Escape Sequence in Java with Examples
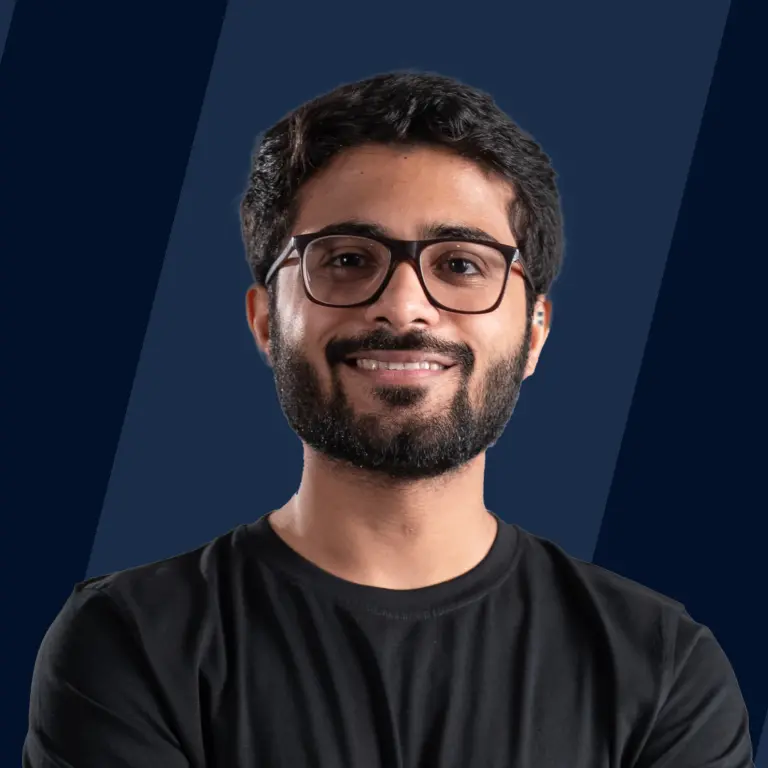
Overview
Escape sequences or escape characters play a crucial role in various programming languages, including Java. These special characters are employed with strings to represent characters that might be challenging to express directly. Typically, escape sequences are initiated by a backslash (\). Their utility extends beyond Java and can be observed in many programming languages. Some common use cases across different languages encompass string formatting, input validation, regular expression (RegEx) matching, SQL queries, and more. In the context of regular expression matching, escape sequences match characters with special meanings.
What is Escape Sequence and Escape Characters in Java
As students or aspiring programmers, you've likely encountered characters within a string that are preceded by a backslash (). These characters are referred to as Escape Sequences or Escape Characters, and they play a crucial role in various programming languages, including Java, Python, and C++.
Escape sequences in Java aim to represent those characters in a string that are difficult to represent directly. We can achieve certain formatting effects and can print certain effects using escape sequences in Java. The escape sequences must be enclosed in double quotes (""). There are a total of 8 escape sequences in Java. They are as follows:
Escape Sequences | Purpose |
---|---|
\n | It is used to insert a new line. |
\t | It is used to insert a tab. |
\r | It is used to represent a carriage return. |
\' | It is used to insert a single quotation mark. |
\" | It is used to insert a double quotation mark. |
\\ | It is used to insert a backslash. |
\f | It is used to insert a form feed. |
\b | It is used to represent a backspace character. |
The Java compiler interprets these escape sequences as a single character that contains a specific meaning.
Importance of Escape Sequences
You might wonder about the importance of Escape Sequences in Java. Let us look at some scenarios where Escape Sequences in Java are required.
1.
Suppose we want to print the statement- "I am learning "Java" and it is interesting". Let us try and print it conventionally.
The output is
It happens because we haven't used the quotation marks properly. Because of this, the compiler gets confused. It interprets the string as "I am learning " followed by a part not enclosed properly, and then " and it is interesting". In this case, we can enclose a string within double quotation marks using the \" escape sequence. We will comprehend this escape sequence in Java in the upcoming section.
2.
Consider the following statement to be printed- "Current directory E:\conf\code".
What do you expect as the output? The output is-
Even this code will encounter an error. This is because when the compiler encounters \, it expects an escape character to follow it. Here, there is no such character, which confuses the compiler. To use \ as the character in our string, we need to use the \\ escape sequence.
Coding Examples of Escape Characters in Java
We will cover an example for each escape sequence character in Java.
1. Code for \n Escape Sequence in Java
As we know, Java's \n escape sequence is used for a new line feed. It is used to format multi-line strings, for example- displaying the text with proper line breaks in the console.
Example
Output
The compiler's control moves to the new line after encountering \n.
2. Code for \t Escape Sequence in Java
\t escape sequence in Java is used for inserting a tab. It is used to format data in the tables.
Example
Output
The compiler adds a tab character after encountering \t.
3. Code for \r Escape Sequence in Java
\r escape sequence in Java is used for inserting a carriage return. Carriage return means returning to the current line's beginning without advancing downward. This might vary from compiler to compiler.
Note- The \r escape sequence might also be used to overwrite in a few cases. It moves the cursor to the beginning of the current line instead of the new line, which also leads to an overwrite effect.
Example
Output (Might vary from compiler to compiler)
The compiler adds a carriage return after encountering \r.
4. Code for \ Escape Sequence in Java
As we know, \\ escape sequence in Java is used for inserting a backslash character.
Example
Output
The compiler prints a backslash when it encounters\\.
5. Code for ' Escape Sequence in Java
\' escape sequence in Java is used for inserting a single quote character.
Example
Output
The compiler prints a single quote when it encounters\'.
6. Code for " Escape Sequence in Java
\" escape sequence in Java is used for inserting a double quote character.
Example
Output
The compiler prints a double quote when it encounters\".
7. Code for \f Escape Sequence in Java
\f escape sequence in Java inserts a form feed character. It is an old way to represent a page break.
Example
Output
The compiler prints a page break when it encounters \f.
8. Code for \b Escape Sequence in Java
\b escape sequence in Java is used for inserting a backspace character. It moves one character back and may not delete the previous character. They can be used for user input validations where the users delete input characters.
Example
Output
The compiler inserts a backspace character when it encounters \b.
FAQs
Q. What are escape sequences in Java used for?
A. Escape sequences in Java represent special characters in strings that are difficult to express directly.
Q. What does the escape sequence \n do?
A. It is used to insert a new line.
Q. How do you use single or double quotes within strings?
A. We can use the escape sequence \' for a single quote and \" for a double quote.
Conclusion
- Escape sequences in Java aim to represent those characters in a string that are difficult to express directly.
- The escape sequence characters are preceded by a backslash (). The escape sequences must be enclosed in double quotes (""). There are a total of 8 escape sequences in Java.
- We can achieve certain formatting effects and print specific effects using escape sequences in Java.
- \n escape sequence in Java is used for a new line feed, \t escape sequence in Java is used for inserting a tab, \r escape sequence in Java is used for inserting a carriage return, \\ escape sequence in Java is used for inserting a backslash character.
- \' escape sequence in Java is used for inserting a single quote character, \" escape sequence in Java is used for inserting a double quote character, \f escape sequence in Java is used for inserting a form feed character, and \b escape sequence in Java is used for inserting a backspace character.