What is C++ Enum?
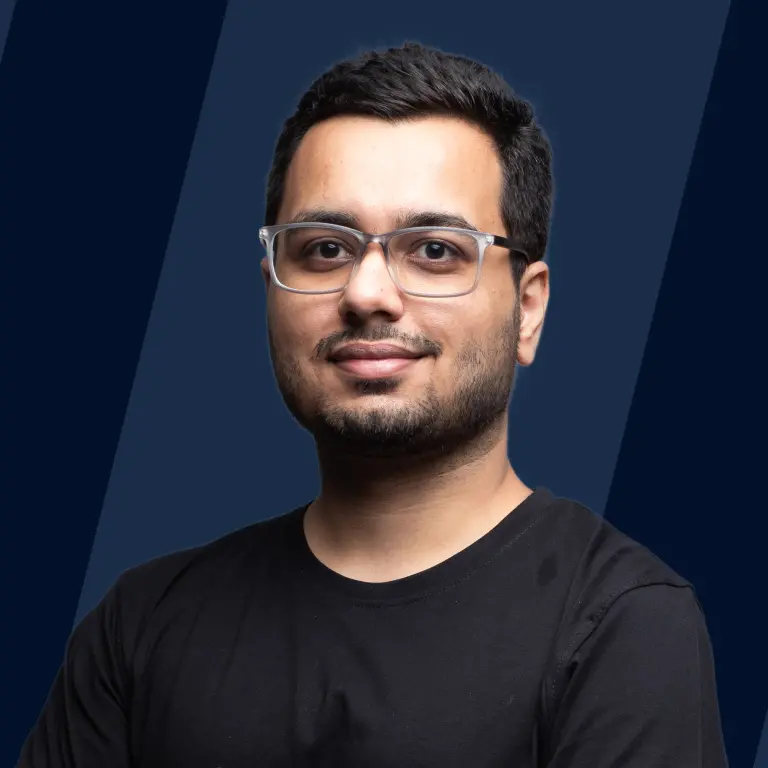
Introduction
Enum in C/C++ language also known as Enumeration is a user-defined datatype that is used to assign names to the integral constants. The enum keyword is used to declare an enumeration in C/C++. The enum is purposely used because it makes a program easy to read and maintain.
Syntax of C++ Enum
Below given is the syntax of C++ enum.
Declaration of C++ Enum
The syntax of the enum is shown above and here is the declaration of the enum.
enumName = It is the name given by the programmer
constant1, constant2 , ... = These are the values of the constant variables. By default, the values are 0 for constant1, 1 for constant2, 2 for constant 3, and so on...
The enum keyword, as shown below is used to define the variables of the enum data type.
Important Points to Remember Regarding Enums
- Enums can be traversed using loops.
- Enums can be easily used in the switch-case statement.
- Enums cannot extend any class because they internally extend Enum class although they may implement many interfaces.
- Enums can have methods, fields, and constructors.
- Enums are used improves type safety.
Why Enums are Used in C++?
The enums in C++ are the variables that take only one particular out of many possible values in the enum data type. Working with enums provides us efficiency along with flexibility because it does not waste memory.
Enum Conversions
C++ Int to Enum Conversion
In the above code, we can see the conversion of int data type to enum data type.
- d1 will give the error because the proper syntax is not followed.
- d2 will be assigned as TUESDAY, because the value of TUESDAY was 2 inside the enum structure.
- d3 will be assigned with 3, as 3 is not available in the enum structure, so d3 is explicitly assigned as 3.
- d9 will be assigned with an unspecified value because 9 exceeds the values inside the enum structure, but the syntax is correct.
C++ Enum to Int Conversion
In the above code, we can see the conversion of enum data type to int data type.
- d1 will give the error because the proper syntax is not followed.
- d2 will be assigned as 2, because the value of TUESDAY was 2 inside the enum structure.
C++ Enum to String Conversion
There are three methods in which we can convert enum to string
Approach 1: Using stringify() Method to Convert Enum to String in C++
In this method, we will use the stringify() method. The stringify() method in C++ is used to convert an enum into a string. Only the text written inside the parenthesis is converted using the stringify() method.
Output:
Explaination:
In the above code, we have used the stringify() method to convert enum to string and it works as follows
- In the first step we have created an enum of Week, and we have initialized the value of Monday with 0
- The convert_enum_to_string function uses the stringify() method, which internally converts the enum (which is passed as an argument) to string.
- Finally, we are printing all the enums after they are converted to a string.
Approach 2: Using the Constant Char Array to Convert Enum to String in C++
The const char* array is one of the easiest methods for converting an enum to a string. In this method, we will utilize the default values of the enum to access the elements of the string array.
Output:
Explaination:
In the above code, we have used the constant char* array to convert enum to string and it works as follows
- In the first step we have created an enum of days, and we have not initialized any variable with any value, so by default, the value taken by Monday will be 0, Tuesday will be 1, and so on...
- The enum_to_string function takes the enum name as an argument and converts it to a string by matching the enum number with the string number.
- Finally, we are printing all the enums after they are converted to a string.
Approach 3: Using a Defined Function to Convert Enum to String in C++
In this method, we will implement our function that accepts an integer of enum class as an argument and converts it into a string by returning a string as an output.
Output:
Explaination:
In the above code, we have used a defined function to convert enum to string and it works as follows
- In the first step we have created an enum of days, and we have not initialized any variable with any value, so by default, the value taken by Monday will be 0, Tuesday will be 1, and so on...
- The enumStrings function takes the enum name as an argument and converts it to a string by matching the enum number with the string number.
- The enum_to_string function is a helper function that calls the enumString function and converts the enum to string.
- Finally, we are printing all the enums after they are converted to a string.
C++ String to Enum Conversion
Below is the code for converting a string to an enum.
Output:
Input | Output |
---|---|
MONDAY | MONDAY |
MONDAY | MONDAY |
WEDNESDAY | WEDNESDAY |
THURSDAY | THURSDAY |
FRIDAY | FRIDAY |
SATURDAY | SATURDAY |
SUNDAY | SUNDAY |
Explaination:
In the above code, we have used the switch-case statement to convert string to enum and it works as follows
- In the first step we have created an enum of days, and we have not initialized any variable with any value, so by default, the value taken by MONDAY will be 0, TUESDAY will be 1, and so on...
- The convert_to_enum function takes a string as an argument and converts it to enum by checking the conditions in the if else statement.
- Finally, we have used the switch-case statement inside our main() function to print the value of the strings.
Using C++ Enum with Flags
We can use Enums with flags, by getting the value from the operation of existing values. Below is an example showing how we can get the values.
Output:
Explaination:
As we know that the binary representation of 1 is 00000001 and the binary representation of 4 is 00000100 (representing both in 8 bits). So the xor of both the numbers will be 00000101 which is 5 in decimal representation.
Examples of Enums in C++
Example 1: Enumeration Type
This is the simplest example of enums where we are using the default values taken by the enum variables starting from 0.
Output:
Explaination:
As we know, by default the value of the data inside the enum starts with 0. So if we are printing the month number of September, we have to add 1 to the this_month variable, to make it correct.
Example 2: Changing Default Value of Enums
In this example, we will manipulate some values of the enum variables to see the working of enums.
Output:
Explaination:
In the above example, we have set the values of all the days except Thursday and Friday. So in this case the value of Thursday will become one more than the value of Wednesday, and the value of Friday will become one more than the value of Thursday, so that is why the value of Friday is printed as 3. And as we have already mentioned the value of Tuesday is 34, it is printed as it is.
Frequently Asked Question (FAQ)
Q. Can We Convert Enum to Float?
A. Yes, we can convert enum to float in the same way as we have converted enum to int.
Related Articles:
Conclusion
In this quick tutorial, we have learned about the Enum in C++, and we can extract the following conclusions from the article
- Syntax and declaration of Enum in C++.
- Important points to remember regarding Enums.
- Enum conversions
- C++ Enum to int and Vice-versa
- C++ Enum to string and Vice-versa
- How to use enums with flags?