Difference between Constructor and Method in Java
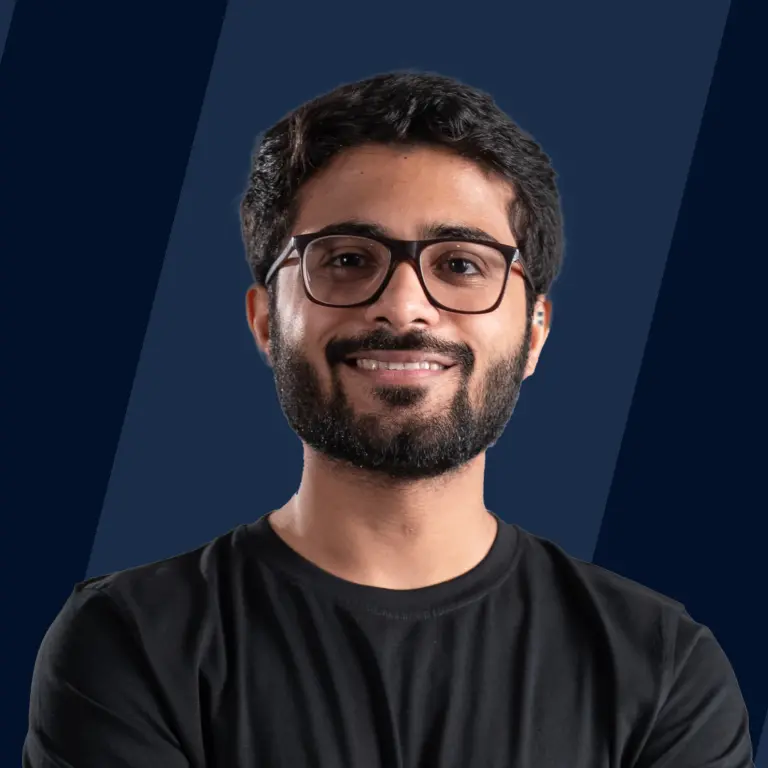
Overview
Constructors and methods are critical building elements in Java programming, each serving a specific purpose. A constructor takes the front stage during object instantiation, bringing an object to life by initializing its state. On the other hand, the methods are created for specified actions or calculations on objects. Constructors are called implicitly during object formation, whereas methods are called expressly to activate their functionality. Constructors create objects, while methods drive an object's behavior, emphasizing the distinction between object creation and ongoing behavior.
Constructors in Java
Constructors are the foundation upon which objects are formed in Java programming. A constructor is a particular function within a class that helps with object creation and initialization. It functions similarly to a blueprint, outlining how an object should be created and its initial state. Constructors have the same name as their associated class, but unlike conventional methods, they do not have a return type.
Constructors allow developers to define default settings and guarantee correct resource allocation before using an object. There are two kinds of constructors in Java: default constructors and parameterized constructors. Default constructors are called automatically when an object is created, whereas parameterized constructors allow developers to give particular values as arguments to the object during instantiation.
To learn more about Constructors in Java, please click Constructors in Java
Example
The class Car in this illustrated code snippet has both a default and a parameterized constructor. The former sets the properties of the automobile to default settings, but the latter allows developers to customize these attributes during object creation. These constructors are critical components of Java's object-oriented paradigm, providing the foundation for efficient and organized programming.
In a nutshell, constructors in Java act as architects, laying the basis for object creation. Constructors, with their many kinds and capacities, give the flexibility and structure required for molding things to fulfill unique programming demands.
Methods in Java
Methods are key building blocks of Java programming, allowing developers to design efficient and organized code. Methods allow specialized functionality to be encapsulated within a self-contained unit, boosting reusability and maintainability. This simple guide explains methods in Java and includes an instructive code-based example.
At its heart, a method is a piece of code that performs a specific task and may be called any time it is needed. It takes parameters as input, processes them, and provides a result. Java methods comprise two parts: the method declaration and the method body. The method's name, return type (or void if no return value), and parameters are all specified in the declaration. The body is where the method's functionality is implemented.
A Java method has a structure like this:
To learn more about Methods in Java, please click here
Example
Consider this simplified example demonstrating the creation and usage of a method:
In this example, we created a class called "Calculator" with a method called "add". The method takes two integer inputs, sums them, and returns the result. The "main" method shows how to call the "add" function, pass data, and get the calculated total.
Java methods provide code modularity, creating clearer organization and easier debugging. They allow developers to focus on certain tasks, improving code readability and maintenance. Understanding the fundamentals of Java methods allows programmers to harness their power to create powerful and well-organized programs effectively.
Finally, methods are essential in the Java programmer's armory, promoting reusability and readability. Developers who understand how to create and use methods may confidently navigate the complexities of software development.
Constructors vs. Methods in Java
Understanding the difference between constructors and methods is critical when starting with Java programming. Within a class, these two essential components fulfill unique functions, preserving the integrity and functionality of your code. Let's debunk Constructors and Methods in Java and emphasize their distinct functions.
The main difference is in their duties and invocations. Constructors facilitate object formation by assuring appropriate initialization, whereas methods perform actions and calculations on objects. Constructors have no return type, whereas methods specify their return type explicitly.
Constructors are responsible for correct object construction and setup, whereas methods enable object interaction and behavior execution. While constructors are automatically called when an object is created, methods must be called explicitly. It's critical to pick between them based on whether you're building up beginning conditions or executing actions on existing objects. This understanding provides a good basis for building efficient and maintainable Java code.
Finally, constructors and methods in Java each play an important part in class design and object handling. Constructors set the framework for object formation, whereas methods allow for the execution of specific activities. A comprehensive knowledge of these distinctions enables developers to design efficient, well-organized, and useful Java programs.
FAQs
Q. What is the primary distinction between constructors and methods in Java?
A. Constructors are specialized methods that create objects and initialize them, whereas methods are functions that execute operations on objects or return data.
Q. Can constructors be inherited and overridden in the same way that methods can?
A. Constructors are not passed down through the inheritance tree, but they are invoked. Constructors of the superclass, unlike methods, are not immediately available in the subclass.
Q. How do constructors and methods differ in terms of return types?
A. Constructors don't have a return type, not even void because they automatically return the class instance but methods have their return types.
Conclusion
- A method is a piece of code that performs a certain purpose and may be called at any time needed. It takes parameters as input, processes them, and provides a result.
- Constructors allow developers to define default settings and guarantee correct resource allocation before using an object. Java provides two kinds of constructors.
- Constructors are specialized methods that create and initialize objects, whereas methods are functions that execute operations on objects or return data.
- Constructors are not passed down through the inheritance tree, but they are invoked. Constructors of the superclass, unlike methods, are not immediately available in the subclass.
- Constructors are responsible for correct object construction and setup, whereas methods are responsible for object interaction and behavior execution.
- Constructors have no return type, whereas methods specify their return type explicitly.