Delete Element From List Python
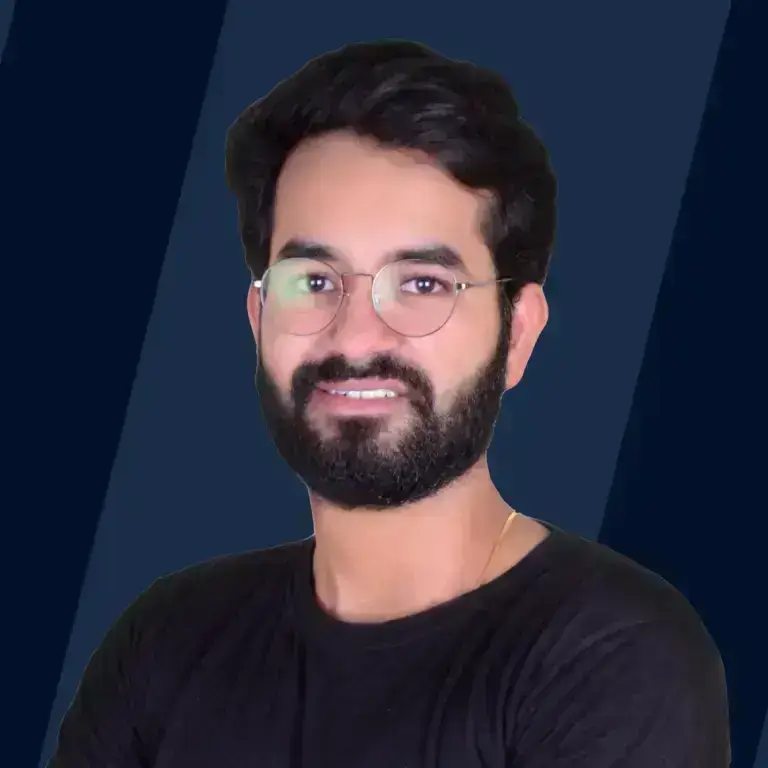
This article explores various methods to delete or remove elements from a Python list. We will discuss syntax, showcase examples, and highlight common coding errors. The focus is on a Python list representing student marks in Computer Science, where absentees are marked with zero. The objective is to remove these zeroes. Additionally, we'll address situations where a list contains incorrect data and needs to be cleared entirely. The article will guide you through multiple techniques, including the 'clear()' method, to efficiently manage list elements in Python.
Method 1: Remove All Elements Using Clear()
Suppose you have a list
Now, if we call .clear() method on a list (object) then, all the elements in the Python list get deleted.
Syntax:
Please note that
- .clear() method returns None value, therefore, running the following code will result in res being None:
- calling .clear() method on an empty list returns an empty list only
Example:
Code:
Output:
Explanation: After calling the .clear() method on the list l, the size of the list becomes 0 and it becomes empty. All the elemets have been deleted from the Python list.
Method 2: Using pop()
Given a list l, calling pop() method
- deletes and returns the last element in the Python list if no index is specified
- deletes and returns the element at the specified index in the Python list
Syntax
There are 2 possible syntaxes for using the pop() method to remove an element from a list in Python:
Example 1
Code:
Output:
Explanation: Since no index was specified, the last element was removed from the Python list and returned.
Example 2:
Code:
Output:
Explanation: Since in this case, we specified an index, the element at index 4 in the list (that is, 5) gets removed and returned.
Please Note
- The list on which the .pop() method is being called should not be empty
- If the index is specified, it should not be out of range for the list
In both of these cases, errors will be thrown by the program. See the following examples:
Error Code 1:
Error Output 1:
Error Code 2:
Error Output 2:
Error Code 3:
Error Output 3:
Method 3: Using remove() Method
We can remove an element from a list in Python using the remove() method on a list data structure. remove() takes a target value (that is to be removed) and deletes it from the Python list.
If there are multiple occurrences of the target value, then only the first occurrence of that element is removed from the Python list.
Syntax
Example 1
Code:
Output:
Explanation: Since the target value 32 is present in the list l, it is removed when we call the remove(32) method on it.
Example 2
Code:
Output:
Explanation: Since the string aman was first present at index 0, it is removed and the aman present at index 3 is not. Remember, the remove() method only removes the first occurrence of the target.
Example 3
Code:
Output:
Explanation: The program will automatically match the data type to be removed, so there is no strict type that the method should be adhered to.
Please Note
- The target value should be present in the list before calling the remove() method.
- Calling the method will raise a TypeError. Unlike the pop() function we described above, the argument is required here.
See the following examples to understand the errors:
Error Example 1
Code:
Error Output:
Explanation: As mentioned above, the target value must be present in the Python list before the remove() method is called on it. Since 100 was not present in l, the program raises a ValueError.
Error Example 2
Code:
Error Output
Explanation: Since we provided 0 arguments where 1 argument was expected, we get a TypeError.
Method 4: Using del() Statement
del is a very powerful statement in Python that can be used to delete elements from Python lists in a variety of ways.
It can be used to:
- delete an element by providing its index
- delete a range of elements (that is, a slice) in a list
- delete all the elements in the list
Considering the first way, it is very similar to the pop() method we saw above. The difference being
- pop() is a method, del is a statement
- del statement does not return the deleted elements, whereas the pop() method does.
Syntax
Example 1:
Code:
Output:
Explanation: Using the del statement, we removed the element present at index 1 in the Python list, that is 2.
Example 2;
Code:
Output:
Explanation: In the first operation, we specified the slice 1:3, where the elements [2,3] were removed from the Python list.
In the second operation, since the entire length of the list was given as slice, the entire list was cleared out.
Please Note:
Using del with the name of the list will remove the list's reference from the memory itself, hence you won't be able to access it at all.
Code:
Output:
Explanation: Before using del, l was present in the memory, but after using that statement, it was no longer present in memory, so can't be printed.
Method 5: Removing Items from a Python List that Meet a Certain Conditions
For removing items (from a list in Python) that meet a certain condition, we use list comprehensions. List comprehensions let you create a new list with a given predicate.
Syntax
Using list comprehensions is more like a sub-set selection rather than deletion, strictly speaking. But we convert it to deletion operation by assigning the variable name to the newly assigned list.
Example 1:
Code:
Output:
Explanation: Using list comprehensions, we first choose the elements that fulfil our criteria, that is, they are multiples of 3 and put them in a list. After that, the variable l is assigned to the newly created list and removed from the old one.
Example 2:
Code:
Output:
Explanation: Iterating over the elements of l with index i, we choose elements l[i] such that it is even and put them into a new list. After that, the list is assigned the variable name l.
Conclusion
- List is a data structure that contains homogenous as well as heterogeneous collections of data and is iterable and indexable.
- To delete all the elements in a Python list myList we can use any one of the following:
- To delete just the last element in Python list we can use:
- To delete the first (or only) occurrence of a given element in a list in Python
- To delete the element at a particular index i in a list in Python
- To delete a slice of a list in Python:
- Care should be taken for IndexError, ValueError, and TypeError while calling many of these methods.