Enum in JS
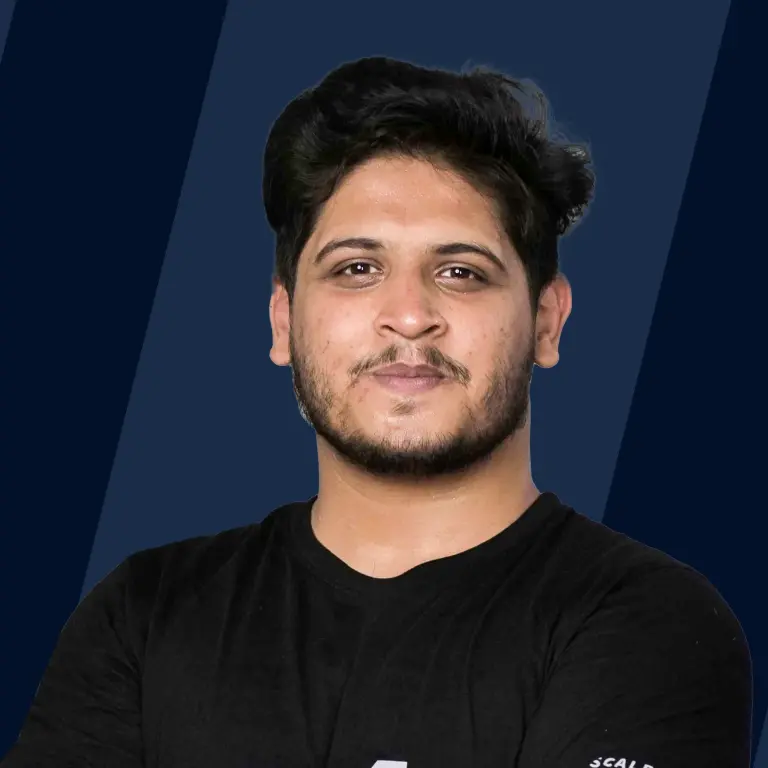
Enum is a data type in programming languages that defines a set of constant values. It is a distinct value type (i.e.) all values in an enum are unique. Using an enum makes the code readable and maintainable. Some examples of enum are all weekdays from Monday to Sunday, T-shirt sizes like S, M, L, XL, etc.
The pseudocode of an enum variable can look like below:
Does JavaScript Support Enum ?
Even though enum data type is widely used in many programming languages like C++, Java, etc., Javascript doesn't have a built-in enum data type. Enum's functionalities can be implemented in Javascript using classes and objects.
Ways to Implement Enum in Javascript
Enum Using Objects
Enums can be implemented in Javascript using objects. The object can have key-value pairs where the key can be treated as the enum value, and the matter can be anything.
Syntax :
Example :
Let's use a Javascript object to implement an enum for the directions such as north, east, west, and south.
One problem with the above implementation is that the values in the Direction object are mutable. Since enums are immutable, we can achieve immutability by freezing the object using the Object.freeze() method.
The Object.freeze() method freezes an object meaning new properties cannot be added, existing properties, and the object's prototype cannot be re-assigned.
The values can be accessed line below :
Enum Using Symbols
One problem we see with the above approach is collision (i.e.) the value of one object may collide with the value of the other object. Let's understand it with the enums Direction and Pole.
The output of the above code will be :
Even though the North direction and North pole are different enum instances, they are treated equally. Symbol can be used to overcome this issue.
Symbol is a built-in object whose constructor returns a value guaranteed to be unique. Let's use symbols to implement the Direction and Pole enum.
The output of the above code is :
The above code returns false because the values of the Direction instance and Pole instance are different, as Symbol returns unique values. Use this Online JavaScript Formatter to format your code.
Enum Using Classes
Enums can be implemented using Javascript classes, where each value will be the static instance of the class itself but with different values.
Let's understand it with an example :
Example :
Let's implement the Direction enum using Javascript classes.
The enum value can be accessed like below :
Output :
When to Use Enums in Javascript ?
Enums can be used in Javascript when it provides the below advantages :
- Improves the readability and maintainability of the code.
- Reduces errors caused by mistyping.
- Useful when there is a fixed number of values like days of the week, months of the year, etc.
Learn About Important Concepts in JavaScript
Please visit Scaler Topics' Javascript hub to learn more about Javascript
Conclusion
- Enum is a data type in programming languages that defines a set of constant values.
- All values in an enum should be unique.
- Although the enum data type is widely used in many programming languages, Javascript doesn't have the enum data type.
- Enum can be implemented using objects, classes, and symbols in Javascript.
- Symbol is a built-in object whose constructor returns a value that is guaranteed to be unique
- Symbol avoids collision between two enums with the same values.