HashMap entrySet() Method in Java
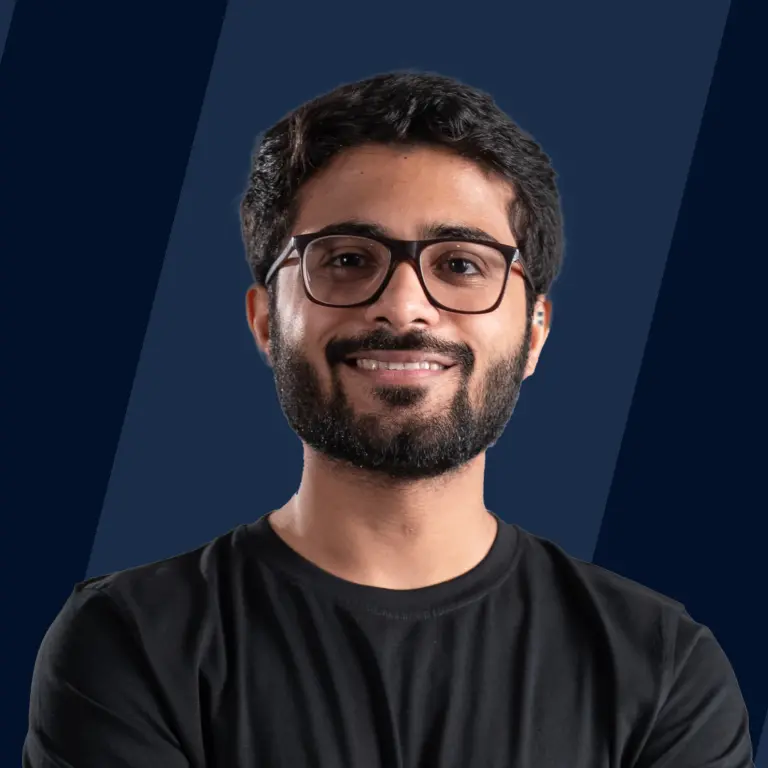
The java.util.HashMap class has an entrySet() method. HashMaps are used to store key-value pairs in Java, where all keys must be unique. The method returns a Set of maps.Entry objects represent a key-value pair contained in the HashMap. It enables direct access to both keys and values within the map. It's often used for iterating, sorting, filtering, and performing operations on key-value pairs.
Syntax of Java HashMap entrySet()
The syntax of the HashMap is as follows:
Initially, we created the HashMap. We can add values to this HashMap and use the entrySet() method to retrieve the entries in the Map.
Parameters of Java HashMap entrySet()
It doesn't take any arguments.
Return Value of Java HashMap entrySet()
The entrySet() method of the Map interface returns a set view of the key-value pairs contained in the map. Each element in the set is a Map.Entry object, representing a single key-value pair.
Examples of Java HashMap entrySet()
Let us look at examples of HashMap entrySet().
Basic Example of Java HashMap entrySet()
Let us take a very simple example of a class. We are using a map to store the scores of the students in a class. We will store the entries in a set using the entrySet() method that generates a Set of Map.Entry object. This set is further converted to the list to filter out students who score fewer marks than required to pass the examinations.
We are storing all the entries in the HashMap into a set, which is later converted to a list with the help of the copyOf() method. It simply takes each item from the set and copies it to the List. Next, we are using a simple for loop and traversing through each entry to print the names and scores of the students that crossed the threshold.
entrySet() Method in for-each Loop
Instead of all the hurdles in the previous method of converting into a Set, then into a List, and finally using a for loop, Java provides us with the for each loop. We can skip the first two steps and directly jump to each loop to perform the above task.
Let us look at the same example using the for each loop:
The above code looks cleaner and is easier to understand. The for each loop helps to perform the same. It visits each entry consisting of a key and a value. This is then separated using the getKey() and getValue() methods.
Mapping String Values to Integer Keys
Let us look at an example of HashMap, whose keys are of Integer data type and whose values are of string data. We use the ID as an int type and department names as a String. This data is stored in HashMap. Later, while printing user data, we try to retrieve the department name with the help of the employee's integer key.
Mapping Integer Values to String Keys
Another case could be using string values as keys and integers as values. Sometimes, when the ID is composed of characters and digits, we can use the String data type to store them.
In the example above, we are taking products and their sales. The names are keys, and their sales are values. Lastly, we print the map using each loop and entrySet().
Difference between KeySet() and entrySet()
Feature | keySet() | entrySet() |
---|---|---|
What it returns | Set of keys | Set of Map.Entry<K, V> (key-value pairs) |
Type of elements | Elements are keys | Elements are key-value pairs |
Iteration efficiency | Efficient for key-based operations | Efficient for key-value-based operations |
Access to values | Requires additional map lookup | Values are readily available in Map.Entry |
Memory consumption | Less memory as only keys are stored | Slightly more memory due to key-value pairs |
Use cases | When you only need keys | When you need both keys and values |
Example | Set<K> keys = map.keySet(); | Set<Map.Entry<K, V>> entries = map.entrySet(); |
Memory Consumption in keySet() and entrySet():
The keySet() method consumes less memory than the entrySet() method. This is because the keySet() method only returns a set of keys, whereas the entrySet() method returns both the keys and values.
- Another overhead is returning the objects of Map.Entry using the entrySet() method, which consumes more memory than an individual key.
Conclusion
- HashMap data structure in Java stores key-value pairs where each key must be unique. The java.util.HashMap class contains a method entrySet() that returns a set of entries. These entries are objects of Map.Entry, and each entry consists of a key and value.
- To get the key and value from the entry, we use the entry.getKey() and entry.getValue() methods, respectively. The major difference between the methods map.keySet() and map.entrySet() is that the latter returns both keys and values, while keySet() only returns a set of keys in the hashmap.