Difference Between Break and Continue Statement in C
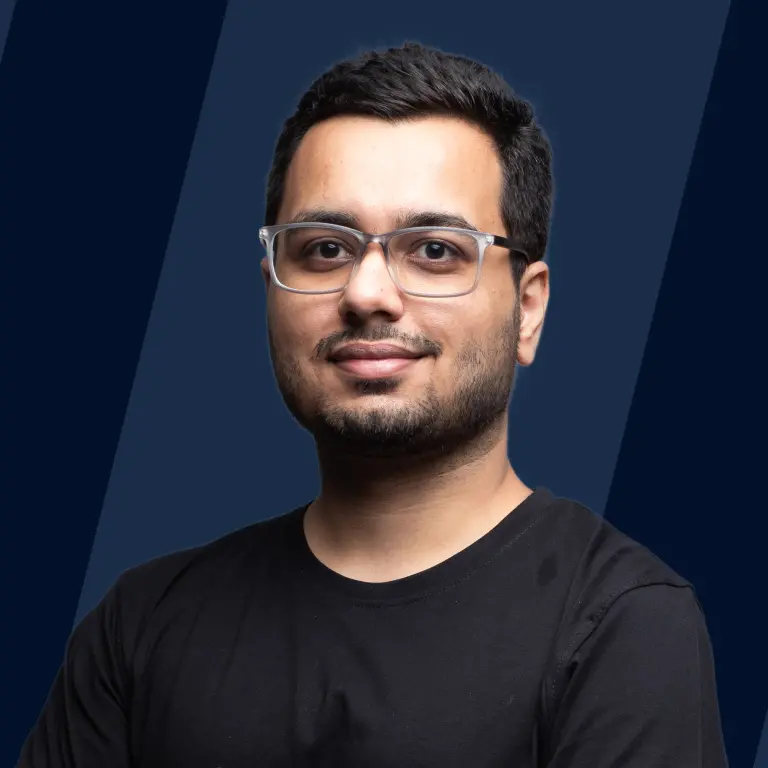
Overview
The "continue" statement in C programming jumps to the next loop iteration, bypassing the following code in the current iteration. On the other hand, the "break" statement completely stops the loop, enabling the program to depart the loop's scope. These statements govern loop flow, allowing for more efficient code execution.
Break Statement
The break statement in C programming is essential for improving loop control. Consider a situation where you need to escape a loop early - here is where the break statement comes in handy.
Consider this: You're iterating through a loop when a condition occurs that requires an instant exit. This is when you use the break statement. It immediately ends the loop's execution, allowing you to go to the next code block.
Here's a snippet to illustrate:
In this example, when i hits 5, the break expression takes effect, terminating the loop. As a result, the output highlights the loop's interruption at that moment.
In summary, C's break statement provides an efficient and elegant way to regulate loops, optimizing code logic and execution.
Continue Statement
The continue statement is a significant feature in C programming that allows for exact control over loops. When inserted within the body of a loop, it informs the program to skip the remaining code in the current iteration and move straight to the next iteration. This is handy when specific conditions exist, and you want to avoid doing certain computations or actions while keeping the loop running.
Take the example of iterating through a list of values. The continue statement allows you to define criteria under which the current iteration should be stopped, and the program will go to the next iteration. This improves the loop's performance and streamlines the code's logic.
Here's a simple code example:
When i equals 3, the continue statement avoids writing three and goes right to the next iteration, keeping the loop's flow intact. The result will be 1 2 4 5.
The C continue statement provides an intuitive way to manage loops and adapt their behaviour based on specified situations, improving code efficiency and readability.
There are situations where breaking out of multiple nested loops might be necessary. Let's consider a concrete example using a C code snippet:
In this example, we have two nested for loops. The continue statement is not suitable for breaking out of both loops when a specific condition is met (i.e., when i is 2 and j is 2). Instead, we use a goto statement labelled end_of_loops to exit both loops simultaneously. While the use of goto should be sparing and controlled, it can provide a clean and efficient solution in certain situations, like breaking out of nested loops.
Difference Between Break and Continue Statement
Aspect | Break Statement | Continue Statement |
---|---|---|
Purpose | Terminates the current loop entirely, exiting the loop block. | Skips the remaining code in the current iteration and advances to the next. |
Affected Loops | Applicable to all loops: for, while, and do-while. | Applicable to for, while, and do-while loops. |
Behavior | Stops loop execution once the break is encountered. | Jumps directly to the next iteration's loop condition check upon encountering a continue statement. |
Nested Loops | Break affects only the innermost loop where it's placed. | Continue affects the innermost loop containing it without affecting outer loops. |
Usage Scope | When a specified condition is satisfied, the loop must be terminated prematurely. | Useful when skipping some iterations based on particular criteria without quitting the loop. |
Control Flow | Transfers control immediately after the loop block. | Shifts control to the loop's iteration condition check, skipping the remaining code in that iteration. |
Break allows you to prematurely exit a loop prematurely when a specific condition is met. It's valuable for terminating a loop early if, for example, you find the desired value in a search or want to stop processing when a specific condition is satisfied.
Continue, on the other hand, is used to skip the current iteration of a loop and move to the next one. This can be beneficial for avoiding unnecessary computations or iterations when certain conditions are met within the loop.
In contrast, the return statement exits a function and returns a value to the calling code. It doesn't affect loops directly but controls the flow of the entire function.
Programmers must be cautious when using break and continue. Common mistakes include forgetting to update loop variables, leading to infinite loops or incorrect results. Excessive use of break statements can make code less efficient by disrupting the natural flow of loops. In contrast, the judicious use of continue statements can optimize loop execution by skipping unnecessary operations in specific iterations. It's crucial to employ these statements thoughtfully to maintain code readability and performance.
Conclusion
- When a given condition is fulfilled, the break statement ends the nearest enclosing loop (such as for, while, or do-while). It aids in escaping the loop early.
- The continue statement allows the current iteration's remaining code to be skipped and jumps to the next iteration. It helps to avoid specific loop iterations.
- The break statement allows you to escape a loop early, but the continue statement will enable you to skip the whole of one iteration and proceed to the next.
- When used in nested loops, the break command ends the innermost loop without impacting the outer loops.
- continue in nested loops skips the remaining code for the current iteration of the innermost loop and moves on to the next iteration.
- Each statement has a particular function in directing loop execution depending on different situations. Understanding these distinctions is critical for efficient programming and loop behaviour optimization.