What is Default Argument in C++?
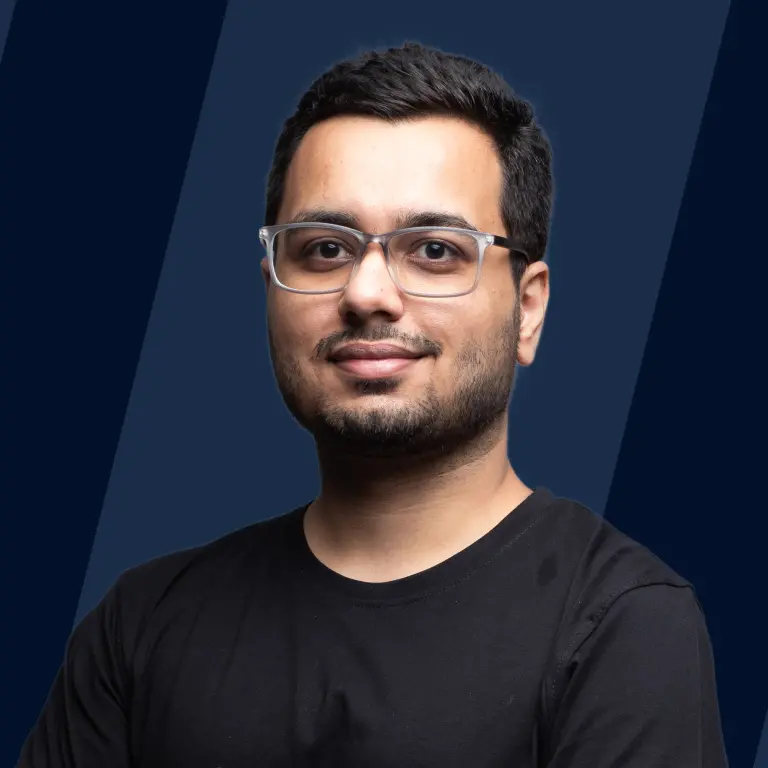
Introduction
Arguments in C++ are the values of parameters passed to a function while calling the function. While writing functions in C++ programming that take arguments as function parameters, we can assign default values to those arguments during the function declaration. These default values assigned to the function parameters are known as default arguments in C++. If the function requiring parameters gets called without any arguments, it uses the default arguments, which are assigned automatically by the compiler at the time of compilation. However, the default arguments get ignored when we call the function by passing the required arguments.
Let's understand the default argument in C++ in detail with this article.
Characteristics for Defining the Default Argument in C++
The characteristics of Default Argument in C++ are as follows.
- The values associated with the default arguments in C++ are not constant. We can use these values only when the function gets called without the appropriate arguments. Otherwise, the declared values get overwritten by the passed values.
The below example shows that the value of default arguments is not constant. Here, we did not use the default argument of b as it gets passed during the function call. The variable c gets its default value as no other argument passes during the function call.
- All the arguments with default values get declared to the right in the list.
- The default arguments get copied from left to right during a function call.
Example
This example shows us the correct way of writing a default argument in the parameters list.
- void function(int x, int y, int z = 0) Explanation: In the above function, we have three parameters x, y, and z. z has a predefined value of 0, which is the default argument. Here, we have declared z to the right in the list of parameters. Thus, the above function is valid.
- void function(int x, int z = 0, int y) Explanation: In the above function, we have three parameters x, y, and z. z has a predefined value of 0, which is the default argument. Here, we have declared z between the parameters x and y, which is not accepted. Thus, the above function is invalid.
Rules of Default Arguments
In the above example, we have defined three different cases. In the first case, we have passed only one argument in the function call. The variables b and c get their values from the default arguments. In the second case, we pass two arguments in the function call. The arguments passed get assigned to the variables a and b. The variable c gets its value from the default argument. In the third case, we pass three arguments in the function call. The passed arguments get assigned to all three variables a b, and c. None of the variables get their default argument.
Working of Default Argument in C++
Let us understand the working of default arguments in C++ using the below example.
- When we perform the function call temp(), the function uses both the default parameters as none of the values passed. Thus the value of i is equal to 10, and f is equal to 8.8.
- When we perform the function call temp(6), it substitutes the first argument's value as 6, and the second parameter takes up the default value. Thus the value of i = 6 and f = 8.8.
- When we perform the function call temp(6, -2.3), the values of both parameters get substituted with their respective default arguments. Thus the value of i = 6 and f = -2.3.
- When we perform the function call temp(3.4), it causes ambiguity as the passed value is float. Since we cannot pass the second argument before the first argument, thus 3.4 is passed as the first argument. Since the data type of the first argument is int, the actual value passed to the first argument is 3.
More Examples of Default Arguments in C++
Let us understand the usage of default arguments in C++ with the help of examples.
Example 1
Output
The above code shows the usage of default arguments in C++. We have only a single function addressing three different statements in the main. It considers the default values of the third and fourth arguments (z and w).
Statement 1 calls the function sum with only two arguments. While compiling the statement from left to right, the values of x and y are assigned 10 and 15. The variables z and w get their default value, zero. Thus, the result of statement 1 is 10+15+0+0 = 25.
Statement 2 calls the function sum by passing three values. While copying the value of the variables from left to right, we assign the value of x,y, and z as 10, 15, and 25, respectively. The variable z overrides its value with 25. The variable w gets its default value, zero. Thus the result of statement 2 is 10 + 15 + 25 = 50.
Statement 3 calls the function sum by passing all four values. While copying the value of the variables from left to right, we assign the value of x, y, z, and was 10, 15, 25, and 30, respectively. The variables z and w override their value with 25 and 30. The default values get ignored as the function gets called by passing all the values. Thus the result of statement 3 is 10 + 15 + 25 + 30 = 80.
Example 2
Output
The above code shows us the errors caused while using default arguments in C++ while there is function overloading. While overloading a function containing default arguments, the compiler throws an error to the program if it finds it ambiguous. In the above program, the function call sum(10,15) checks for the values in both overloaded functions. Since both the function declaration have default arguments of z and w, the compiler finds it hard to decide which one to choose and thus throws an error.
Example 3
Explanation
In the above code, we see the use of default arguments in C++ in the constructor. A class can have multiple constructors with zero or more arguments. The above code has class A with two constructors - One with no argument and the other with one default argument. The constructor with one argument has a default parameter x assigned the zero.
Restrictions on Default Arguments in C++
- Among all the operators, only two operators, namely the function call operator and the new operator, can have default arguments during overloading.
- During the function declaration, the parameters with default arguments must exist at the trailing end of the parameters list, i.e., to the right of the list.
- We cannot use local variables as values in default argument expressions.
For the above C++ code, the compiler gives an error for both g() and h() functions.
Advantages and Disadvantages of Default Arguments
Advantages of Default Arguments in C++
- Use of default arguments in C++ increases the capabilities and the reusability of the code of an existing function. We can consider or ignore the default arguments depending on the number of values passed during the function call.
- The reusability of the same function, again and again, reduces the length of the program.
- It makes the solution approach simple and effective.
- It helps us improve the performance and consistency of the program.
Disadvantages of Default Arguments in C++
- The compiler needs more time to execute the program. It uses the extra time to replace the remaining arguments with their default values during the function call.
Learn More
You can also refer to similar articles on related topics like:
- Functions in C++
- Inline Functions in C++
- Templates in C++
- Templates and default arguments
- Function Overloading in C++
- Default arguments and Virtual functions in C++
- To learn more about C++ properties, visit Scaler Topics.
Conclusion
- Default arguments in C++ are the default values assigned to the function parameters.
- The default arguments are assigned to the right side, i.e., the trailing end of the parameter list.
- The passed values are assigned from left to right in the parameter list.
- It makes the program more reusable, simple, and effective. It makes the program more consistent and efficient.
- The disadvantage of the default argument in C++ is the increase in the execution time of the program.