Java Enum
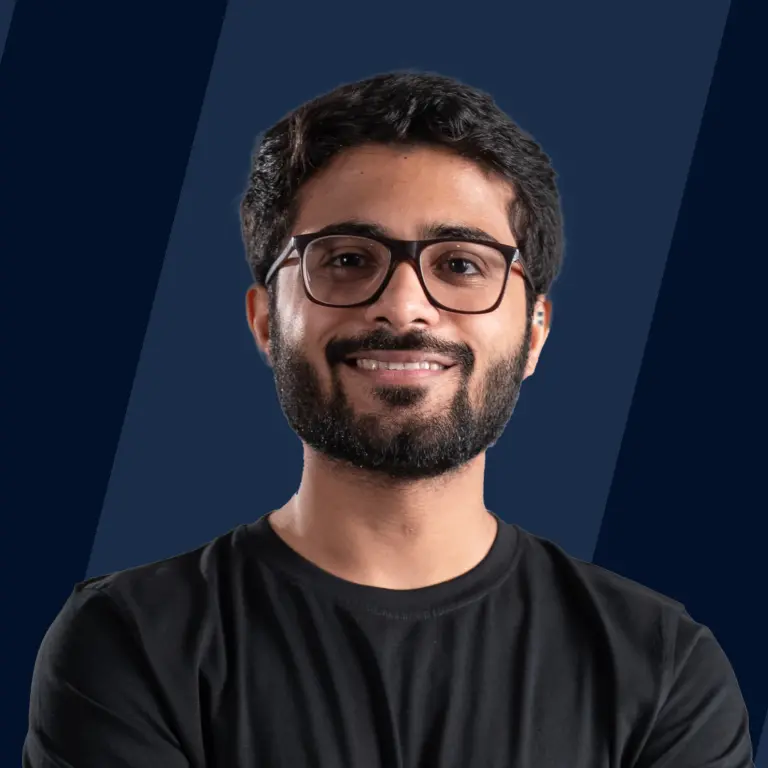
Overview
Enum or Enumeration is a special type of data that consists of a set of pre-defined constants separated by commas. Java enum constants are final and static implicitly. Java enum internally inherits the Enum class and therefore, it cannot inherit other classes but can implement multiple interfaces. We can also have methods, constructors, etc inside enum classes.
Declaration of Enum in Java
Enums can be declared inside or outside a class.
Note: Enums cannot be declared inside a method.
Declaring Enum Outside the Class
Take a look at the example given below, enum class is declared outside our HelloWorld class. This creates a top-level enum and can be used by other classes of the same package.
Declaring Enum Class Inside the Class
Let us now see how we can declare an enum inside a class. In this case, the scope of the enum is limited to that class and is used when the enum is closely related to the class and not intended to be used outside of it.
Depending on the use case, we can declare the enum inside or outside the class. The enum declared outside the class has increased scope compared to the enum declared inside a class.
Enum Inside a Class
Let us take an example of a Season class. Inside the Season class we will have an enum class Month that stores the Months and their respective Seasons. This enum class has a constructor to store the season for each month object and the getSeason() method returns the season in the form of a String.
Code:
Output:
Enum in a Switch Statement
Enums provide a concise way to represent distinct values, making switch statements more readable and avoiding the use of magic numbers or strings.
Let us take an example of traffic signals. Inside the class TrafficLight we have a Signal class that stores various signals, RED, GREEN, BLUE.
The TrafficLight class has a member variable currentSignal of type Signal. The changeSignal method is used to simulate changing the traffic light signal from RED to GREEN to YELLOW and back to RED in a cyclic manner.
Code:
Output:
Loop Through an Enum
The simplest operation in the Enum class is looping through all the objects of the Enum class. This can be done in two ways, either we can use the normal for loop or we can use the for each loop.
In the example, the Planet enum represents the planets in our solar system. The values() method is used to obtain an array of enum constants, and then you can loop through the array using either a for-each loop or a traditional for loop.
Code:
Output:
Main Function Inside Enum
In Java, the main method is usually associated with the class and not directly with the enum class. Enums are typically used to represent the set of predefined constants and encapsulate their behavior.
However, we can still declare a main class within an enum as we do in other classes.
In the example, the Operation enum represents basic mathematical operations (ADD, SUBTRACT, MULTIPLY, DIVIDE). Each enum constant has an associated apply() method, which performs the corresponding operation on two integer values.
Code:
Output:
Properties of Enum in Java
- Internally every enum in Java is implemented as a class.
- Every constant in the enum class is an object of enum type.
- Enums can be passed to an argument to switch statements.
- Enum objects are by default static and final. As they are static, can be accessed by their enum Name. Since they are final, their children cannot be created.
- The main() method can be created inside an enum class. Therefore, enums can be invoked directly.
Difference between Enums and Classes
Feature | Classes | Enums |
---|---|---|
Purpose and Usage | Used for modeling objects with attributes and behaviors. | Used for defining a fixed set of constants representing distinct, named values. |
Instances | Multiple instances can be created, each with its state and behavior. | Enum instances are constants, predefined, and immutable of the enum type. |
Extensibility | Supports inheritance and can be extended by creating subclasses. | Enums are usually closed; the set of enum constants is fixed at compile-time. These can implement multiple interfaces. |
Constructor | Can have constructors with various access modifiers. | Enum constructors are restricted (default is private) and implicitly called for each enum constant. |
Instantiation | Instantiated using the new keyword. | Enum constants are created implicitly, and the new keyword cannot be used. |
Example | java public class MyClass { /* ... */ } | java enum Day { SUNDAY, MONDAY, TUESDAY } |
These are some of the fundamental differences between classes and enums in Java. Enums are especially useful when dealing with a fixed set of values or when you want to create constants with associated behavior.
Enum and Inheritance
- Enums by default extend the class java.lang.Enum class in Java. In Java, we can only have one parent class and therefore, enums cannot extend any other classes.
- They can implement multiple interfaces as other Java classes allowing them to provide a certain level of abstraction and polymorphism.
- The method toString() is overridden in the java.lang.Enum class which returns the name of the enum constant.
Enum and Constructor
- Enums as Java classes can have constructors but these are restricted in terms of accessibility. Enum constructors can only be private.
- Implicitly, each enum object invokes an enum constructor with its required arguments.
- We cannot instantiate new enum objects with the new keyword as we do in the case of Java class objects.
FAQs
Q. What is the difference between an enum and a class in Java?
A. Enums are used to define a set of named constants, while classes are used to model objects with attributes and behaviors.
Q. Can enums have methods and constructors?
A. Yes, enums can have methods and constructors. Enums are not limited to constants; they can include behaviors, fields, and constructors. The constructor is called implicitly when each enum constant is created.
Q. How can I loop through enum values in Java?
A. You can loop through enum values using a for-each loop or a traditional for loop. The values() method provides an array of enum constants.
Q. Can enum constants be modified during program execution?
A. No, enum constants are typically immutable. Once defined, their values cannot be changed.
Conclusion
- Enums or Enumerations are special data types to store the sets of predefined constants separated by commas.
- Enum class is created using the enum keyword before the class name in Java. These can be declared inside a class or outside a class.
- The .values() method returns an array of all the objects of the enum class. It can be used to loop through it.
- Enums by default inherit the java.lang.Enum class and thus cannot have any other parent classes but can implement multiple interfaces.
- We can have constructors and main methods inside the enum class.
- Objects of Enums are constant and thus, unlike normal classes its objects cannot be created or modified at runtime.