Python Dictionary Comprehension
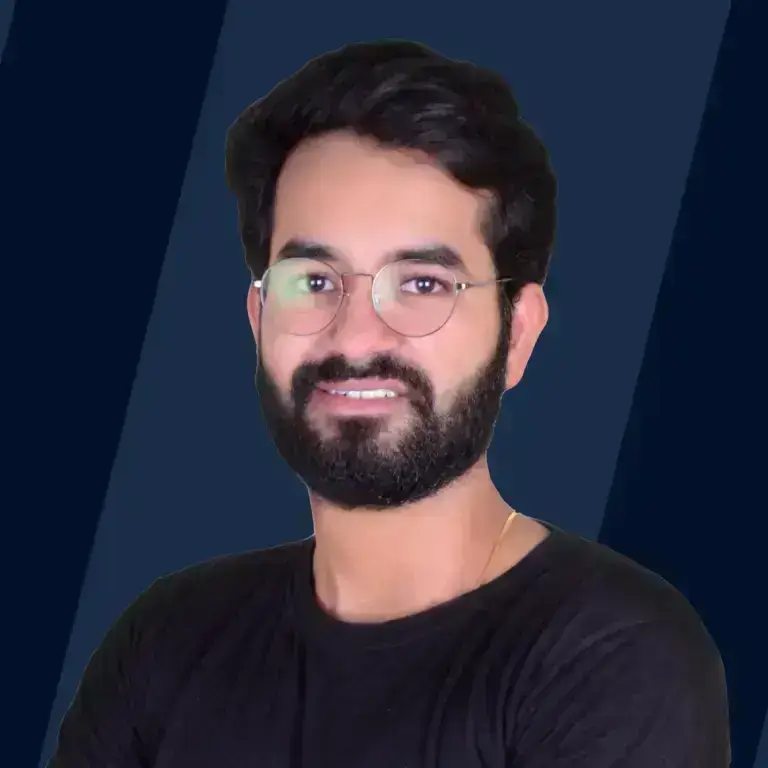
What is Dictionary Comprehension in Python?
Dictionary comprehension in Python is a concise way to create dictionaries. It allows you to generate dictionary elements from an iterable in a single line, making your code cleaner and more readable. This method is similar to list comprehensions but for dictionaries.
Python Dictionary Comprehension: Example
Let's say you have a list of fruits and their prices, and you want to create a dictionary where the fruit names are the keys and their prices are the values. Instead of using a loop, you can achieve this in one line with dictionary comprehension.
Output:
In this example, fruit_prices will be a dictionary that maps each fruit to its price, created efficiently through dictionary comprehension.
Using fromkeys()
The fromkeys() method is used to create a new dictionary with keys from a given sequence and a single value for all of them. This method is not a direct example of dictionary comprehension, but it's a useful technique for initializing dictionaries.
Example:
Output:
Using Dictionary Comprehension to Make a Dictionary
Dictionary comprehension is a method to succinctly construct a dictionary from a sequence or range. It's a powerful way to create dictionaries dynamically.
Example:
Output:
Using Dictionary Comprehension Using Data from Another Dictionary
You can also use dictionary comprehension to create a new dictionary by manipulating the keys and values of an existing one. This approach is very flexible, allowing for complex transformations.
Example:
Output:
In these examples, we see the versatility of dictionary comprehension and methods like fromkeys() to efficiently create and manipulate dictionaries in Python.
Using Conditional Statements in Dictionary Comprehension
Dictionary comprehension in Python can be enhanced with conditional statements, allowing for more refined control over which elements to include or how to modify them. This feature makes dictionary comprehension even more versatile, enabling complex transformations based on conditions.
Example:
Suppose you have a dictionary of stock items with their quantities and you want to create a new dictionary with only those items that have a quantity greater than 100.
Output:
In this example, the dictionary comprehension iterates through the original stock dictionary, and the conditional statement if value > 100 ensures that only items with a quantity greater than 100 are included in the filtered_stock dictionary. This method efficiently filters data and constructs a new dictionary based on specific criteria, showcasing the power and flexibility of using conditional statements within dictionary comprehensions.
Nested Dictionary Comprehension
Nested dictionary comprehension involves creating a dictionary with dictionaries as its values, using a single expression. This advanced technique is useful for structuring complex data, allowing for efficient data organization and manipulation within a compact syntax.
Example:
Imagine you're organizing information about students in different grades, each with their own set of scores. You want to create a nested dictionary where each key is a grade, and its value is another dictionary containing student names as keys and their scores as values.
Output:
In this example, the outer dictionary comprehension iterates through each grade, and for each grade, a nested dictionary comprehension creates a dictionary of student names to scores. This method efficiently organizes complex hierarchical data, demonstrating the power and flexibility of nested dictionary comprehension in Python.
Advantages of dictionary comprehension in python
- Dictionary comprehension allows for the creation of dictionaries in a single, concise line of code, reducing the need for multiple lines and loops.
- When familiar with the syntax, dictionary comprehensions make the code more readable and straightforward by expressing the intention clearly.
- It offers a slight performance advantage over a manual for-loop for creating dictionaries due to optimization in Python's implementation.
- It allows for the inclusion of conditional logic within the comprehension, enabling complex data transformations and filtering without additional code.
- It can be used for creating new dictionaries from iterables, transforming the keys and values of existing dictionaries, and nesting to create complex data structures.
Conclusion
- It streamlines the process of creating dictionaries, making code more concise and easier to maintain.
- The syntax of dict comprehension in python is clear and expressive, making the code's purpose immediately apparent to readers.
- Incorporating conditions into dict comprehension in python allows for sophisticated data filtering and transformation directly within the comprehension itself.
- The ability to nest dictionary comprehensions enables the construction of intricate data structures in a straightforward and readable manner.
- Dict comprehension in python are not only about cleaner code but also about performance, offering a more optimized way of creating dictionaries compared to traditional methods.
Learn More
To learn more refer Python Comprehensions