Program to Convert Double to Int in Java
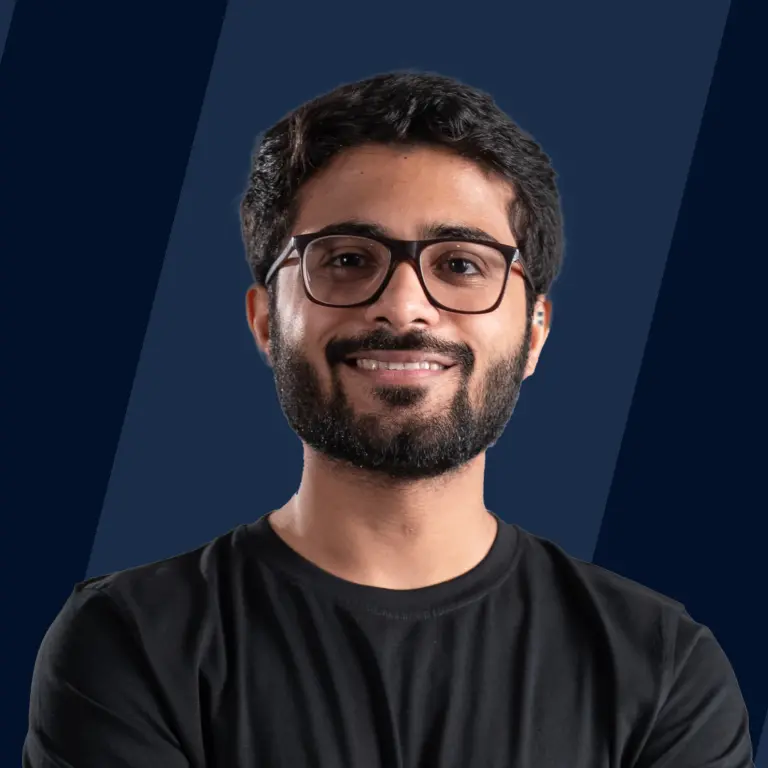
Overview
- The primitive data types include double to int in Java. The primitive data type int is used to represent integer values such as , etc., whereas double is used to represent floating-point integers such as , , etc.
- In some cases, input data to Java programs are available in Java double, but it is necessary to round it off to its closest integral value.
- In such cases, the double value must be transformed to an int data type. For example, to print average weight, height, etc., or to generate a bill, it is preferable to represent the value as an integer rather than a number with floating-point precision.
How to Convert Double to Int in Java?
The double type is used to store floating-point values, and the integer type is used to store non-decimal values (integer) values. A Double primitive has decimal digits. When these numbers are converted to integers, the decimal digits are reduced by rounding off the number to the nearest integer using the technique you select.
The following are the primary concerns when converting a double to an int in Java:
- Range: The range of a double is substantially larger than that of an int. What should happen if you have a double value that will be out of range if converted to an int?
- Rounding: How a double with a decimal value is rounded when converted to an integer.
Java provides three methods for converting Double data to integer numbers:
- TypeCasting: Simple and user-friendly. It is used when you want to remove the numbers preceding after the decimal point.
- Math.round(): This technique rounds a Double value to the nearest integer.
- Double.intValue(): It is used when you have a Double-object.
Method 1: Using TypeCasting
- Typecasting is used in Java to transform variable values from one type to another. Typecasting is a method or technique for manually and automatically converting one data type to another. The compiler performs an automatic conversion, while the programmer performs a manual conversion.
- If you want to convert a double to the nearest integer, such as to , you can't use typecasting method because it will give , which is not the nearest integer.
- Java restricts the number of digits you can save for any double number to about 14-15 digits. You should be aware that the precision of any computer calculation is limited by the fact that computers can only store a limited number of digits.
Syntax:
Code:
Output of the above code:
Method 2: Using Double.intValue() method
- It is an instance method of Double class. This method converts the Double-object value to the primitive data type int and returns the int value.
Syntax:
Code:
Output of the above code:
Method 3: Using Math.round() method
The java.lang.Math.round() is a built-in math function that returns the closest value to the argument. The result is rounded to an integer by adding 0.5, taking the floor of the result after adding 0.5 and casting the result to type long. Suppose you want to round off to , i.e., the nearest integer, then you should use this method because both the above-discussed methods will convert it to , which is not the nearest integer value.
- If the input is NaN, the Output will be 0.
- If the input is negative infinity or any value less than or equal to Integer.MIN VALUE. The Output is equal to the value of the Integer.MIN VALUE.
- If the input is positive infinityor any value greater than or equal to Integer.MAX VALUE. The Output is equal to the value of the Integer.MAX VALUE.
Note: Double to int is only possible if the value is not greater than Interger.MAX VALUE and not smaller than Integer.MIN VALUE.
Syntax:
Code:
Output of the above code:
Conclusion
- The simplest approach to converting a double to an int in Java is downcasting it. This will return the non-fractional half of the integer as an int. However, it does not round to the nearest integer. Thus, even will be transformed to .
- If you want to convert a double to the nearest integer, such as to , you should use the Math.random() method. It adds to the double value and then truncates the decimal value to round it to the nearest integer. However, this technique returns a long, which must be further downcast into an int.
- Another option is to use the Double.intValue() function, which is best if you already have a Double object. Still, you can also use auto-boxing to convert a double primitive to a Double object and then call this method.