What is Defaultdict Python?
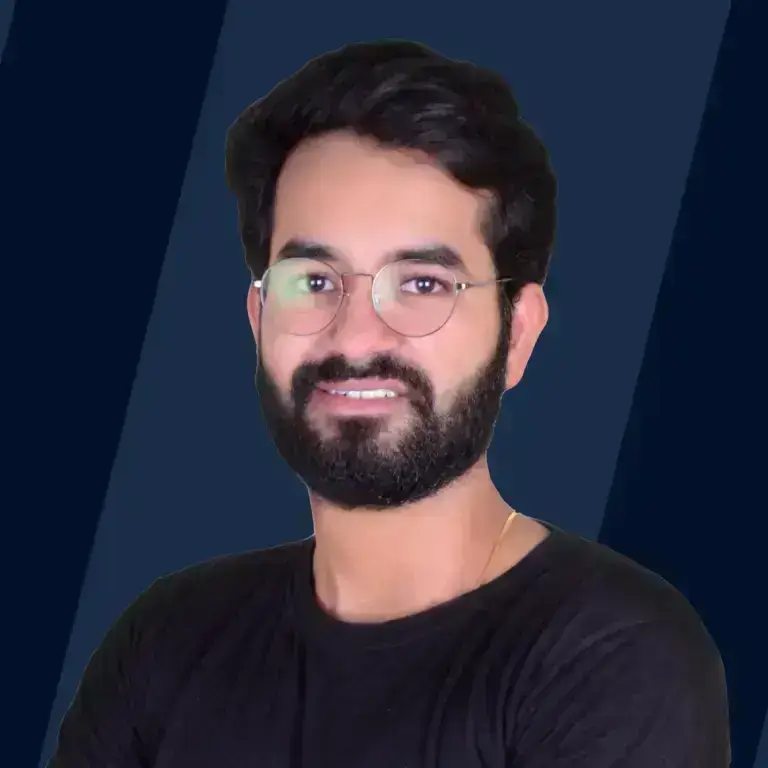
In Python, a normal dictionary is an unordered collection of data values that can be used to store data values in the same way that a map can. The Dictionary has a key-value pair and the key must be unique and immutable but it throws KeyError when we try to access non-existent key and breaks up the code. To overcome this problem, we use defaultdict in python, the only difference between defaultdict() and simple Dictionary is that when you try to access a non-existent key in defaultdict(), it does not throw an exception or KeyError. Hence, using defaultdict in python is a good option to handle errors.
Example
Lets take an example of a simple dictionary where we are accessing a key that is non existent, so it will throw KeyError.
Output:
KeyError is raised and the program is stopped.
In those cases defaultdict() is used to handle those cases:
Example:
Now, lets use defaultdict in python in the above case:
Output:
If we don't pass an object reference such as int or bool when initialising the defaultdict, we'll get a KeyError and won't be able to get the default fallback value in those cases. In this case, we've set the default value to int, which means that if a key is absent, it'll return 0. When we tried to get the value 4 in the dictionary, it just returned 0 instead of KeyError.
Syntax of Defaultdict
For importing and initializing defaultdict in python, we use the below syntax:
Parameters of Defaultdict
- default_factory: It is a function that returns default value for each data type in which the decitionary is defined. If nothing is passed as the argument then the dictionary raises a KeyError.
Further you can use inbuilt function for defaultdict in python like:
How Does Defaultdict Work in Python?
In addition to the conventional dictionary operations, defaultdict in python includes one writable instance variable and one method. The default_factory parameter is the instance variable, and the method supplied is __missing__.
Example 1: Using Default_factory Parameter
default_factory is a function that returns default value for each data type in which the decitionary is defined. If nothing is passed as the argument then the dictionary raises a KeyError.
Output:
As the default_factory is int, 0 is returned for non-existing value
Example 2: Using Missing()
__missing__() function is used to return the default value of the dictionary, so everytime we will be using the __missing__, we will get the default value of the dictionary. In this example, we are setting the default value using a lambda function.
Output:
In every case, we are getting "Default Value" as output.
More Examples
Giving Set as the Parameter:
When a set is passed as default_factory argument to the defaultdict, then each values of defaultdict will be a set.
Output:
It can be seen that all the values in the dictionary are set.
Giving List as the Parameter:
When a list is passed as default_factory argument to the defaultdict, then each values of defaultdict will be a list.
Output:
It can be seen that all the values in the dictionary are lists.
Conclusion
- In Python, a normal dictionary is an unordered collection of data values that can be used to store data values in the same way that a map can.
- The Dictionary has a key-value pair and the key must be unique and immutable but it throws KeyError when we try to access non-existent key and breaks up the code.
- The only difference between defaultdict in python and simple Dictionary is that when you try to access a non-existent key in defaultdict(), it does not throw an exception or KeyError.
- Defaultdict in python includes one writable instance variable and one method. The default_factory parameter is the instance variable, and the method supplied is __missing__.
- default_factory is a function that returns default value for each data type in which the decitionary is defined.
- __missing__() function is used to return the default value of the dictionary, so everytime we will be using the __missing__, we will get the default value of the dictionary.