Program to Convert dict to CSV in Python
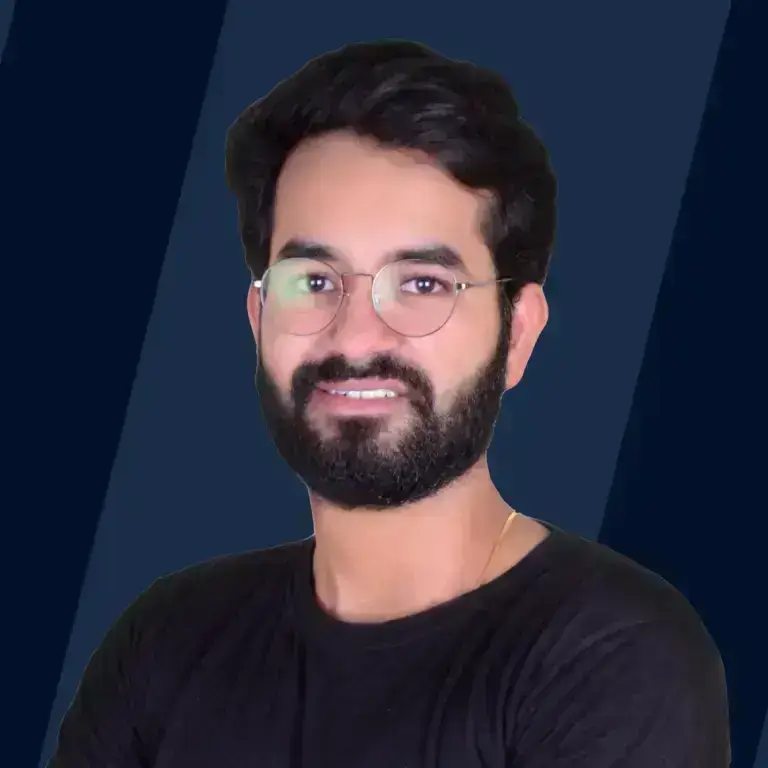
Overview
Python provides various methods to convert all the data in the dictionary to a CSV file. Some popular methods include using Pandas and CSV modules. A CSV(comma-separated values) file is the most common and popular file format. It contains comma-separate values, whereas a dictionary is a built-in data structure in Python that contains key-value pairs separated by commas.
Dict Files in Python
The dictionary stores the data in the form of key-value pairs. It's a collection that is ordered, changeable, and does not allow duplicates.
Things to keep in mind while working with a dictionary in Python:
- The key must be only a single element.
- The keys are case-sensitive, i.e., the same name in either uppercase or lowercase will be treated differently.
- The keys in the dictionary are always unique and must be of an immutable data type, such as strings, numbers, or tuples.
- The values in a dictionary can be of any data type.
For example:
Note: You can read more about the dictionary in Python here.
CSV Files in Python
A CSV(comma-separated values) format is one of the simplest data storage methods. To create a CSV file, save the file with a .csv extension.
Case 1: Open the notepad and write some comma-separated text in the file. Here, , is a delimiter. You can write the text given below.
Then save the file with the .csv extension. Open the file, and you will see that the above data has been organized into columns and rows.
The above image shows that all the comma-separated data has been converted into tabular form.
Case 2: Open excel, enter some data, and save the file with a .csv extension. Below is the sample data that you can write to your excel sheet.
Now, open the above CSV file with notepad, and you will see that all the data has been arranged as comma-separate in the text file.
Note: You can read more about the CSV file in Python here.
Different Methods to Convert Dict to CSV in Python
Now, we will see different methods to convert a dict to CSV Python. We will see how to use the CSV and Pandas modules for this conversion. But before using these functions, let's see how to convert dict to CSV without using these modules.
In the below code, we create a dictionary that has some data. First, we will extract all the keys and make a header for the CSV file. Then iterate over the list of dictionaries and write all the values in the CSV file.
Code:
Output:
In the above output, you can see that in row 1 we have all the keys, and then a new line, and then from row 2, we have all the values for the particular key.
Using CSV Module
Suppose we have a list of dictionaries we want to export into a CSV file. To do this, follow the steps below:
Step 1: Import the CSV module.
Step 2: Create a list of dictionaries
Step 3: Make a list of keys (this list will work as a header).
Step 4: Open the CSV file in write mode. The DictWriter() function creates a write object and maps a dictionary into rows. It takes file object and header as parameters. After creating an object, write a header in a CSV file using the writeheader() function.
Step 5: Iterate through all the dictionaries in a list and write them in a CSV file.
Code:
Output:
Note: As you have seen above, after writing the header using the writeheader() function, we are iterating through all the dictionaries in a list to fetch the values and insert them into a CSV file.
But, is there any function that can do this just by passing the name of the dict?
Yes, we have one more built-in function, writerows() that is also a part of the CSV module. This function only converts a list of dictionaries into a CSV file. Let's see how we can do this easily.
Code:
Output:
You can see that both outputs are the same. You can use either the writerows() or writerow() function.
Using Pandas Module
Using the Pandas library is one of the easiest ways to convert a Python dict to a CSV file. Suppose we have a list of dictionaries we want to export into a CSV file. To do this, follow the steps below:
Step 1: Import the Pandas Module.
Step 2: Convert the list of dictionaries to a Pandas dataframe. A Pandas dataframe is like a 2D array or a table with rows and columns.
Step 3: Now, convert the pandas dataframe to a CSV file using the to_csv function.
Code:
Output:
Now, in the above output image, you can see that we are also getting the index in the 1st column, like 0, 1, and 2. If you don't want to show these indexes, then you can just mark them as false: index = False
Output:
You can see from the above output image that we have marked the index as false and now the relevant data remains.
Conclusion
Let’s summarise our topic Dict to CSV Python by discussing some important points.
- Python provides various methods to convert all the data in the dict to CSV Python. Some popular methods include using Pandas and CSV modules.
- The dictionary stores the data as key-value pairs. It's a collection that is ordered, changeable, and does not allow duplicates.
- A CSV(comma-separated values) format is one of the simplest data storage methods. To create a CSV file, save the file with a .csv extension.
- Using CSV module: Import CSV module -> Create a list of dictionaries -> Make a list of keys -> Write a header in a CSV file using the writeheader() function -> Iterate through all the dicts and write in a CSV file.
- Using Pandas module: Import Pandas module -> Convert the list of dicts to a Pandas dataframe -> Pandas dataframe to CSV file using to_csv function.