Python Design Patterns
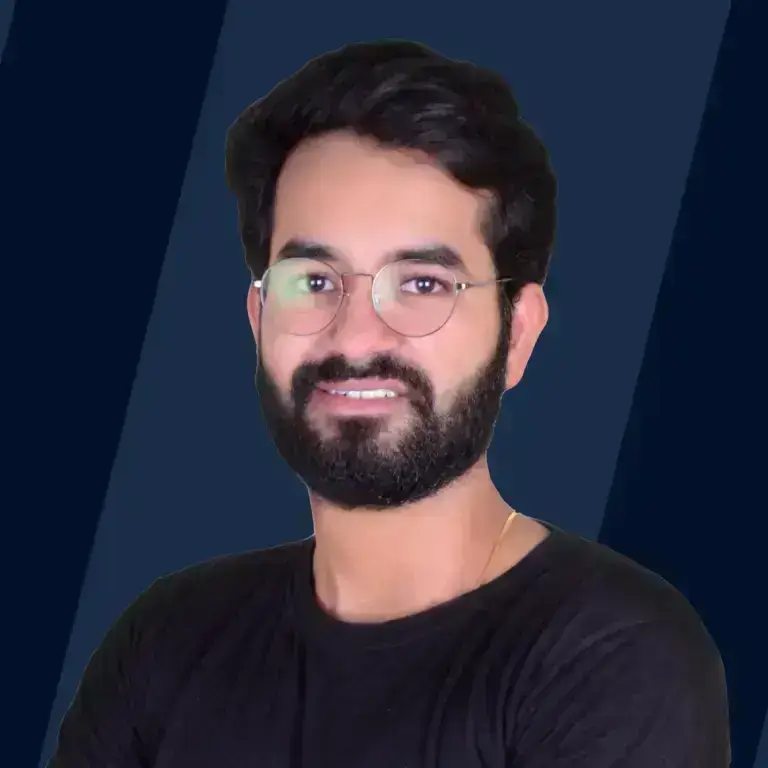
Design patterns in Python are crucial blueprints for creating efficient and maintainable code. These established solutions solve common software design difficulties while improving flexibility and scalability. Python developers use structures such as the original Singleton and the flexible Strategy pattern to simplify their codebases. By embracing design patterns, you are not only creating code; you are also designing sturdy solutions that will withstand the test of time in the ever-changing context of software development. Explore the world of Python design patterns and improve your coding skills.
Creational Design Pattern
Creational Design Patterns in Python programming, serve as architectural blueprints for creating things. These patterns simplify the object generation process, providing greater flexibility and speed in code development. Let us begin on a trip to understand the essence of Python's Creational Design Patterns.
Singleton Pattern
The Singleton pattern limits a class to a single instance and provides a global access point. In Python, this pattern is easily accomplished by storing the instance in a class property and calling a function to obtain or construct it as needed.
Factory Method Pattern
When using the Factory Method pattern, subclasses can change the kind of objects that are generated. Python demonstrates this pattern well, allowing developers to specify an interface for constructing an object and allowing subclasses to change the type through inheritance.
Abstract Factory Pattern
The Abstract Factory design allows you to create families of connected or dependent items without specifying their actual classes, broadening your horizons. Python's dynamic typing and flexibility show through as it allows for the seamless development of a wide range of object families.
Builder Pattern
The Builder pattern distinguishes between building a complicated thing and representing it. Python's expressive syntax makes it easier to implement this pattern, allowing for the step-by-step building of things with varying complexities.
In the Python coding world, learning Creational Design Patterns not only improves code modularity but also allows developers to handle the complexities of object creation with ease. With these patterns at your disposal, you have the keys to building powerful and versatile Python programs. So embrace the beauty of Creational Design Patterns and improve your Python programming skills.
To learn more about the design patterns and creational design patterns, clickhere.
Structural Design Patterns
Structural design patterns in Python serve an important role in improving code organisation and adaptability, ensuring a solid basis for future growth. These patterns simplify the composition of classes and objects to improve code reuse and maintainability.
The Adapter pattern is a well-known structural design pattern that acts as a bridge between incompatible interfaces, making cooperation easier. This enables systems to interact effortlessly, even if they were not originally meant to do so.
Another useful pattern is the Decorator pattern, which provides a flexible approach to dynamically extend or add responsibilities to objects. It encourages clean and scalable codebases by enabling behaviour to be introduced progressively.
The Composite pattern is extremely useful for consistently addressing individual items as well as object combinations. This simplifies client code, rendering it insensitive to whether it is dealing with a single object or a complicated hierarchy.
The exterior pattern simplifies the interfaces of a subsystem, making it easier to use. It protects customers from the complexity of the subsystem, minimising dependency and enhancing overall system understanding.
These structural design principles help Python developers construct code that is well-organized, adaptable, and scalable. By using these patterns, programmers may improve code readability, decrease repetition, and promote a more efficient and maintainable software development process.
To read more about the design patterns and structural design patterns, click here
Behavioral Design Pattern
Behavioural Design Patterns are important in Python programming because they define how objects interact and communicate. These patterns emphasise the transfer of responsibility amongst objects, as well as their collaboration and coordination.
The Observer Pattern is a common behavioural design pattern in which an object, known as the subject, keeps a list of dependents, or observers, who are alerted of any state changes. This approach is especially effective in situations when numerous items must react to changes in another object.
Another notable pattern is the Strategy Pattern, which enables developers to construct a family of algorithms, encapsulate them, and make them interchangeable. This increases flexibility and allows for dynamic algorithm selection at runtime. Python's dynamic nature and support for first-class functions make it an ideal choice for implementing the Strategy Pattern effortlessly.
Command Pattern is yet another valuable tool in the behavioural patterns arsenal. It encodes a request as an object, allowing for client customisation with various requests, request queuing, and parameter reporting. Python's command pattern implementation improves code flexibility and extensibility, resulting in an elegant solution for a variety of use scenarios.
Finally, learning Behavioural Design Patterns in Python enables developers to write more modular, adaptable, and maintainable programs. By knowing and applying these patterns, you can elevate your Python programming skills and design robust software that stands the test of time.
To learn more about design patterns and behavioural pattern, click here.
Importance of Learning Design Pattern
In Python programming, learning design patterns is an addition to your coding cap. Design patterns, or reusable solutions to common issues, enable developers to build durable and scalable software systems. Understanding their significance in Python is like discovering a treasure trove of efficiency and maintainability.
Python developers who study design patterns obtain a handy arsenal for dealing with reoccurring issues. The Singleton pattern, for example, assures that a class has only one instance, but the Observer pattern promotes efficient communication between objects. These patterns not only improve code readability but also encourage adaptability to changing requirements.
Furthermore, design patterns encourage best practices and a common vocabulary among developers. Whether you use the Factory Method to generate objects or the Strategy pattern to pick algorithms, you are tapping into common expertise that extends beyond individual projects.
Python's flexibility as a language makes it an attractive platform for implementing design patterns. Whether you work in web development, data science, or artificial intelligence, design pattern concepts are universally applicable. They offer an organised approach to issue resolution, which reduces defects and code duplication.
To summarise, investing effort in mastering design patterns is more than simply an academic exercise; it is a smart step to improve your Python skills. When you include these designs into your coding toolbox, you're not simply writing code you are creating a project that is efficient enough to solve real-world problems.
Conclusion
- Design patterns serve as appealing blueprints, offering developers tried-and-true answers to common design difficulties. Encapsulating best practices improves code's maintainability and adaptability.
- Python's design patterns encourage the reuse of effective design solutions, which reduces duplication and prevents developers from reinventing the wheel. This not only speeds up development but also promotes a modular and extensible codebase.
- The strength of abstraction in design patterns enables developers to build flexible and extendable systems. Python design patterns encourage adaptation to changing needs by isolating high-level design decisions from low-level implementation details.
- Design patterns provide engineers with an agreed-upon language to communicate and share best practices. This standardisation improves communication among team members, resulting in a more coherent and efficient development process.
- Design patterns in Python to make your program scalable and maintainable. Patterns like Singleton and Observer help to create scalable structures, whereas Decorator makes it easier to introduce new features without altering old code.
- Design patterns protect against future uncertainty. Python developers may future-proof their code by adding these tried-and-true strategies, making it more adaptive and resilient as the software ecosystem changes.