How to Validate Email Id Using jQuery?
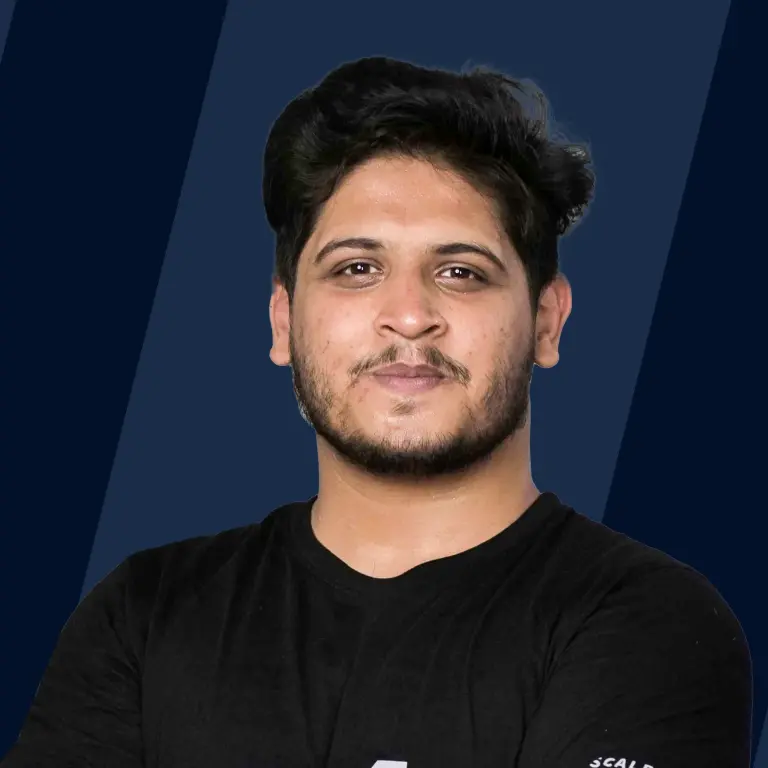
Overview
Validating email IDs using jQuery is essential for ensuring data accuracy in web forms. Begin by incorporating jQuery into your project. Develop an HTML form with an email input field. Use jQuery to trigger validation either on form submission or when the input loses focus. Employ regular expressions to verify if the entered email adheres to the correct format (e.g., /^[\w-]+(\.[\w-]+)*@([\w-]+\.)+[a-zA-Z]{2,7}$/). Provide user feedback through error messages or visual cues and prevent form submission for invalid emails. This process enhances user experience and ensures that only valid email addresses are accepted, improving data quality on your website.
Email Validation Using Regex Pattern in jQuery
Email validation using a regular expression (regex) pattern in jQuery involves using a regular expression to check if an email address entered by a user in a form or input field is valid. jQuery is a JavaScript library that simplifies working with HTML elements and handling user interactions, making it a popular choice for form validation tasks. Here, I'll explain how to perform email validation using a regex pattern in jQuery step by step.
- Include jQuery
First, make sure you have included the jQuery library in your HTML document. You can either download it and host it on your server or use a CDN (Content Delivery Network) link to include it. Here's an example of including jQuery using a CDN:
- HTML Form
Create an HTML form with an input field for the email address and a button to submit the form.
This form includes an input field where users can enter their email addresses and a submit button.
-
jQuery Code Write jQuery code to handle form submission and perform email validation using a regex pattern. In this example, we'll use a basic regex pattern for email validation:
In this code, we define a regular expression pattern (emailPattern) for email validation. The isValidEmail function checks if the provided email matches this pattern using the test method. When the form is submitted, the jQuery code prevents the default form submission behavior (event.preventDefault()), gets the email entered by the user, and checks if it's valid. If it's valid, it displays a success message; otherwise, it shows an error message.
-
Styling and Enhancements You can enhance the user experience by adding CSS styles and additional validation checks as needed, such as checking if the email address is required.
Full Working Code
Output:
Email Validation - Robust Method
Robust email validation is crucial for ensuring that user input adheres to expected email address formats. While the provided code offers a basic email validation approach, a more robust method considers the following aspects:
- Pattern Validation: Use a regex pattern that conforms to the official email format standard (RFC 5322). This pattern should be thorough and cover all valid email address variations.
- Domain Verification: Check if the domain exists and has valid DNS records. You can use DNS lookup techniques to validate the domain's existence.
- MX Record Check: Ensure that the domain has valid Mail Exchange (MX) records, indicating it can receive emails.
- Syntax and Length: Validate that the email adheres to the correct syntax and doesn't exceed the maximum length (usually 320 characters).
- Disposable Email Detection: Detect and reject disposable email addresses to prevent spam.
- Real-time Validation: Implement real-time validation, which provides immediate feedback to users as they type, rather than waiting until they submit the form.
- Internationalization: Support internationalized email addresses with non-ASCII characters, if applicable.
- Feedback: Provide clear and helpful error messages to guide users when their input is invalid. Explain why their input is not accepted.
- Security: Protect against email injection attacks by properly sanitizing and escaping user input.
A more robust email validation method combines regex patterns with additional checks to ensure that the provided email address is not only syntactically correct but also functionally valid for sending and receiving emails.
Remember that while client-side validation is useful for improving user experience, server-side validation is essential for security and should always be implemented to prevent malicious input.
Email Validation Using jQuery Validate Plugin
Validating email addresses using the jQuery Validate plugin is a straightforward process. The jQuery Validate plugin is commonly used to perform client-side form validation, including email validation. Here's a step-by-step guide on how to set up email validation using this plugin:
-
Include jQuery and the jQuery Validate plugin in your HTML Make sure to include jQuery and the jQuery Validate plugin in your HTML document. You can include them from content delivery networks (CDNs) or download them and host them locally. Place these script tags in the <head> or before the closing </body> tag:
-
Create an HTML form Create an HTML form with an email input field that you want to validate:
-
Initialize the jQuery Validate plugin In a <script> tag after including jQuery and the jQuery Validate plugin, initialize the plugin and define the validation rules. In this case, we'll use the email rule to validate the email field:
In the above code:
- $("#myForm") selects the form element with the ID "myForm."
- validate() initializes the validation plugin for the form.
- rules specify the validation rules for each form field.
- required: true makes the email field required.
- email: true validates the email format using the built-in email rule.
-
Add error messages The messages option in the configuration defines custom error messages for the email field. You can customize these messages to fit your needs.
-
Handling form submission You can prevent the form from submitting if there are validation errors by adding a submit handler:
In this example, the submitHandler function is called when the form is valid, allowing you to perform further actions like submitting the form using AJAX.
Email Validation Using HTML5 Attribute
HTML5 introduced several new input types and attributes, including the email input type, which allows you to specify that an input field should accept email addresses. However, HTML5 alone does not provide built-in email validation. To add email validation using the HTML5 email input type along with jQuery, you can use the following approach:
- Create an HTML form with an input field of type email and a submit button:
- Create a JavaScript file (e.g., script.js) to add validation using jQuery:
Explanation:
- We use jQuery to select the form with the ID emailForm and add a submit event handler to it.
- Inside the event handler, we prevent the default form submission using event.preventDefault().
- We get the value of the email input field using $("#email").val().
- We use a regular expression (emailPattern) to validate the email format. You can adjust this regular expression to suit your specific email validation requirements.
- If the email is valid, we display a success message in the <p> element with the ID message in green text. If the email is invalid, we display an error message in red text.
This code will perform basic email validation using the HTML5 email input type and jQuery. Keep in mind that client-side validation is useful for improving user experience, but server-side validation is essential for security and should always be implemented as well.
Conclusion
- Validating email IDs using jQuery improves the user experience by providing real-time feedback to users as they fill out forms.
- jQuery email validation can prevent unnecessary server requests for invalid email addresses, reducing server load and improving website performance.
- Users receive immediate feedback about the validity of their email address, reducing the likelihood of form submission errors.
- jQuery allows for customization of error messages, enabling developers to create user-friendly prompts for correcting email input.
- Email validation with jQuery is a client-side process, minimizing the need for round trips to the server and speeding up form submission.
- jQuery is widely supported across different browsers, ensuring consistent email validation across various user devices.
- Developers can tailor the email validation process to suit specific project requirements, such as enforcing unique email addresses or allowing for disposable email providers.
- By filtering out invalid or potentially malicious email addresses on the client side, jQuery validation can contribute to improved security by reducing the risk of spam or abuse.
Incorporating jQuery for email validation is a valuable technique for web developers looking to enhance their websites' usability, performance, and security.