Difference between Type Casting and Type Conversion
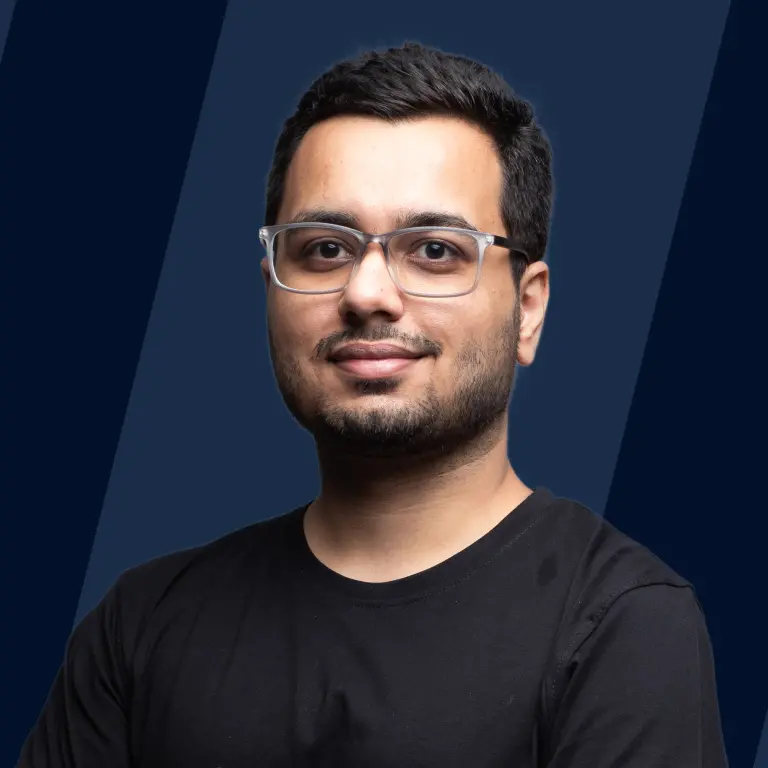
Understanding the difference between type casting and type conversion is critical in C programming. Type casting is analogous to a directive, allowing a variable to temporarily assume a new data type for a specified action. It does not change the original data but assures compatibility throughout the procedure. Type conversion, on the other hand, entails permanently altering the data type of a variable and storing the result.
Type Casting
In C, type casting is the process of explicitly altering a value's data type. It is critical to preserve data integrity and ensure the program performs as intended while interacting with various kinds of data. This function is very handy when doing arithmetic or comparison operations on variables of different data types.
Incorrect use of typecasting can lead to data loss and unexpected behaviour. For example, converting a floating-point number to an integer may truncate decimal values, potentially causing data loss.
Syntax
To perform type casting, you use the following syntax:
Here, (new_data_type) is the target data type you want to cast, and expression represents the value you want to convert.
Example
Let us now look at some examples for more clarity.
Example 1 - Integer to Float:
Suppose you have an integer value and need to store it as a float:
In this example, we cast the integer value 42 to a float, preserving the value but changing its data type.
Example 2 - Float to Integer:
Conversely, you can cast a float to an integer:
This will truncate the float's decimal component and save it as an integer.
Type Conversion
Data types are extremely important in the domain of programming. They specify the type of data that a variable may store and how the computer should handle that data. However, there are times when we need to change one data type to another, which is known as type conversion. Type conversion is a key notion in C that allows us to operate nicely with diverse data kinds.
In C, we use two types of conversion: implicit and explicit.
1. Implicit Type Conversion
The C compiler does implicit type conversion, also known as type coercion, automatically. It occurs when a value of one data type is assigned to a variable of another data type and the conversion is safe. As an example:
2. Explicit Type Conversion
Explicit type conversion, on the other hand, is programmer-controlled and requires the usage of casting operators. The following is the syntax:
Here's an example of explicit type conversion:
Example
Consider the following real-world example. You're coding code for a calculator, and the user enters a decimal number. If you're creating a basic calculator that only works with whole numbers, you may need to transform this input to an integer before calculating.
In this example, we use (int). to explicitly change the userInput from a double to an integer.
Use cases and Examples
Common examples include assigning an integer to a floating-point variable (int to float), combining strings and integers (string concatenation), or promoting smaller integer types (e.g., byte) to larger ones (e.g., int) without loss of data. These conversions are typically performed to facilitate operations or assignments while maintaining data integrity.
Difference between Type Casting and Type Conversion
Aspect | Type Casting | Type Conversion |
---|---|---|
Definition | Type casting is a process of explicitly changing the data type of a variable or an expression. | Type conversion involves changing data from one type to another, either implicitly or explicitly. |
Usage | Usually performed manually by the programmer using casting operators like (int) or (float). | Can be automatic (implicit) or manual (explicit) depending on the programming language. |
Purpose | Primarily used to enforce the specific data type for an operation or expression. | Used to ensure compatibility between different data types and for data manipulation. |
Examples | Casting from the float to the int: int x = (int) 5.67; | Conversion from string to int: int num = int.Parse("42"); |
Risks | May result in data loss or unexpected behavior if the new type cannot accommodate the original data. | Implicit conversions can lead to unexpected results if not handled carefully. |
Explicitness | Requires explicit developer intervention, making it clear when type changes occur. | Can happen implicitly, which can sometimes be less clear to developers. |
Syntax | Uses casting operators like (new_type) or functions provided by the languages, such as Convert.ToInt32(). | Uses language-specific functions or methods like ToString(), Parse(), or type constructors. |
Applicability | Commonly seen in statically typed languages such as C++ or Java. | Observed in both statically and dynamically typed languages such as Python or JavaScript. |
Compatibility | Works best when the target type is closely related to the source type, e.g., numeric types. | More adaptable and capable of handling conversions between a broader range of data formats. |
Performance | It is often quicker since it directly changes the type without creating a new object. | May involve the creation of a new object, potentially affecting performance. |
Conclusion
- Type Casting is mostly used for explicit data type changes that do not result in data loss. Type Conversion, on the other hand, entails changing data types, frequently implicitly, and may result in data loss.
- Type casting, through strict type rules, ensures data reliability. Poorly handled type conversion can compromise data integrity.
- To alter the data type, type casting uses explicit syntax such as (int)variable. Type conversion frequently uses built-in methods and may be less obvious in code.
- Type Casting may lead to runtime errors if not done correctly but helps catch issues early. Type Conversion can be less error-prone but may hide potential problems until runtime.