equalsIgnoreCase() in Java
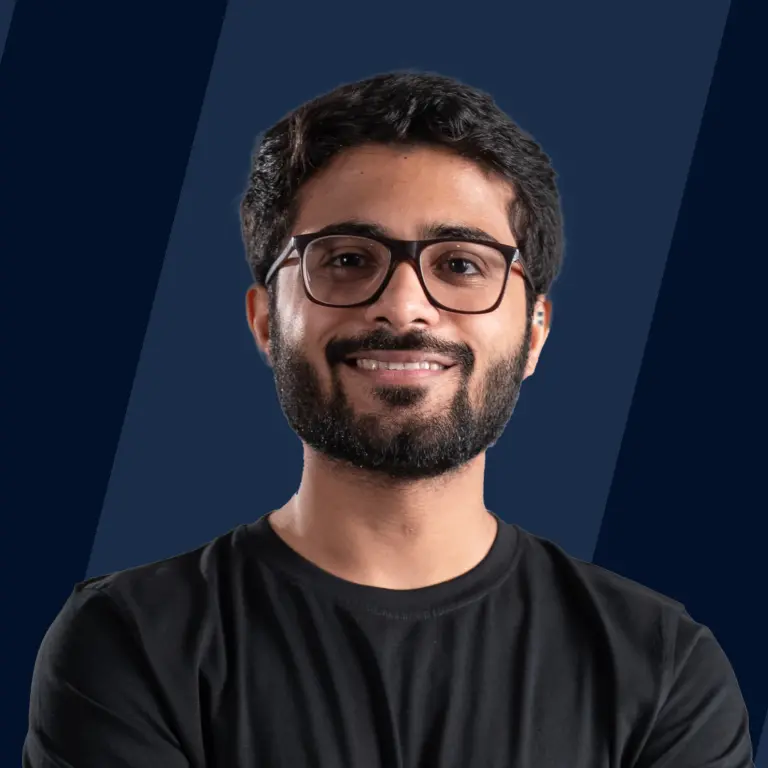
Overview
The equalsIgnoreCase() method in Java compares the specified string with another string, ignoring lower and upper case differences. The method returns true if the strings are equal, and false if not. equalsIgnoreCase() is found in String class of java.lang package.
Syntax of equalsIgnoreCase()
The method signature for equalsIgnoreCase() method is:
The syntax for equalsIgnoreCase() method is:
Here, string1 and string2 are both variables of String type. The object string1 calls the equalsIgnoreCase() method and passes a String argument, i.e., string2.
Note: Here, string2 is a string that needs to be compared with string1.
Parameters of equalsIgnoreCase()
The string equalsIgnoreCase() method accepts a single parameter of String type.
For example: str.equalsIgnoreCase(anotherString)
anotherString - the string to be compared with str.
Return type of equalsIgnoreCase()
Return type : boolean
The string equalsIgnoreCase() method returns a boolean value:
- true - If the corresponding characters of both the strings are equal, ignoring case differences.
- false - If the strings are not equal.
Exceptions of equalsIgnoreCase()
Let's consider a code snippet (string1.equalsIgnoreCase(string2)) to understand the exceptions. The following cases may arise:
- If the input string, i.e., string2 is null, the method will return false, it doesn’t throw any exceptions.
- If string1 is null, the method will throw an exception, i.e., NullPointerException.
Example of equalsIgnoreCase()
Output:
What is equalsIgnoreCase() in Java?
The equalsIgnoreCase() method in java compares the specified string with another string based on the content of the string while ignoring their case, i.e., lower or upper case of the string. Two strings are considered equal if they are of the same length and corresponding characters in the two strings are equal, ignoring case differences.
Two characters, c1 and c2 are considered the same, ignoring the case if at least one of the following is true:
-
The two characters are the same (as compared by the == operator)
-
Applying the method Character.toUpperCase(char) to each character produces the same result
-
Applying the method Character.toLowerCase(char) to each character produces the same result
The following code is the internal implementation of equalsIgnoreCase() method.
As we can see in the above code, the equalsIgnoreCase() method invokes the regionMatches() method, as a result, the equalsIgnoreCase() method becomes case-insensitive.
The regionMatches() method accepts five arguments that are listed below:
- ignoreCase - if true, ignore case while comparing characters.
- toffset - the starting offset of the subregion in this string.
- other - the string argument.
- ooffset - the starting offset of the subregion in the string argument.
- len - the number of characters to compare.
regionMatches(true, 0, anotherString, 0, value.length) : Here, the first parameter ignoreCase is set to true to ignore the case differences. After that, the corresponding characters from each string are taken and compared. If the comparison returns a false value, then both the characters are converted to upper case and checked again. If the comparison returns a false value, both characters are converted to lowercase and compared again. If the comparison returns the true value, then the contents of both strings are equal; otherwise, they are not equal.
One may claim that since we made a comparison after converting to uppercase, why do we need to make another comparison by converting to lowercase. The reason is conversion to uppercase does not work properly for the Georgian alphabets, as they have strange rules about case conversion. Therefore, one extra comparison is required by converting characters to lowercase.
More Examples of equalsIgnoreCase()
Example 1: Java String equalsIgnoreCase()
The following java program determines the equalsIgnoreCase() method.
Output :
Explanation :
- For output 1 : It returns true because the content of both the strings is the same, and the case is ignored.
- For output 2 : It returns false because the content of both the strings is not the same.
- For output 3 : It returns false because str4 is an empty string.
- For output 4 : It returns false because str5 is null.
Example 2: Check if Two Strings are Equal
In the following example, we will check if two strings are equal or not by comparing the string “ScAlER” with the string “Scaler”. And it returns true because the content of both the strings is the same, also, the case is ignored.
Output :
Explanation : It returns true because the content of both the strings is the same, and the case is ignored.
Example 3: Comparing a String with ArrayList elements
In the following example, we are comparing the string “apple” with all the ArrayList elements, and if a match is found, the condition's statement is printed.
Output :
Explanation : Here, we are searching for the string "apple" in the fruits ArrayList. As the string is present in the ArrayList, it prints the statement inside the advanced for loop.
Conclusion
- The method equalsIgnoreCase() is found in the java.lang.String class.
- The method equalsIgnoreCase() in java compares a string with another string, ignoring the case differences.
- The String method equalsIgnoreCase() accepts a single argument that is a String, and returns a boolean value.
- The equalsIgnoreCase() method will return true if both the strings are equal, else false.
- The equalsIgnoreCase() method doesn’t throw an exception, if the input string is null, it will return false.