, also known as base-10, is the standard number system used by humans. It employs ten digits (0-9) and operates on the principle of place value, where the position of a digit within a number determines its value relative to powers of ten. Each place represents a multiple of ten, allowing us to represent both whole numbers and fractions.
Binary System: The binary system, also known as base-2, is fundamental in digital computing and electronics. It utilizes only two digits, 0 and 1, to represent all numbers. Similar to the decimal system, the position of a digit in a binary number determines its value, but each position represents a power of two. Binary numbers are essential in representing electronic signals and data storage within computers and other digital devices.
Methods for Decimal to Binary Conversion
Using Bitwise Operators
Learn more about Bitwise operators in Java, Bitwise Operators in Java.
Now, let us see how to convert decimal to binary in Java using Bitwise operators.
Code:
Output:
Complexity of the above method:
Explanation:
This method checks every single bit of number and prints it bit by bit.
Using Java Integer.toBinaryString() Method
Here is the code to convert decimal numbers to binary using Java's Integer.toBinaryString() method. This is a built-in method in Java that directly returns the binary representation of a decimal number as a String.
Code:
Output:
Complexity of the above method:
Using Arrays
To learn more about Arrays in Java, please visit here.
Now let us see how to convert decimals to binary in Java using arrays.
Code:
Output:
Complexity of the above method:
Explanation:
- The decimalToBinaryArray() method takes a decimal input and returns an array of binary digits.
- It creates a 32-sized binaryArray to store binary digits.
- A loop stores remainders as binary digits and divides the decimal by 2.
- Binary digits are returned in reverse order to get the binary representation of the decimal number.
Using Match.pow() Function
Now, let us see how to convert decimal to binary in Java using the Match.pow() Function.
Code:
Output:
Complexity of the above method:
Explanation:
- The function decimalToBinary() takes an integer N (the decimal number to be converted) as input and returns an integer (binary equivalent).
- The loop continues Inside the while loop until N becomes 0. This loop iterates through the decimal number, bit by bit.
- Within the loop, remainder is calculated using N % 2. This remainder is the current binary digit (0 or 1) for the least significant bit.
- Then powerOfTen is calculated using Math.pow(10, exponent). It correctly positions the current binary digit in the binary representation.
- The remainder is added to the binaryNumber after multiplying it by the appropriate powerOfTen. This effectively appends the current binary digit to the binary representation.
- N is then divided by 2 , shifting it to the right by one-bit position. This discards the least significant bit and prepares the loop for the next iteration.
- The exponent is incremented to keep track of the bit position. It calculates the appropriate powerOfTen for the next iteration.
- The loop continues until N becomes 0, when the entire decimal number has been converted to its binary equivalent.
- The binary number is returned as a result.
FAQs
Q. How do I convert decimal to binary?
A. To convert decimal to binary, repeatedly divide the number by two and note down the remainder in reverse order.
Q. What's the significance of the least significant bit in converting decimal to binary in Java?
A. The least significant bit represents the units placed in the binary number and corresponds to the remainder when dividing by 2.
Q. Are there built-in functions to convert decimal to binary in Java?
A. Yes, programming languages often provide functions like Integer.toBinaryString() to convert decimals directly to binary.
Conclusion
Here are the key takeaways from this article on how to convert decimal to binary in Java:
- To convert decimal to binary in Java, divide the decimal by 2 iteratively, taking the remainder as binary digits and writing them in reverse order. Due to logarithmic iterations, complexity is O(log N) for both time and space.
- You can use bitwise operators to convert decimal to binary in Java. Utilize shifts and masks to access binary bits efficiently. With fixed 32 iterations for 32-bit integers, time and space complexities are constant, O(1).
- You can use built-in method Integer.toBinaryString() to convert decimal to binary in Java. Time and space complexities are O(log N).
- You can use Arrays to convert decimal to binary in Java. Opt for arrays to store binary digits systematically. Time and space complexities remain O(log N).
- You can use Math.pow() to convert decimal to binary in Java. Rely on Math.pow() to determine binary digit positions. While time and space complexities are O(log N), the process clarifies bit positioning.
- Varying methods offer trade-offs between efficiency and readability. Bitwise is optimal but less intuitive, while built-in functions and arrays maintain clarity at slightly higher costs. You can choose based on context and priorities.