Destructor in C++
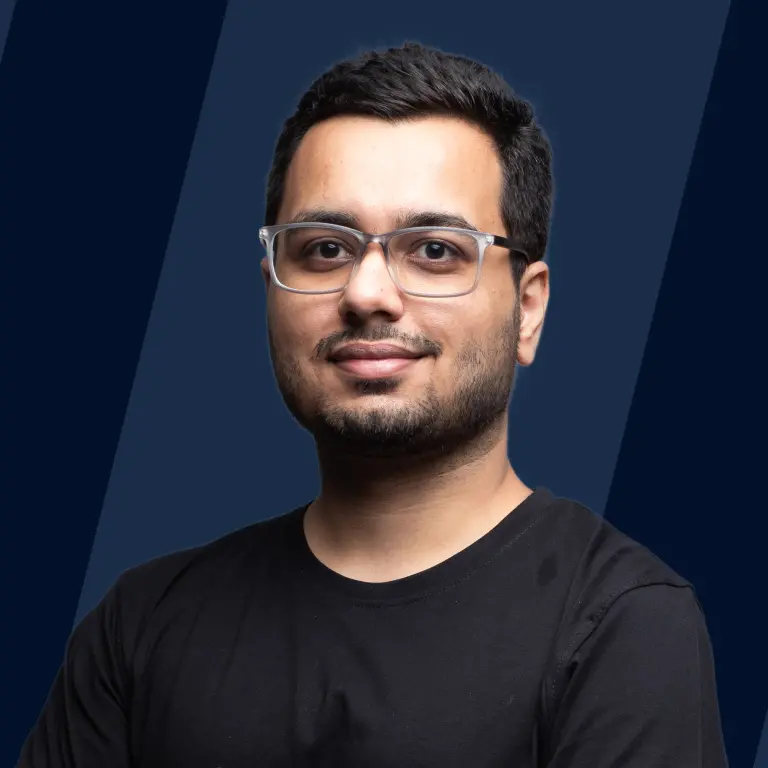
Introduction
Destructors are essential in C++ for managing resources and cleaning up after things. They are called when the scope of an object expires or when 'delete' is explicitly invoked. Understanding the order of destruction is critical because it ensures optimal resource allocation. Developers may improve the speed and stability of their C++ programs by designing destructors, which provide a graceful exit for objects.
Syntax
Destructors are essential in C++ programming because they ensure efficient resource management and cleaning. They are constructors' equivalents, in charge of allocating resources obtained over an object's lifecycle. Understanding their logic is critical for building memory-efficient C++ programs.
A destructor in C++ has the same name as the class prefixed by a tilde (~). Here's an example of basic syntax.
When an object exits the scope or is expressly removed, the destructor is automatically called. Its principal role is to free resources held by the object, such as memory, files, or network connections.
Destructors are particularly important for avoiding memory leaks and ensuring appropriate resource deallocation. They offer a dependable method for performing cleaning actions, making them an essential component of C++ programming best practices.
Developers can improve the efficiency and stability of their programs by using the right syntax for destructors. Remember to use caution while implementing them, taking into account the unique cleaning needs of your objects. This guarantees that your C++ programs not only run efficiently but also have a high level of resource management.
Properties of Destructor
Destructors are essential in resource management and ensuring that things are left cleanly. Understanding destructor attributes is essential for competent C++ programming.
Automatic Invocation:
Destructors are special member functions that are automatically invoked when an object goes out of scope or is explicitly deleted. This automated invocation guarantees that resources are released on time, avoiding memory leaks and resource mismanagement.
Example:
Reverse Order of Constructors:
The order in which destructors are called is the reverse of the order in which constructors are called. This helps to preserve consistency and guarantees that object dependencies are handled effectively after destruction.
Example:
No Return Type or Parameters:
Destructors, unlike constructors (builders of an object), do not have a return type or arguments. They are distinguished by the tilde (~) sign, which is followed by the class name. This simplicity in syntax reflects the straightforward purpose of destructors – "cleaning up resources".
Example:
Implicit and Explicit Definition:
If a class lacks an explicitly specified destructor, C++ generates an implicit destructor. An explicit destructor is advised for classes that manage dynamic memory or other resources, however, to ensure proper cleanup.
Example:
Inheritance and Virtual Destructors:
In inheritance scenarios, it is necessary to specify the base class destructor as virtual. This enables the proper destruction of derived class objects via a base class reference, preventing memory leaks and ensuring the correct sequence of destructor calls.
Example:
Finally, learning destructor properties is essential for writing robust and efficient C++ programs. The appropriate use of destructors ensures good resource management, which helps the reliability and stability of your C++ applications.
Examples
Let us take some examples to understand more about the Destructors in C++.
Default Destructor
When the default destructor is not expressly stated by the programmer, the compiler produces it. It is called without arguments when an object is withdrawn from scope or manually deleted. This destructor runs in the background, freeing dynamically allocated memory and cleaning up resources.
Copy Constructor and Destructor
When a copy constructor is used to create a deep copy of an object, a corresponding destructor is necessary to avoid memory leaks. This dynamic pair ensures that the copied object's resources are appropriately relinquished when it is no longer needed.
Virtual Destructor for Polymorphism
When working with polymorphic classes and inheritance, a virtual destructor is essential. When deleting a derived class using a base class reference, the correct destructor is run.
Array Destructor for Dynamic Arrays
When arrays are allocated dynamically using the new[] keyword, each array member requires its array destructor to deallocate memory.
Understanding and implementing these destructor types allows C++ programmers to develop durable, memory-efficient programs that leave no trace of resource misuse. So, the next time you write C++, remember that a well-defined destructor is your program to up after your objects and keep your code tidy.
FAQs
Let us look at some of the most frequently asked questions related to the destructors in C++:
Q: In C++, when do we use the phrase "destructor"?
A: When an object departs scope or is expressly wiped with the delete keyword in C++, a destructor is called.
Q: How Do I Explicitly Call a Destructor in C++?
A: In most circumstances, you do not need to call a destructor manually. It is automatically called when the scope of an object ends or when delete is invoked. However, specifically naming it is not common behavior.
Q: In C++, what separates Destructors from Regular Functions?
A: Destructors are named after the previous class, but with a tilde(~). Destructors, unlike regular functions, do not have a return type and cannot be explicitly invoked.
Q: Can a class in C++ have more than one destructor?
A: No, each class is only allowed to have one destructor. Multiple destructors are impossible because the destructor is identified by the class name followed by a tilde.
Q: When should you utilize user-defined destructors in C++?
A: User-defined destructors become useful when a class incorporates dynamic memory allocation or specific cleaning mechanisms. When an object is destroyed, developers can implement custom actions.
Q: Can a Destructor be Virtual in C++?
A: Yes, a destructor can be virtual. When destroying a derived class object via a reference to the base class, virtual destructors guarantee that the right destructor is invoked.
To understand more about the virtual destructors in C++, please click here.
Conclusion
- In C++, destructors play an important role in guaranteeing smooth resource management. They act as a cleanup manager, freeing up allocated memory and closing open files to prevent memory leaks and resource hogging.
- C++ coders have the benefit of an automated cleanup method using destructors. When objects created within a scope leave that scope, their destructors are executed, relieving programmers of the effort of manual resource deallocation.
- Destructors aid in the orderly termination of things by executing in the reverse order in which they were created. This property guarantees that object dependencies and relationships are smoothly dissolved, facilitating a smooth shutdown procedure.
- Destructors play an important role in exception safety in C++. By performing resource cleaning within the destructor, developers may rely on the destructor's automatic invocation even when exceptions are present, ensuring program stability.
- C++ allows programmers to specify bespoke destructors, allowing for customized resource management methods. This adaptability is especially useful when working with complicated items that need specialized cleansing techniques.
- Destructor proficiency improves developers' grasp of object lifecycles. This understanding is essential for designing strong and efficient C++ code since it fosters a greater respect for the complexities of memory management.