Java Double compare()
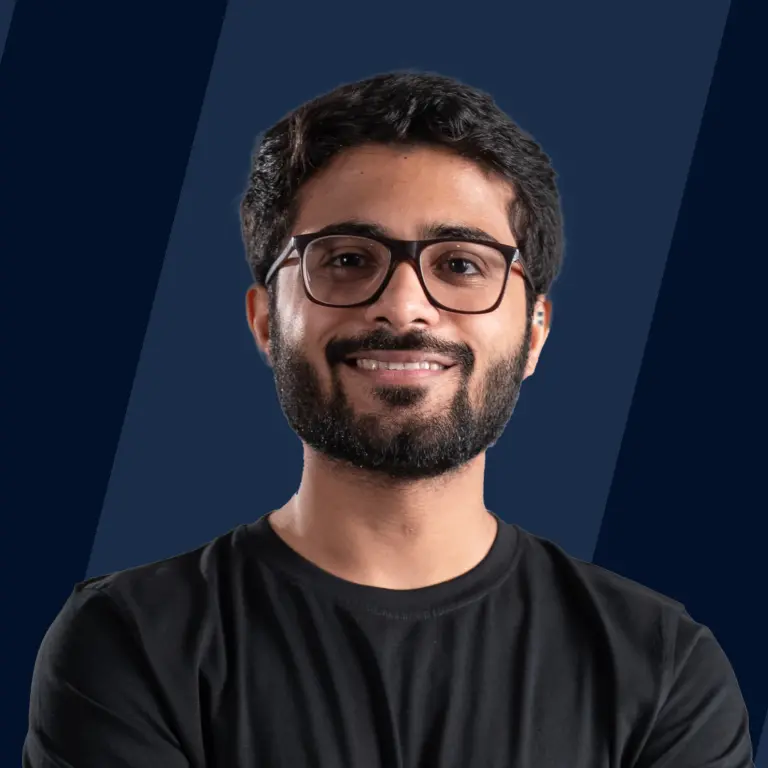
Overview
The compare() method in Java Double class is a static method used to compare two double values. It returns an int value that indicates the relative ordering of the two double values.
Syntax of Java Double Compare()
Let us look at the syntax of the Double compare() method in Java.
Syntax :
Explanation :
In the above syntax, compare() method is called, which is a static method and consists of two parameters of double type. This method returns an integer value explained below.
Parameters of Java Double compare()
The Double compare() method in Java accepts two parameters :
- double d1 : The first double value to be compared.
- double d2 : The second double value to be compared.
Return value of Java Double compare()
The Double compare() method returns an int value. Different values in different cases, let us look at them :
- Zero (0) : The Double compare() method will return 0, if both the parameter values d1 and d2 are numerically equal to each other.
- Positive value: The Double compare() method will return a positive value, if the parameter value d1 is numerically greater than the parameter value d2.
- Negative value: The Double compare() method will return a Negative value, if the parameter value d1 is numerically less than the parameter value d2.
Exceptions of Java Double compare()
The Double compare() method does not have any exceptions in particular.
Example
Let us now look at a small example of the Double compare() method in Java code, to make it more clearly understanding.
Abstract :
In this code, we will see how the Double compare() method is used to compare two values of double type in Java programming language.
Code :
Output :
Explanation :
Let us understand the above code :
-
In the above code, firstly, we are creating two instance variables of Double type, and they are private in nature, that is, they cannot be accessed outside the class, and we have done this just for the sake of privacy.
-
Inside the main() method, firstly we are defining the instance variables, and creating the object of those variables, of Double type with some values in them.
-
Then we are performing the central part of this code, that is, calling the compare() method, to compare the two Double values and storing the result in a compare variable of integer type. As we know the compare() method will return the result of integer type, so we are storing the result in an integer variable.
-
Then depending on the result we are checking if both the values are equal, or which one is greater or lesser with three conditions :
-
If the result value is zero (compare = 0), that means both the Double type values are equal and we are also printing the difference between the two values, and in this case, it will be 0.
-
If the result value is greater than 0 or positive (compare > 0), that means the first Double type value is greater than the second one, and we are also printing the difference between the two values, and in this case, it will be greater than 0.
-
If the result value is less than 0 or negative (compare < 0), that means the first Double type value is less than the second one, and we are also printing the difference between the two values, and in this case, it will be less than 0.
What is Java Double compare()?
- In Java, comparing two Double type values is not as straightforward as using the == operator for comparing two primitive data type variables.
- This is because double values can have rounding inaccuracies due to their representation in the computer's limited memory space of usually 64 bits.
- Using the == operator for double type values can lead to difficult to trace bugs at runtime.
- Instead, Java provides the compare() method for accurately comparing two Double type values.
The compare() method is a built-in method that belongs to the Double class in Java. The main purpose of the compare() method is to compare the two specified double values and to check whether both the values are equal or which value is greater or lesser.
More Examples
Now let us look at some more examples of the Double.compare() method covering different scenarios for more clear understanding.
Example 1 : When Both the Values are the Same
Abstract :
In this code, we will see how the Double compare() method is used to compare two same values of double type and what it returns in Java programming language.
Code :
Output :
Explanation :
Let us understand the above code :
- Inside the main() method, firstly we are creating two double type variables and storing values in them for comparison.
- Then we are performing the compare operation between d1 and d2.
- In the above code, as we can see both the values d1 and d2 are the same, so the compare() method is returning a 0 , hence the output is Both d1 =10.1 and d2 = 10.1 are equal!.
Example 2 : When the First Value is Greater than the Second Value
In this example, we will see the output of the compare() method when the first value d1 is greater than the second value d2.
Code :
Output :
Explanation :
- In the above code, as we can see both the values d1 and d2 are different and the second value d2 is less than the first value d1, so the compare() method is returning a positive value.
- Hence the output is d1 = 11.1 is greater than d2 = 10.1.
Example 3 : When the First Value is Less Than the Second Value
In this example, we will see the output of the compare() method when the first value d1 is less than the second value d2.
Code :
Output :
Explanation :
Let us understand the above code :
- Inside the main() method, firstly we are creating two double type variables and storing values in them for comparison.
- As we know the compare() method will return the result of integer type, so we are storing the result in an integer variable.
- In the above code, as we can see both the values are different and the first value d1 is less than the second value d2, so the compare() method is returning a negative value , hence the output is d2 = 11.1 is greater than d1 = 10.1.
Conclusion
- The compare() method is a built-in method that belongs to the Double class in Java.
- The main purpose of the compare() method is to compare the two specified double values and to check whether both the values are equal or which value is greater or lesser.
- Syntax of compare() method is :
- The Double.compare() method in Java accepts two parameters of Double type.
- The Double.compare() method returns an integer type of value.
- The Double compare() method will return 0, if both the parameter values d1 and d2 are numerically equal to each other.
- The Double compare() method will return a positive value, if the parameter value d1 is numerically greater than the parameter value d2.
- The Double compare() method will return a Negative value, if the parameter value d1 is numerically less than the parameter value d2.