Difference Between Array and Structure in C
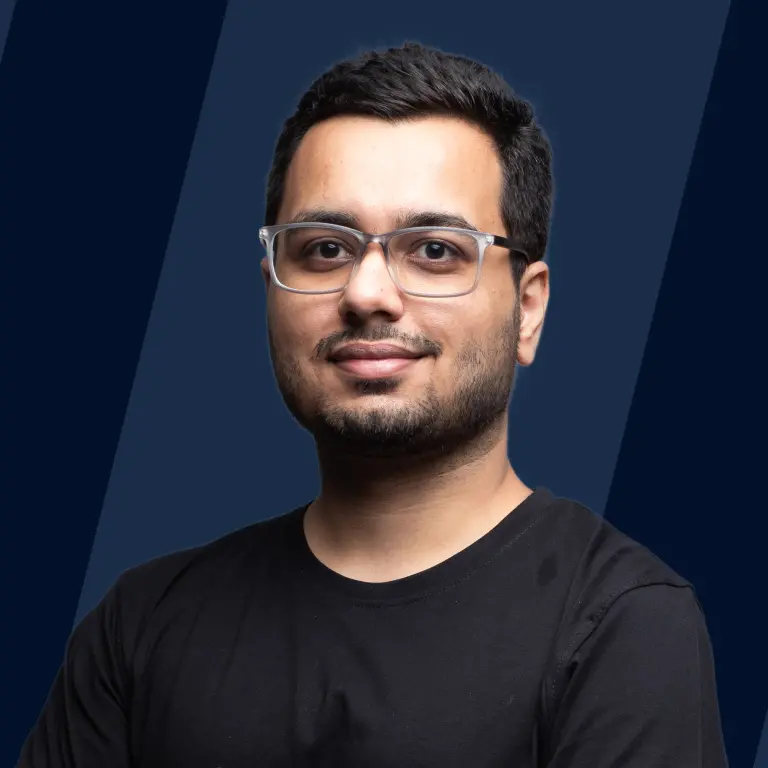
In C programming, arrays and structures are fundamental concepts used for storing data. The primary difference between an array and a structure lies in their data storage and flexibility. Arrays can store elements of the same data type, whereas structures allow storing different data types as part of a single unit. Additionally, array sizes are defined at declaration using square brackets, while structure sizes are determined by the number of elements declared within the structure. Now, let’s delve deeper into the concepts of array and structure in C programming.
Array in C
We can define an array as a data structure that stores data or elements that belong to the same data types. While defining an array, we can declare its size, which must be written in numbers within a square bracket that is mainly preceded by the name of the array, which also states that an array can store data and memory allocation dynamically. It is mandatory for the programmer creating an array to mention the number of elements that the array must be stored; hence, this gives the array a fixed length.
Let us consider an example below:
As seen in the above example we have declared an array of integer data types and having elements at max 20. This means that the array cannot store more than 20 elements where the first element will start from the zero indexes and the last element will fall at index 19. In addition to this, all the elements will be stored in the contiguous memory location as the property of an array defines. Hence, an array is said to have a static memory allocation.
Hence, an array can be defined as a collection of elements that are stored at contiguous memory locations.
let us see a simple program to create an array:
Code:
Output:
Explanation: In the above example, a simple array is defined in C language. The age is an array that can store ten integer values as we have initialized it with ten marks. We then use the ‘for loop’ to iterate the array where the i variable increments to 9 as the value starts from 0 indexes. After which it will consecutively print the age in the array sequentiall. The element at the start of the array is 2 and the array ends at 33.
Structure in C
While studying C programming language, having a good understanding of the structure is also important. We can define a structure as a single variable that stores data or elements that belong to both (different and same) data types. It is also described as a set of variables with dissimilar data types. While defining a structure the size is defined by the number of elements that are declared at the time of defining the structure. The programmer needs to declare the number of elements at the time of the creation of the structure itself using the dot (.) operator.
Let us consider an example as below:
As seen above, we have declared a structure with continuing variable data types and the structure Corporate is acting as a container to store the various details of the employee in it.
Hence, a structure is a user-defined type of data where a data collection for various data types can be stored. We use it grouping items of varied kinds into a single one.
let us see a simple program to create a structure:
Code:
Output:
Explanation:
In the above example, a simple structure is defined in C language. The structure Corporate contains two properties: employee_name (char) and employee_id (int). We described the c1 as the structure type variable in the main method where we have assigned the value 33 to the id property of the structure. We use the strcpy function to allow the string “Shalini” to get copied as the name property of the structure. We can access the properties by using the dot operator while finally printing the name and id of the employee in the print statement.
Quick Tip: To learn more about structure in C, please refer to the module shared in the link structures in c as it helps to broaden your understanding of structure in C and helps you understand this module at a glance.
Difference Between Array and Structure
While we studied the array and structure above, let us compare and study in-depth the difference between array and structure as explained below:
ELEMENTS OF COMPARISON | ARRAY | STRUCTURE |
---|---|---|
Definition | An array can be defined as a container where a collection of variables can be stored that are of the same data type as the array and also support contiguous memory allocation. | A structure can be defined as a container where a collection of variables can be stored that are of both (same and different) data types. |
Syntax | type array_name[size]; | struct struct_name{ type element1; type element2; ... } variable1, variable2, ...; |
Operator | The operator that is used while we declare an array and the elements accessing it is a square bracket "[ ]". | The operator that is used while we declare a structure and the elements accessing it is a dot operator".". |
Memory | The array stores its data in a contiguous memory location. | The structure may not store its data in a contiguous memory location. |
Pointer | The array name is referred to as the first element in the array hence we can say that an array is a pointer. | The structure name does not highlight the first element of the structure hence, the structure name is not a pointer. |
Access | All the elements in the array are accessed by their index number. | All the elements in the structure are accessed by their actual names. |
Size | The elements found in the array are of the same size. | The elements found in the structure are of different data types. |
Objects | No objects or any instances are created in the array. | Instances or structure variables can be created in the structure. |
Accessing | To access an array it usually takes less time | To access a structure it comparatively takes longer. |
Bit field | In an array no bit field can be defined | In a structure bit field can be defined |
Keyword | No keyword is used to declare an array | "struct" is a keyword that is always used while declaring a structure. |
User-defined | As an array is directly declared we don't need to specifically define it. | Structure is user-defined datatype. |
Performance (searching and accessing) | The performance for an array is always higher as the data types are of the same type which makes searching and accessing any element faster. | The performance for a structure is always lesser than the array's performance as the data types are of different data types which makes searching and accessing any elements slower. |
Key Differences: Array Vs Structure
As we studied all the aspects where we covered how an array is different from a structure, let us study some of the key differences between array and structure as below:
-
An array is defined as a collection of variables where elements have a similar data type whereas in a structure it is also a collection of variables but here the elements are of different data types.
-
The array stores its variable in a contiguous memory location which means that the data is stored in a memory model where the memory blocks hold consecutive addresses whereas in the structure the variables may not be stored in a contiguous memory location.
-
As the data type in an array is of similar data type, therefore, searching and accessing singular or multiple elements is faster as it is easier to find the elements using its index number that shows their position in that array whereas if we want to search and access elements in a structure then it is a bit difficult and takes a longer time as the datatype is different and the searching will happen using structure name that is followed by a dot followed by a variable name.
-
The operator used in an array is square bracket "[ ]" whereas in the structure the operator used is a dot operator "."
-
We do not make use of any keyword while declaring a while for declaring a structure we must provide the keyword 'struct'.
-
As the elements in a structure are of different data types hence the size of all the elements in a structure is different whereas as an array has the same data type all the elements found in an array are of the same size.
-
A structure is user-defined datatype while an array is not a user-defined data type.
Conclusion
- Arrays and structures are both used as containers for data in C programming.
- The main difference lies in their data storage and flexibility: arrays store elements of the same data type, while structures allow different data types.
- Arrays have fixed sizes defined at declaration, while structure sizes depend on the number of elements declared within the structure.
- Arrays use square brackets for access, while structures use the dot operator.
- Arrays have better performance for searching and accessing elements due to their homogeneous data types, while structures may require more time for the same operations due to their heterogeneous data types.