C++ Program to Make Division Calculator
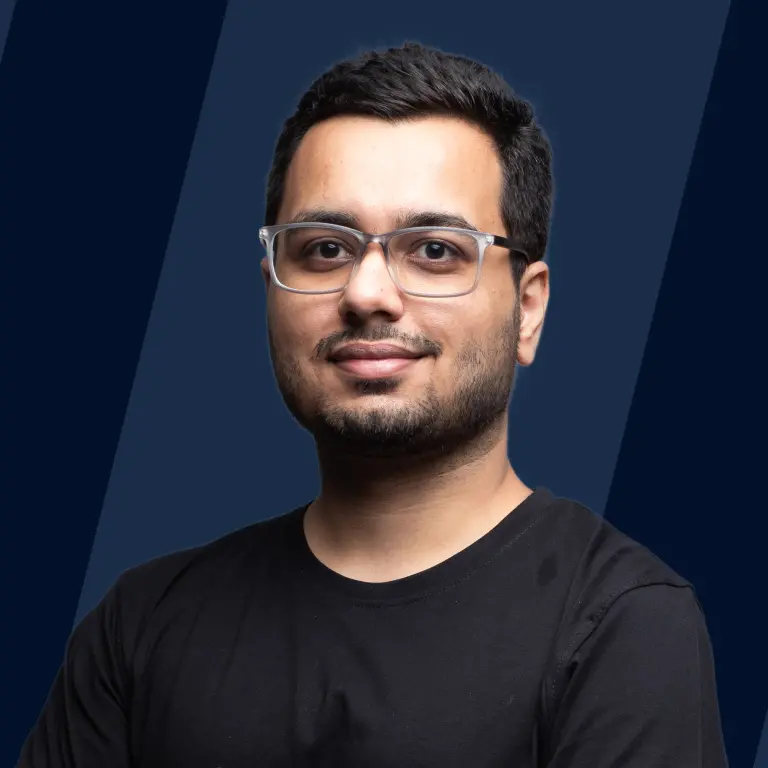
Overview
The division is a method of splitting a group of things into equal parts. Division can be referred to as an inverse of the multiplication operation. As we know, division is one of the basic and important arithmetic operations. It is necessary to know how to calculate division while programming. In C++, we can calculate division using the division operator.
Introduction to C++ Division Arithmetic Operation
Division is one of the four basic operations in mathematics: Addition, Subtraction, Multiplication, and Division.
The division is defined as the splitting of a group of objects into equal parts. It is the inverse of multiplication in mathematics. The basic equation of division is as follows:
- Dividend: A dividend is a number that is to be divided by any other number.
- Divisor: Divisor is the number by which the dividend is to be divided.
- Quotient: The resultant of the division is called the quotient.
- Remainder: The number left after dividing the dividend by the divisor is called the remainder.
In C++, the division is performed using the arithmetic operator /. In this article, we will see the division of both integers and floating-point numbers in C++.
Syntax of C++ Division Operator
The syntax of the division operator in C++ is as follows:
Here operand_1 is dividend and operand_2 is divisor. If operand_1 is divisible by operand_2, then the result displayed is exact. If operand_1 is not divisible by operand_2, then based on the datatypes of operands and results, the result would be displayed would not be exact and may have some loss.
Note:
- Case 1: Dividend is divisible by the divisor. The operation gives a quotient, and the remainder is 0.
- Case 2: Dividend is not divisible by the divisor. In practical operations, the quotient is considered a result of division. When a dividend is not completely divisible by the divisor, we get the result in fractions. In the case of programming, if the result has datatype as an integer, then the fraction is discarded. And if the result has a datatype float, then the result can be displayed with precision.
C++ Division with Two Integers
In C++, we can divide two integers using the division operator "/". Below is a code snippet showing the types of operands and return values when two integers are divided.
As shown above, both the operands and the result are integers, if the dividend is not exactly divisible by the divisor, then the operation returns the quotient as a result, and the remainder is discarded.
Let's see a division calculator program to perform the division of two integers:
Program:
Output:
Explanation: In C++, division with two integers division calculator program, we have initialized variables with datatype int. / operator is used to perform division of these variables and store their results in variables with datatype int. Finally, we have displayed the result of the operation on screen using the cout statement in C++.
C++ Division with Two Floating Point Numbers
In C++, we can divide floating point numbers using the division operator /. Let's see a code snippet that shows data types of operands and return values in the division of two float values.
In this case, both dividend and divisor are floating point numbers, and the result is stored in a floating point number. The division operator divides the dividend by divisor and returns a value with full precision.
Program for division of two floating point numbers
Let's see a division calculator code that displays the division of two floating point numbers using the division operator /.
Program:
Output:
Explanation: In C++, division with two floating point numbers division calculator program, we have initialized variables with datatype float. The / operator is used to divide these variables and store their results in variables with datatype float. Here the result of division is precise. Finally, we have displayed the result of the operation on screen using the cout statement in C++.
Program for Division of Two Floating Point Numbers – Entered by user
Now, let's see a division calculator program that divides two floating numbers by taking input from users.
Program:
Output:
Explanation: In this division calculator program, we have used I/O functions in C++, cout & cin, to take user inputs and store them in variables. Division operation is performed on these variables, and the result is displayed on the screen.
C++ Division with Integer and Floating Point Number Using Function(user defined function)
In C++, we can divide an integer by a floating-point number and vice versa. Let's see a code snippet that shows data types of operands and return values in the division of integer and floating point numbers.
Let's use a C++ division calculator program to see the division of integer and floating-point numbers.
Program:
Output:
Explanation: In the above division calculator program, we have performed the division of integers by the float and vice-versa. The result of both operations is in floating point numbers, as different data types are not completely divisible by each other.
We have defined two functions in this program, divFun1 and divFun2. These functions take inputs in specific datatypes from the user and perform division calculations. We have called these user-defined functions in the main function, and the results are displayed for the respective calculations.
Chaining of Division Operator
Chaining of the division operator is one of the important functions of the division operator in C++. We can chain the division operator / and perform a division of more than two operands in a single C++ statement. The syntax of this operation is as follows:
In the chaining of the division operator, the calculation happens from left to right. Hence, in the above pseudo-code, firstly operand1/operand2 calculation is done, and its result is divided by operand3 and so on.
Let's write a C++ division calculator program to perform the chaining of the division operator.
Program:
Output:
Explanation: In the above program, the chaining of the division operator is executed using C++. Here, int result = a / b / c / d; is used to execute the chaining of the division operator. Here the division operations are done as follows:
The final output is the result of the above operations.
Conclusion
- Division is one of the four basic arithmetic operations. In C++, division calculation is possible using the division operator denoted by /.
- We can divide integers and floating point numbers using the / operator. The result's precision depends on the divisibility and datatypes of operands and results.
- Chaining of the division operator is possible in C++, allowing the division of multiple operands in a single statement.