Difference between Comparing String Using == and .equals() Method in Java
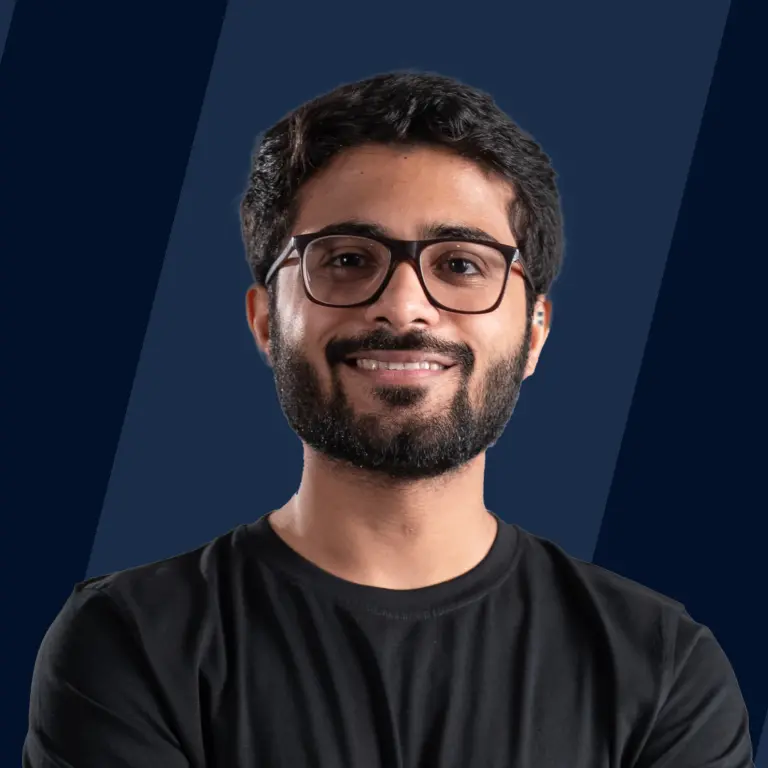
Let's say we want to check if two objects or data items are the same. In Java, we have the "==" operator and equals() method for doing this. In this article, we will learn the difference between == and equals in Java.
The equals() method in Java compares two objects for equality. It is defined in the Object class in Java. The equals() method compares characters by characters and matches the same sequence present or not in both objects. The == operator matches memory references and the characters.
- The equals() method is a function while == is an operator.
- The == operator is generally used for memory references comparison.
- If a class doesn't implement the equals method, it will inherit and utilize the implementation defined in the nearest parent class that has overridden this method.
Example
Output:
Equality Operator(==)
In Java, we use the "==" operator to compare two variables of primitive data type and also compare objects. Just like equals() method, "==" also returns boolean output. Since "==" is an operator, it is not overrideable, so we avoid using it to compare two objects. It can be used to compare two variables of int, char, byte, short, long, boolean, float, and double types.
Let us look at an example of this.
Example: Comparison of two integers.
Code:
Output:
Explanation:
- Here, we define five integers. For the first comparison, we can see that n1 is 10 and n2 is 20, so the result is false.
- For the second comparison, we can see that since n4 and n5 both are 20, we are getting true as a result.
- We have used the extra variables to show that the == operator does not compare the memory locations.
Note:
- Using the "==" operator to compare two strings is not illegal, and hence, it will not cause any errors.
- But since it compares the locations of the strings and thus, it will not give the correct/desired output.
Java String equals() Method
We use equals() in Java to compare two objects. It is defined in java.lang package. From the names, we can understand that the equals() method in Java checks for some equality in the two objects. The equals() method, for objects, checks if two objects have the same content or not.
Signature of the equals() method in Java:
Here, obj2 is the object compared against the object that calls the equals() method. Let's understand this with an example.
Here, we are trying to compare obj1 with obj2. The second object is the object passed as a parameter in the signature of the equals function. If obj1 is equal to obj2, the equals() method returns true; otherwise, it returns false.
Example:
Output:
Points to Remember:
- Since we are comparing the strings char by char using the equals() method, it is an O(n) operation where n is the size of the shortest string.
- For comparing two strings without case-sensitiveness, we can use equalsIgnoreCase() function in Java.
- For lexicographically calculating the difference between two strings, check out the compareTo() function in Java.
Difference between Comparing String Using == and .equals() in Java
Let us look at the difference between == and .equals() in Java:
"==" Operator | equals() Method |
---|---|
"==" is a Java operator. Hence, it can not be overridden. | equals() is a method in Java; hence, it can be overridden. |
It is generally used to compare two variables of primitive data types but can also be used to compare objects. | It is used to compare objects. It can not compare primitive data types. |
It compares the memory location of the objects. For the String objects, it compares them character by character if memory location equality fails. | It compares the data of the two variables, but in the case of objects, it compares the memory locations. |
It takes O(1) time for comparison. | It takes O(1) time for normal objects and O(n) time for String objects. |
It throws a compile time error if the two variables are not of the same data type. | It returns a "false" value if the objects are not of the same type. |
Conclusion
- In this article, we learnt the difference between == and equals in Java.
- The equals() method in Java is used to compare two objects based on their sequence of characters.
- == is a Java operator, generally used to compare primitive data types but can also be used to compare objects.
- == operator compares the data for primitive data types and addresses for objects.
- equals() method is overridable while == is not.
FAQs
Q: What is the == operator in Java?
A: The == operator in Java is used to compare the values of two objects or primitive data types for equality.
Q: What does <<= mean in Java?
A: In Java, the <<= operator is a bitwise left shift and assignment operator.
Q: What are the rules of equals() method?
A: The equals() method in Java is used to compare the contents of two objects for equality, and it should adhere to reflexivity, symmetry, transitivity, consistency, and handle null values.