Disable Button in jQuery with Examples
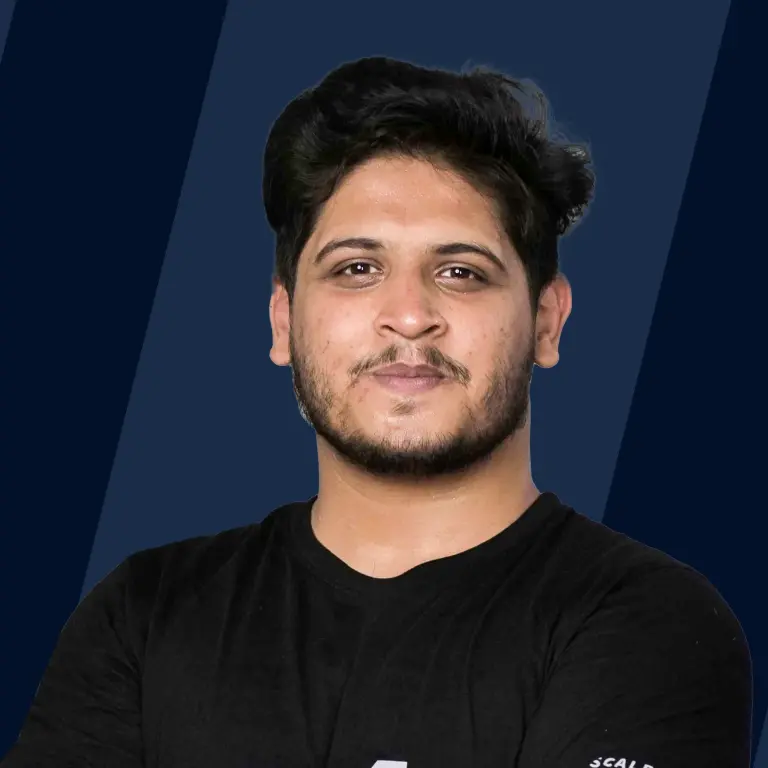
Overview
In jQuery, disabling a button is a common interaction technique used to prevent users from clicking it or submitting forms multiple times. This is typically done by selecting the button element using a jQuery selector and then using the .prop() or .attr() methods to set the disabled attribute to true.
This prevents further interactions with the button until the attribute is reset to false. Disabling buttons is often used to enhance user experience by preventing accidental double-clicks or submission of duplicate data. It's a straightforward and effective way to control user interactions and ensure the proper functioning of web applications.
Disabling Button on Click
To disable a button when it's clicked using jQuery, we can attach a click event handler to the button and then set its disabled attribute to true within that event handler.
First, make sure we have included the jQuery library in our HTML file. We can do this by adding the following script tag to our HTML document, typically in the <head> section or just before the closing </body> tag.
Next, create our HTML button that we want to disable on click.
Now, use jQuery to add a click event handler to this button and disable it when clicked.
Output:
Before:
After:
Explanantion:
In the above code: We use $(document).ready() to ensure the DOM is fully loaded before attaching the click event handler. The click event handler is attached to the button with the ID myButton Inside the event handler, $(this) refers to the clicked button, and we use .prop("disabled", true) to disable it.
Disabling Submit Button
Disabling a submit button when a form is submitted is a common practice to prevent users from submitting the form multiple times. Here's an explanation with code on how to achieve this using HTML and JavaScript.
Output:
Before:
After:
Explanation:
In this example, We have an HTML form with a username and password input fields and a submit button. When the form is submitted, the event listener is triggered. Inside the event listener, we prevent the default form submission behavior using event.preventDefault() to allow for custom handling of the submission.
We disable the submit button by setting its disabled property to true. This prevents users from clicking the button multiple times. After the simulated form submission is complete, we re-enable the submit button by setting its disabled property to false.
Disabling Button on Page Load
Disabling a button on page load can be useful in scenarios where we want to prevent users from interacting with a button until certain conditions are met or until the page is fully loaded.
Here's an explanation with code on how to disable a button on page load using JavaScript.
Output:
Explanation:
In this code, We start with a basic HTML page containing a button with the ID myButton. JavaScript code waits for the DOMContentLoaded event to ensure the button element is fully loaded. We fetch the button element using document.getElementById("myButton").
The button is initially disabled, preventing user interaction on page load. We employ setTimeout to potentially re-enable the button after a 3-second delay. Adjust this timing or add your custom conditions for enabling.
Enabling Button Using jQuery
Enabling a button using jQuery is a common interaction technique, typically used after disabling it or based on certain conditions. Below is an explanation with code on how to enable a button using jQuery.
Output:
Before:
After:
Explaination:
This code snippet begins with a basic HTML page featuring a button initially disabled with the "disabled" attribute. In the JavaScript section, it includes the jQuery library and ensures code execution after full DOM loading. A jQuery selector selects the button by its ID, and within a setTimeout function, the button is re-enabled after a 3-second delay. This is achieved using myButton.prop("disabled", false);, allowing the button to become clickable again. We can customize the enabling logic to suit specific conditions or events, providing control over user interactions in a web application.
Checking If a Button is Disabled or Enabled Using jQuery
We can use jQuery to check if a button is disabled or enabled by examining its "disabled" property.
Output:
Explanation:
This code involves an HTML page with two buttons: one initially disabled with the ID myButton, and another button, checkButton, used to inspect the first button's state.
In the script section, jQuery is included via a CDN link, ensuring it runs after DOM loading. Both buttons are selected and stored using jQuery.
An event handler is attached to checkButton to check "myButton's" state. When checkButton is clicked, it examines "myButton's" "disabled" property. This code snippet provides a way to dynamically check and respond to the state of buttons in a web page.
Conclusion
-
In jQuery, disabling a button is done by selecting the button element and setting its disabled attribute to true using methods like .prop() or .attr().
-
To prevent multiple clicks and interactions, we attach a click event handler to a button and disable it when clicked. This helps control user actions.
-
For form submissions, it's common practice to disable the submit button. This is achieved by adding a submit event listener, preventing the default form submission, disabling the button, and re-enabling it after processing to prevent multiple submissions.
-
Disabling a button on page load is useful for controlling initial user interactions. JavaScript is used for the initial disable, and you can enable it based on specific conditions or criteria, like delays or custom logic.