Difference Between Sort and Sorted in Python
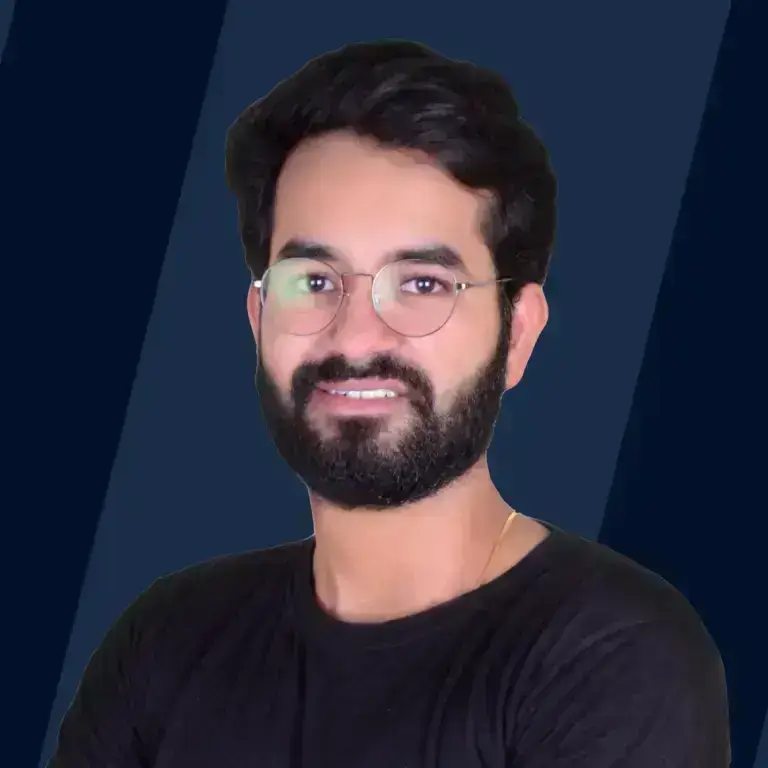
Python language supports two built-in methods to perform the sorting operation on any sequence.
These two methods are given below:
- sort()
- sorted()
The sort() function returns nothing and changes the original sequence, while the sorted() function creates a new sequence type containing a sorted version of the given sequence.
Let's discuss the above two methods of our topic difference between sort and sorted in Python in detail.
sort() function in Python
- The sort() function is used to perform the sorting operation, like the Sorted Function, but is very different in terms of its uses.
- The sort() function is a list method, i.e., it can only be used to sort a list.
- The sort() function returns nothing, which means it makes changes to the object passed, i.e., the original sequence.
Syntax of sort() function in Python
Parameters of sort() function in Python
Two parameters are passed in the sort () built-in method, which is as follows:
- Key: It is an optional parameter. This works as a basis for comparison while sorting.
- Reverse: It is an optional parameter. It is a boolean variable, i.e., if set to True, it sorts the variable in descending order, else it sorts in ascending order. The default value of reverse is false.
Return Type of sort() function in Python
Sort() function makes changes to the original sequence. Therefore, its return type is None.
sorted() function in Python
- The sorted function in Python is one of the most versatile functions.
- It can be used to sort a list, set, and dictionary as well. It works on sequences, such as lists, tuples, or strings, as well as collections, such as a dictionary, set, or frozenset.
- It by default sorts in ascending order but can also sort in descending using the reverse parameter, i.e., if the reverse parameter is set to true, it sorts the dictionary in descending order.
- The specialty of the sorted function is that it does not affect the original sequence of the object, i.e., it creates a sorted copy of the object and returns it.
Syntax of sorted() function in Python
Parameters of sorted() function in Python
Three parameters are passed in the sorted() method in Python, which are as follows:
- Iterable: This is the Python object that needs to be sorted. It can either be a sequence, i.e. a list, tuple, or string, or a collection, i.e., a dictionary, set, or a frozenset
- Key: It is an optional parameter. This works as a basis for comparison while sorting.
- Reverse: It is an optional parameter. It is a boolean variable, i.e., if set to True, it sorts the variable in descending order, else it sorts in ascending order. The default value of reverse is false.
Return Type of sorted() function in Python
The sorted function returns a list of sorted items from whatever iterable you pass in.
Sort vs Sorted in Python
Sort() method | Sorted() Method |
---|---|
The Sort() function only works on the list. | The Sorted()function can work both on Sequences and Collections. |
It sorts the object in-place, so it returns None. | It returns a sorted list of the Iterable passed. |
It sorts the original sequence, i.e., Inplace sorting. | It creates a sorted copy of the Python object. |
It takes 2 parameters, i.e., key and reverse. | It takes 3 parameters, i.e., iterable, key, and reverse. |
Syntax: list_name.sort(key, reverse=False) | Syntax: sorted(iterable, key, reverse = True) |
Examples of sort() function in Python
Example 1: Using sort() function with List in Python
Code:
Output:
Example 2: Using sort() function to sort in descending order
Code:
Output:
Explanation:
-
In the above code, we can observe that first, we create a list that stores a sequence of unsorted sequence numbers.
-
Then we apply the sort() method, which sorts the list in place.
-
And lastly, we will get the sorted sequence of numbers.
Examples of sorted() function in Python
Example 1: Using sorted() function with List in Python
Code:
Output:
Example 2: Using sorted() function with Set in Python
Code:
Output:
Example 3: Using sorted() function with Dictionary in Python
Code:
Output:
Example 4: Using sorted() function with Tuple in Python
Code:
Output:
Example 5: Using sorted() function with String in Python
Code:
Output:
As you can see from the above examples, that the sorted() function returns a list of sorted items from whatever iterable you pass in.
Conclusion
Let’s summarise our topic difference between sort and sorted in Python by mentioning some of the important points.
- Python supports two different built-in methods (sort and sorted method) to perform the sorting operation on any sequence.
- The main difference between sort and sorted in Python is that sort function returns nothing and makes changes to the original sequence, while the sorted () function creates a new sequence type containing a sorted version of the given sequence.
- A sorted function takes 3 parameters, i.e., iterable, key, and reverse, whereas a sort function takes 2 parameters, i.e., key and reverse.