The Difference between Iterator and Generator in Python
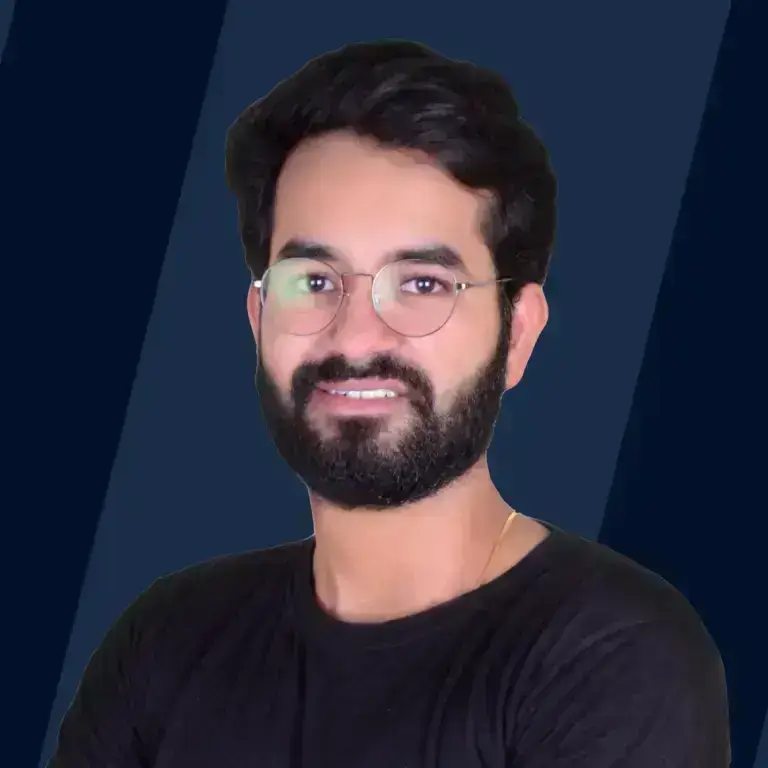
Overview
Python is lauded for its coding simplicity and efficiency. Two key tools for managing data sequences in Python are iterators and generators. In this article, we will go through the difference between an iterator and a generator in Python.
Iterator
An iterator is a fundamental Python construct, serving as an object that facilitates the traversal of a container or sequence, one element at a time. It offers a means to access the elements within a collection without exposing the collection's underlying structure. In Python, iterators are crafted by implementing two crucial methods: __iter__() and __next__(). The __iter__() method returns the iterator object itself, while the __next__() method provides the subsequent value within the iterator.
Syntax:
Example:
In this example, we are demonstrating the usage of a custom iterator, MyIterator, to iterate through and print the elements of a list (my_list).
Code:
Output:
Explanation:
In this example, we define a custom iterator, MyIterator that iterates through a list of integers. The __iter__() method returns the iterator object itself, and the __next__() method is used to access the next element in the list until the end is reached.
Generators
In contrast, a generator is a specialized type of iterator. It is defined using a function that incorporates one or more yield statements. Generators empower developers to traverse potentially extensive data sequences without the need to store the entire dataset in memory. When a generator function is invoked, it returns a generator object that facilitates the iteration through the values generated by the yield statements.
Syntax:
Example:
In this example, we are showcasing the use of a generator function, my_generator, to yield and print each element from a list (my_list) efficiently.
Code:
Output:
Explanation:
In this example, we define a generator function my_generator that yields each element from a list. When we iterate through the generator object my_gen, it produces values on the fly without storing the entire list in memory.
Note: Python 3.3 introduced a powerful syntax enhancement known as yield from designed to simplify working with nested generators and enhance code readability. This feature is particularly valuable when dealing with complex data processing tasks or recursive algorithms.
Table of Difference between Iterator vs Generators
In the table below, we summarize the difference between an iterator and a generator in Python:
Aspect | Iterator | Generator |
---|---|---|
Implementation | Implemented as a class | Implemented as a function |
Memory Efficiency | May consume more memory | Consumes minimal memory |
Simplicity | Requires more code for iteration | Simpler and concise code |
Use of yield statement | Not used | Essential for defining generators |
Data Generation Efficiency | Generates data as needed | Generates data on-the-fly |
Use Cases | Suitable for small datasets | Suitable for large or infinite datasets |
When to Choose Which?
This section describes the difference between an iterator and a generator in python.
Iterators
- Use iterators when dealing with small datasets or collections.
- When you need precise control over iteration logic.
- When you want to encapsulate the iteration logic within a class.
- When you don't need lazy evaluation and can load all data into memory at once.
- When you need to support multiple independent iterators over the same data.
- Ideal for organized, controlled iteration over finite datasets.
- Useful for custom iteration strategies and maintaining state.
Generators
- Use generators for large or infinite datasets to conserve memory.
- When you want to create iterable sequences without defining a custom class.
- When you need lazy evaluation, generate values only as needed.
- When working with functions that return an iterable.
- When implementing pipeline-style data processing, chaining multiple generators together.
- Efficient for processing data on the fly without loading it all into memory.
- Effective for handling infinite data streams or large datasets.
Conclusion
- Iterators offer precise control over data traversal, making them suitable for organized, controlled iteration over finite datasets.
- Generators conserve memory and effectively handle large or infinite datasets, making them ideal for efficient data processing.
- Generators simplify code structure with concise syntax, while iterators require more code for iteration logic.
- Generators enable lazy evaluation, generating values only as needed, which is valuable for conserving memory.
- Python 3.3 introduced the "yield from" syntax, enhancing code readability when working with nested generators.
- Iterators are suited for small datasets, while generators shine in scenarios involving large or infinite datasets.
- Python's simplicity and versatility are evident in its constructs like iterators and generators, empowering developers to handle data sequences efficiently.