Deque in Python
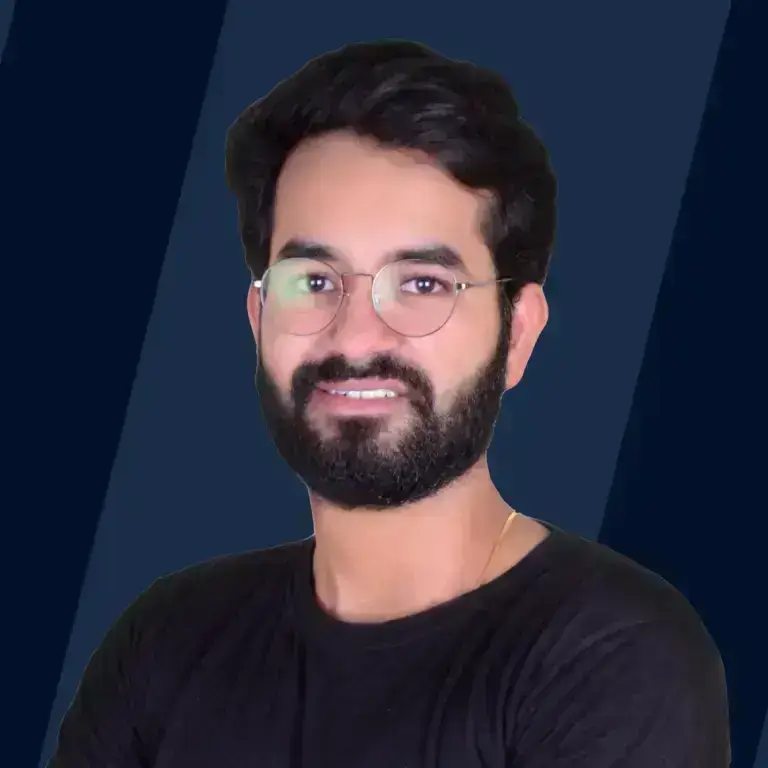
In the realm of Python programming, if you find yourself pondering, "What is deque?" look no further. Known as a superhero-like tool, deque stands for double-ended queue. This powerful feature enables you to add or remove elements from both ends of a queue with lightning speed, making it a staple for programmers who value efficiency and flexibility.
Types of Restricted Deque Input
Restricted deques confine operations to one end or limit the number of operations to enhance performance or enforce data management rules. The two common types of restricted deque in python are:
-
Input-Restricted Deque: In this type, insertion is limited to one end, although deletion can occur at both ends. This setup is particularly useful when you want to ensure that new data enters the queue in a controlled manner, maintaining an orderly processing sequence.
-
Output-Restricted Deque: Conversely, an output-restricted deque allows insertion at both ends but restricts deletion to just one end. This configuration can be advantageous in situations where you need to process elements in a specific order but require flexibility in how new elements are added to the sequence.
Each type of restricted deque serves unique requirements, enabling developers to tailor data management strategies effectively within their Python applications.
Python code to demonstrate deque
Output:
Explanation
As you can see in the above example that has months in it. When we just append() "May" to it then it will be added in the deque at the rightmost side but when we want to append "Jan" on the leftmost side of the dequeue then the appendleft() is used. Similarly when we want to pop the "May" from the deque then just the pop() method is used but when "Jan" has to be removed then the popleft() method will be used.
Operations on deque
Popping and Appending Items Efficiently
Deque in python is designed for fast appending and popping of items from either end. Use append() to add items to the right end and pop() to remove them from the right. To work with the left end, use appendleft() and popleft().
Example:
Output:
Accessing Random Items in a Deque
While deque in python are optimized for operations on the ends, you can access elements at any position directly with indexing, albeit less efficiently than list due to its underlying data structure.
Example:
Output:
Size of a Deque
To determine the number of items in a deque, use the len() function.
Example:
Output:
Front and Back of a Deque in Python
Access the first item with dq[0] and the last item with dq[-1] in a deque.
Example:
Output:
Building Efficient Queues With Deque
Deque in python are ideal for implementing queues where you need quick appends and pops from either end.
Example:
Output:
Limiting the Maximum Number of Items: maxlen
The maxlen parameter limits the number of items in a deque, automatically discarding items from the opposite end as needed.
Example:
Output:
Rotating the Items: .rotate()
Rotating the deque in python moves elements around. A positive value rotates to the right, and a negative value to the left.
Example:
Output:
Adding Several Items at Once: .extendleft()
The .extendleft() method adds multiple items to the left end of the deque. Note that the iterable is added in reverse order.
Example:
Output:
Reverse the Order of Deque Elements: .rotate()
Interestingly, you can reverse the order of elements in a deque by using .rotate() cleverly, or more directly with .reverse().
Example with .reverse():
Output:
These operations showcase the flexibility and efficiency of deques in Python, making them a powerful tool for a wide range of applications.
Complexity Analysis
Understanding the efficiency of different operations in Python's deque is crucial for writing optimized code. Here is a concise breakdown of how each operation stacks up in terms of time complexity and the extra space required:
Actions on Deque | Complexity of Time | Space Needed |
---|---|---|
append() | Constant (O(1)) | Constant (O(1)) |
appendleft() | Constant (O(1)) | Constant (O(1)) |
pop() | Constant (O(1)) | Constant (O(1)) |
popleft() | Constant (O(1)) | Constant (O(1)) |
index(value) | Linear (O(n)) | Constant (O(1)) |
insert(position, value) | Linear (O(n)) | Constant (O(1)) |
remove(value) | Linear (O(n)) | Constant (O(1)) |
count(value) | Linear (O(n)) | Constant (O(1)) |
length | Constant (O(1)) | Constant (O(1)) |
access (dq[0]) | Constant (O(1)) | Constant (O(1)) |
access (dq[-1]) | Constant (O(1)) | Constant (O(1)) |
extend(iterable) | Linear (O(k)) | Constant (O(1)) |
extendleft(iterable) | Linear (O(k)) | Constant (O(1)) |
reverse() | Linear (O(n)) | Constant (O(1)) |
rotate(steps) | Linear (O(k)) | Constant (O(1)) |
Note: In the table, 'n' represents the number of elements currently in the deque, while 'k' denotes the number of elements being added or rotated.
Use cases of Deque in Python
Deque in python are a double-ended queue data structure that offers fast appends and pops from both sides and can be used in a variety of practical scenarios. Here are three common use cases for deques in Python, along with code snippets:
Keeping a Page History
In web browsers, deques can be used to stores track of the pages a user has visited, allowing for a quick back and forth navigation.
Example:
Output:
Emulating the Tail Command
The tail command in Unix systems is used to display the last few lines of a file. Deque in python can emulate this functionality efficiently by keeping track of a limited number of lines.
Example:
Note: Replace 'example.log' with the path to the actual file and uncomment the last two lines to see the output.
Multithreaded Applications
In producer-consumer scenarios, deques can be used as a thread-safe queue for inter-thread communication without needing additional locking mechanisms.
Example:
Note: This example will run indefinitely. In a real-world scenario, you would implement a stopping condition.
Conclusion
- Deque in Python is engineered for high-speed operations at both ends, offering methods like append() and pop() that work in constant time.
- Whether it’s managing browser history or implementing a queue system, the deque's flexibility makes it suitable for a broad spectrum of applications.
- Deque in python provides the maxlen option, enabling automatic size restrictions and thus preventing unchecked growth, which is especially useful in resource-constrained environments.
- With thread-safe operations, deques are an excellent choice for multithreaded applications, ensuring data integrity without the overhead of locking.