How to Define Inheritance in Java?
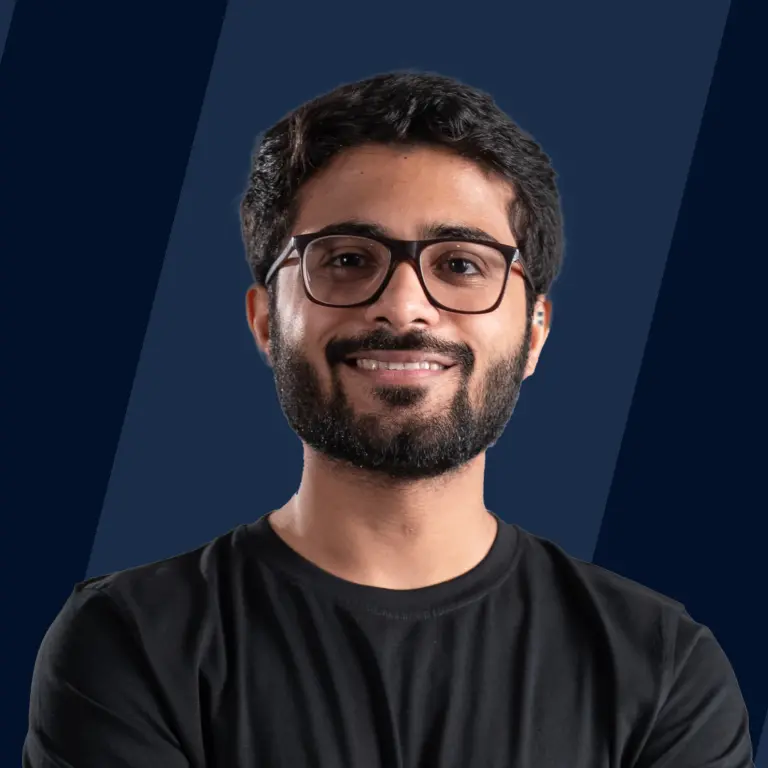
Inheritance in Java is one of the key features of Object-Oriented Programming. It is a concept by which objects of one class can acquire the behavior and properties of an existing parent class. In simple terms, we can create a new class from an existing class. The newly created class is called subclass (child class or derived class) that can have the same methods and attributes as the existing class (superclass or parent class or base class).
We can also add additional new methods and features inside our subclass. Inheritance in Java demonstrates a parent-child relationship between the participating classes and it is also known as Is-A relationship.
Extends keyword
We use the " extends" keyword to demonstrate Inheritance in Java by creating a derived class from the parent class. The extended keyword is used to indicate that a specific class is being inherited by another class.
For example: class A extends B which means class A is being derived from the parent class B.
Some important terms used to define inheritance in Java
- Class: A class is a blueprint or a template for creating objects.
- Subclass: It is also known as the child class or the derived class that inherits the properties of the parent class.
- Parent class: It is also known as the superclass or the base class from which other subclasses inherit the properties.
- Reusability: It is a concept by which we can reuse the methods and the fields of an existing class in our newly derived class without actually writing the code again and again.
Syntax of inheritance in Java
As we can see in the below image, class A is the parent class of class B and class C is further derived from class B using the "extends" keyword. This is demonstrating multi-level inheritance which we will study in the later course of this article.
Why do we need inheritance in Java?
Now the question arises that why we need Inheritance in Java and the simple answer for code reusability purposes.
The code and the methods written for the parent class can directly be used by the child class and also, and we can achieve run-time polymorphism (method overriding).
Example (inheritance in Java)
In this Java example code, we have two classes: A (parent class) and B (derived class, inherited from class A). To demonstrate the concept of Inheritance in Java, we have firstly created an object obj of derived class B. We can now access the fields and methods of the parent class with the help of the object of the derived class.
Code:
Output:
Explanation:
- We have set the value of the name attribute and invoked the display() function to print the name.
- We have also invoked the getName() function by passing "learning" as a parameter to display the resultant value.
Some important facts about inheritance in Java
- A subclass can have only one superclass but a superclass can have any number of subclasses.
- A subclass can inherit all the members (methods and fields) of the parent class except the private members.
- The constructors are not considered members of a class and are not inherited by subclasses.
- While constructors are not inherited by subclasses, subclasses can call their parent class's constructors using the super keyword, and a default constructor is automatically created by the Java compiler only if no explicit constructors are defined in the class.
Example 1: Method Overriding In Java Inheritance
Have you ever wondered what will happen if a specific method is present both in the subclass and the superclass? In such cases, methods of the subclass override the methods of the parent class and hence, this is known as method overriding in Java.
For method overriding, methods must have the same name & the same number of parameters in the parent and the child class. This concept is important to achieve run-time polymorphism.
Let's take an example to understand this.
Code:
Output:
Explanation:
- In this Java example code, we have two classes: a child class (class Two) and a parent class (class One), and both have the same functional method with the same parameters.
- In the case of function calling with the help of the object of the subclass, the method of the subclass will override the method of the parent class, and hence, the output of the subclass's method will be displayed.
Example 2: Super Keyword In Java Inheritance
The super keyword in Java Inheritance is used to invoke the methods, variables, and the constructors of the parent class.
Let's see an example to understand this.
Output:
Explanation:
- In this Java example code, we have a child class B and a parent class A, having the same functional method inside them.
- This is the case of method overriding. When we try to invoke the functional method solve(), it invokes the method inside the subclass.
- The super keyword has been used inside the subclass to access the members and the methods of the superclass.
- Here, super.solve() will invoke solve() method inside class A and super.i will take in the value of i from class A i.e. the parent class of B.
Example 3: Protected Members In Java Inheritance
There is a point to be noted that the protected members (fields and functional methods) of the superclass are also accessible from its subclasses.
Output:
Explanation:
- In this Java example code, we have protected members in the superclass A which are directly accessible from the subclass B.
- As we can see, we are trying to access the name attribute and the solve() function of the superclass with the help of the object obj of subclass B, and accordingly, the result has been displayed.
Types Of Inheritance In Java
There are five types of Java Inheritances and now, we will study them with their implementation code in Java :
- Single Inheritance
- Multilevel Inheritance
- Hierarchical Inheritance
- Multiple Inheritance
- Hybrid Inheritance
1. Single Inheritance
In single inheritance, a single subclass is extended from a single superclass and inherits its properties.
Output:
Explanation:
- In the below Java example code, we have two classes with an Is-a relationship: class One (parent class) and class Two (subclass).
- Using object obj of subclass Two, we are accessing the method of the superclass One and displaying the result.
2. Multilevel Inheritance
In multilevel inheritance, a child class is derived from a parent class and that child class further acts as a parent class and is used to derive another child class. This is a single increasing chain of inheritance.
Code:
Output:
Explanation:
- Here, we are implementing multilevel inheritance. We have three classes: class One, class Two derived from class One, and class Three derived from class Two.
- In this, class One becomes the parent class for class Two and class Two further becomes superclass for class Three.
- Using the object obj of class Three, we can access all the members of class One and class Two, and hence, the result has been displayed.
3. Hierarchical Inheritance
In Hierarchical Inheritance, one superclass is used to derive more than one subclass or we can say that multiple subclasses extend from a single superclass.
For example:
Output:
Explanation:
- In the below Java example code, one parent class A is used to derive two subclasses B and C using the " extends" keyword and both the subclasses inherit the properties of the parent class A.
- For clarity, we have made two separate class objects for class B and class C, and then we are accessing the method of class A to print the resultant value.
4. Multiple Inheritance (Not Supported by Java)
In Multiple Inheritance, one subclass is derived from more than one superclass. Java doesn't support multiple inheritances because of high complexity and logic issues but as an alternative, we can implement multiple inheritances in Java using interfaces.
For example:
Output:
Explanation:
- Here, we have created three interfaces where interface Three extends interfaces One and Two.
- Also, the class child then implements interface Three to inherit properties of all the interfaces.
5. Hybrid Inheritance
Hybrid Inheritance in Java is a combination of two or more types of inheritance. In simple terms, more than one type of inheritance is being observed in the case of Hybrid inheritance. As we know that Java doesn't support multiple inheritance with classes, similarly we can't implement hybrid inheritance that consists of multiple inheritance in Java.
In the case of demonstrating multiple inheritance in Java, we must have to use interfaces for implementation purposes as we have seen in the example of multiple inheritances.
For example:
Output:
Explanation:
- In this Java example code, we have four classes: A, B, C, and D where classes A and B are inherited from class C, and class D is further extended from the class A.
- Class A and B extend class C which showcases Hierarchical inheritance while Class D extends class A and this showcases Single inheritance in Java.
Hence, this form of inheritance in this example is known as Hybrid Inheritance in Java.
Explore Scaler Topics Java Tutorial and enhance your Java skills with Reading Tracks and Challenges.
Conclusion
- Inheritance in Java is a concept by which the objects of one class can acquire the behavior and properties of other objects.
- We use the “extends” keyword to demonstrate Inheritance in Java by creating a derived class from the base class.
- We need inheritance in Java to implement method overriding (run-time polymorphism) and for code reusability purposes.
- A subclass can have only one superclass but a superclass can have any number of subclasses.
- Super keyword in Java Inheritance is used to invoke the methods, variables, and constructors of the parent class.
- There are five types of Inheritance in Java: Single Inheritance, Multilevel Inheritance, Hierarchical Inheritance, Multiple Inheritance, and Hybrid Inheritance.