Program to Print Diamond Pattern in Java
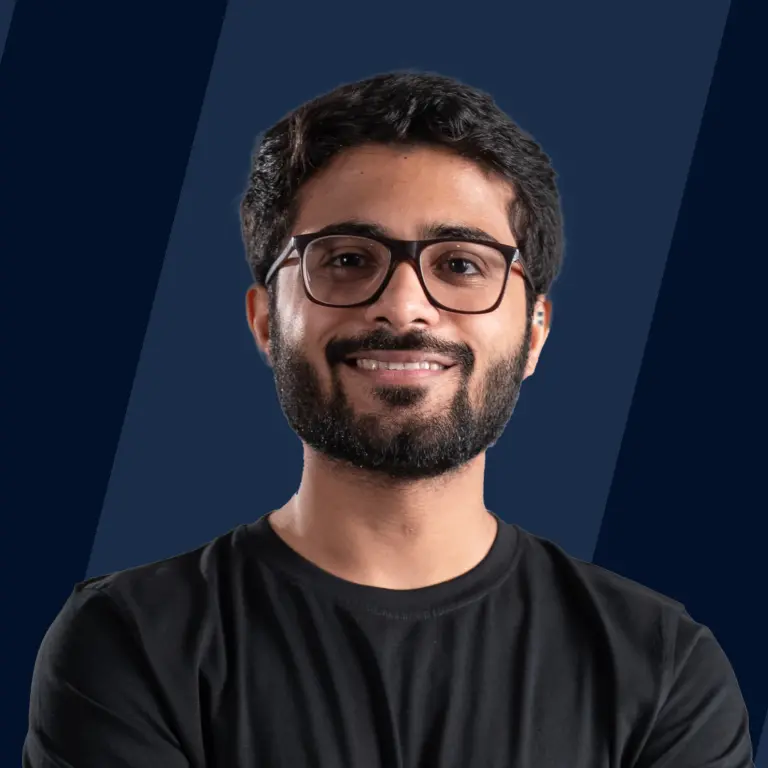
Overview
Creating Java pattern programs is an effective way to improve coding skills, logic understanding, and grasp of looping concepts. These programs are frequently used in Java interviews to assess problem-solving and reasoning abilities. When solving such programs, visualizing the pattern is a helpful starting point. They usually involve nested loops, with the first loop managing rows and subsequent loops handling columns, making extensive use of 'for' loops in Java
Pattern programs fall into three main categories, these are as follows:
- Star Patterns
- Number Patterns
- Character Patterns.
In this article we'll learn how to create a diamond pattern in Java.
Illustration
As you can see from the diamond pattern in Java, each line here consists of two parts: spaces and asterisks.
The number of spaces on each line decreases as you move up, while the number of asterisks increases. Right after that comes the the second half of the pattern, where the number of spaces increases and the number of asterisks decreases in a symmetrical manner thus creating an illusion of a diamond shape in the center, with a tapering effect as we move away from the center.
Algorithm
- Take the number of rows (n) as input from the user.
- Calculate the middle row of the diamond pattern, which is .
- Use nested loops to iterate through each row and column.
- In the outer loop (for rows):
- Initialize a loop variable i from to .
- In the inner loop (for columns):
- Calculate the absolute difference between the current row number (i) and the middle row.
- If this difference is less than or equal to , print a star or space accordingly to create the diamond shape.
- Otherwise, print a space.
- Move to the next row and repeat the process until the diamond pattern is complete.
- End the program.
Why do we use the middle row for symmetry in diamond pattern in Java?
Let's look at the following reasons:
- Increased Symmetry:
Symmetry around the middle row creates a visually appealing diamond shape. - Logic:
Using the middle row as the axis of symmetry simplifies the logic for printing the diamond. It reduces the number of iterations and makes it easier to control the pattern's symmetry. The middle row represents the point where the pattern transitions from increasing to decreasing in size. - Balance:
The middle row ensures that the upper and lower halves of the diamond pattern have a balanced and equal number of rows. This balance ensures that the pattern looks symmetrical. - Modification:
If someone decides to change the size of the diamond pattern, the middle row used as the reference point makes it straightforward to adjust the pattern by modifying the number of rows above and below the middle row.
Print Diamond Pattern in Java
Using While Loop
A while loop is condition-based. It continues executing as long as the specified condition is true.
Output:
Using Do While Loop
A do-while loop guarantees that its body is executed at least once because the condition is evaluated after the loop body.
Code:
Output:
Using For Loop
A for loop consists of three parts in its declaration: initialization, condition, and iteration.
Code:
Output:
FAQs
Q: How can I change the character used to create the diamond pattern in Java?
A: You can change the character used to create the diamond pattern by replacing the asterisk (*) in the code with any other character of your choice, such as '#' or '@'. Simply replace the '' character with your desired character in the code snippet provided earlier.
Q: How can I customize the size of the diamond pattern in java?
A: You can change the size of the diamond pattern by modifying the value of n in the code. A larger n will create a bigger diamond, while a smaller n will create a smaller one.
Q: How can I center the diamond pattern in java, on the console screen?
A: To center the diamond pattern on the console screen, adjust the number of spaces printed before the asterisks in the code. This ensures that the pattern is horizontally centered.
Q: How is the tapering effect achieved in the pattern?
A: The tapering effect is where the number of spaces decreases and asterisks increases until we reach it's center. This can be achieved by adjusting the inner loop parameters based on the current row since as the row number increases, the number of spaces needs to decrease while the number of asterisks should increase.
Conclusion
- Printing a diamond pattern in java involves using nested loops. The outer loop controls the rows, and the inner loop manages spaces and stars within each row
- The logic for printing a diamond pattern in java revolves around calculating the number of spaces and stars for each row based on the row number and the total number of rows
- Handle edge cases, such as when the number of rows is or an even number, differently to ensure the pattern remains symmetrical
- Using the middle row as the axis of symmetry simplifies the logic for printing the diamond. It reduces the number of iterations and makes it easier to control the pattern's symmetry. The middle row represents the point where the pattern transitions from increasing to decreasing in size.
- A do-while loop guarantees that its body is executed at least once because the condition is evaluated after the loop body.
- A for loop consists of three parts in its declaration: initialization, condition, and iteration
- The tapering effect is where the number of spaces decreases and asterisks increases until we reach it's center.