Directories in Python
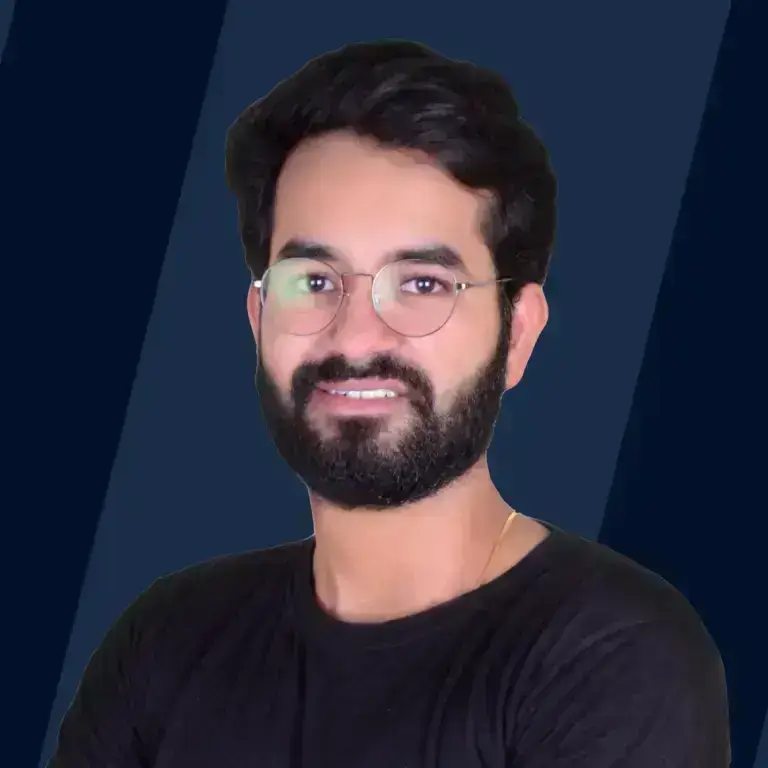
Overview
Directories, also known as folders, are essential for organizing and managing files on your computer. In Python, you can interact with directories using the os module, which provides various methods for creating, navigating, renaming, and deleting directories in Python. This article will explore these directory-related operations in Python.
Creating Directories
The os.mkdir() Method
The os.mkdir() method creates directories in python.
Code:
Explanation:
- We import the os module to access directory-related functions.
- We specify the new directory's name ("my_new_directory").
- The os.mkdir() method is called with the directory path as an argument, creating the directory.
The os.makedirs() Method
The os.makedirs() method creates directories in Python and any necessary parent directories.
Code:
Explanation:
- We import the os module.
- We specify a directory path that includes parent and child directories.
- The os.makedirs() method is called with the directory path as an argument, creating the directory and any necessary parent directories.
Navigating Directories
The os.chdir() Method
The os.chdir() method allows you to change the current working directories in Python.
Code:
Explanation:
- We import the os module.
- We specify the path of the desired directory.
- The os.chdir() method is called with the directory path as an argument, changing the current working directory to the desired location.
The os.getcwd() Method
The os.getcwd() method returns the current working directories in Python as a string.
Code:
Output:
Explanation:
- We import the os module.
- We call the os.getcwd() method, which returns the current working directory as a string.
- We print the current directory.
The os.path.join() Method
The os.path.join() method is used to construct file and directory paths in a platform-independent way.
Code:
Output:
Explanation:
- We import the os module.
- We use the os.path.join() method to construct a path by joining the directory and file components.
- The constructed path is then printed.
Renaming and Deleting Directories
The os.rename Method
The os.rename() method renames directories in Python.
Code:
Explanation:
- We import the os module.
- We specify the current and new directory names.
- The os.rename() method is called with the current directory name and the new directory name as arguments, renaming the directory.
The os.rmdir() Method
The os.rmdir() method removes an empty directory.
Code:
Explanation:
- We specify the directory to be removed.
- The os.rmdir() method is called with the directory path as an argument, removing the empty directory.
Listing Directory Contents
The os.listdir() Method
The os.listdir() method lists the contents of a directory, including files and subdirectories.
Code:
Output:
Explanation:
- The os.listdir() method is called with the directory path as an argument, and its contents are stored in a list.
- We print the list of directory contents.
Conclusion
- Python's os module provides powerful tools for working with directories, allowing you to create, navigate, rename, and delete them.
- You can use os.mkdir() to create a new directory and os.makedirs() to create directories with their parent directories if they don't exist.
- The os.chdir() method helps change the current working directory, while os.getcwd() retrieves the current working directory.
- Construct file and directory paths in a platform-independent way using os.path.join().
- Renaming directories can be done using os.rename(), and empty directories can be removed using os.rmdir().
- To list the contents of a directory, use os.listdir().