What is Double in C?
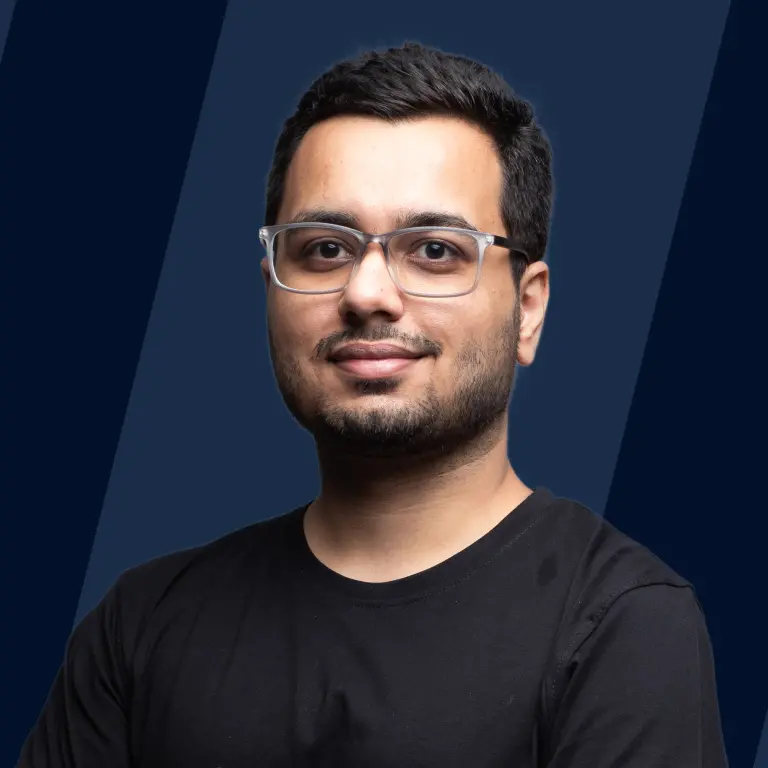
In programming, we often encounter large decimal values in terms of precision (e.g. ). In C language, such numbers cannot be stored in the pre-defined data type for decimals (i.e. float). Thus, a new data type is introduced to store such large decimal values.
The double in C is a data type used to store high-precision floating-point data or numbers (up to 15 to 17 digits). It is used to store large values of decimal numbers.
Values that are stored are double the size of data that can be stored in the float data type. Thus it is named a double data type.
Any variable declared using the double data type in C is of the size 8 bytes (or 64 bits).
Note:
-
In double data type, the 1 bit is for sign representation, 11 bits are for the exponent, and the remaining 52 bits are used for the mantissa.
-
The double in c ranges between 1.7E-308 - 1.7E+308. Double can represent the data of real numbers, decimals, negative values , etc.
Syntax for Declaration
The variables of the double in c are declared using the double keyword followed by the variable's name.
Initialization of Double Variable
When we declare a variable, it mostly contains a garbage value. Thus for our variable to have a certain value, we need to initialize the double variable with that certain value.
There are two ways to initialize a double in C :
-
By assigning the declared double variable a value using the assignment operator :
note: It can store up to 15-17 digits.
-
By assigning the double variable a value during the declaration using the assignment operator :
Example of Double in C
In this section, we will discuss a few examples of double in C.
In the above example, temp1 is declared and assigned the value 98.4. temp2 is declared but not assigned any value; thus, it will contain a garbage value. temp3 is declared; thus, it will have a garbage value, but later, it is assigned the value 97.88.
How to Print the Double Value in C?
Now that we've learned how to declare and initialize a double in C, how do we display a double in C?
A double in c can be printed using both %f and %lf. Both the %f format specifier and the %lf format specifier represent float and double. The printf() in c treats both float and double the same.
Output :
Note :
In the case of a general specifier (e.g. int) the value will be type cast and the integer value will be printed. This is because these general specifiers already assume that the value is of integer type.
Representation of Double in C
The precision of the double in c depends upon its implementation in the code. In general, modern compilers use the IEEE-754 standard.
The IEEE Standard for Floating-Point Arithmetic (IEEE 754) is a technical standard for floating-point computation which was established in 1985 by the Institute of Electrical and Electronics Engineers (IEEE). The standard addressed many problems found in the diverse floating point implementations that made them difficult to use reliably and reduced their portability.
In such compilers, the numbers are represented using 64-bits in the following manner :
- The first bit stands for the sign. The positive is represented by 0 and the negative is represented by 1.
- 52 bits of significand (mantissa).
- 11 bits of exponent.
It can store the value up to 15-16 digits.
Following is the pictorial description of the representation of double in C :
Some Programs of Double Date Type in C
In this section, we will see a few programs that involve the use of double in C.
Program to Get the Size of Data Types Using sizeof() Function
The sizeof() function can be used to determine the size of different data types in c. This function will take the data type as a parameter and return the size in bytes.
Code :
Output :
Explanation of the example :
In the above example, we are passing the data types int, char, float, and double to the sizeof function. The printf is used to display their output.
Program to Convert Feet into Meters Using the Double Data Type
We can convert the measuring units using double in C. This can be done by taking the input in feet and dividing the value by 3.28. Since the result can be a large decimal value, it will be stored in the double data type.
Code :
Output :
Explanation of the example :
In the above code, we have defined the function 'functionToMeter' that takes a double value as a parameter. The feetInput / 3.28 converts the feet value into a meter and returns the value.
Program to Convert an Integer Data into Double Data Type
In this section, we will learn how to convert the data type in c.
Code :
Output :
In the above example, we have a numerator and denominator of integer type. We have created the remainder variable of the double type. The will output an integer value as integer / integer will return an integer.
Thus we will convert the data type of numerator into a double with the (double) numerator. Now the will return a double value which can be stored in the remainder.
Program to Convert Celsius to Fahrenheit Temperature
Output :
Float vs Double Data Type in C
Following are a few differences between float and double in C :
Float | Double |
---|---|
The float data type holds a 32-bit floating-point value. | The double data type holds a 64-bit floating-point value. |
The float type equals 4 bytes. | The double type equals 8 bytes. |
The float data type is relatively faster in terms of operation speed because when you don't need double precision and you are memory-bandwidth bound and your hardware doesn't carry a penalty on floats. They conserve memory bandwidth because they occupy half the space per number. | The double data type is slow as compared to float. |
The float data type can hold approximately 7 digits | The double data type can hold approximately 15-17 digits |
The range of float is in between to | The range of double is in between to |
Learn More
Visit this link to learn more about double in c.
Conclusion
- The double in C stores high-precision floating-point data or numbers.
- The double in C ranges between to .
- The double in C can hold up to 15-17 digits.
- The exact precision of double depends on the code implementation.