Even Odd Program in Python
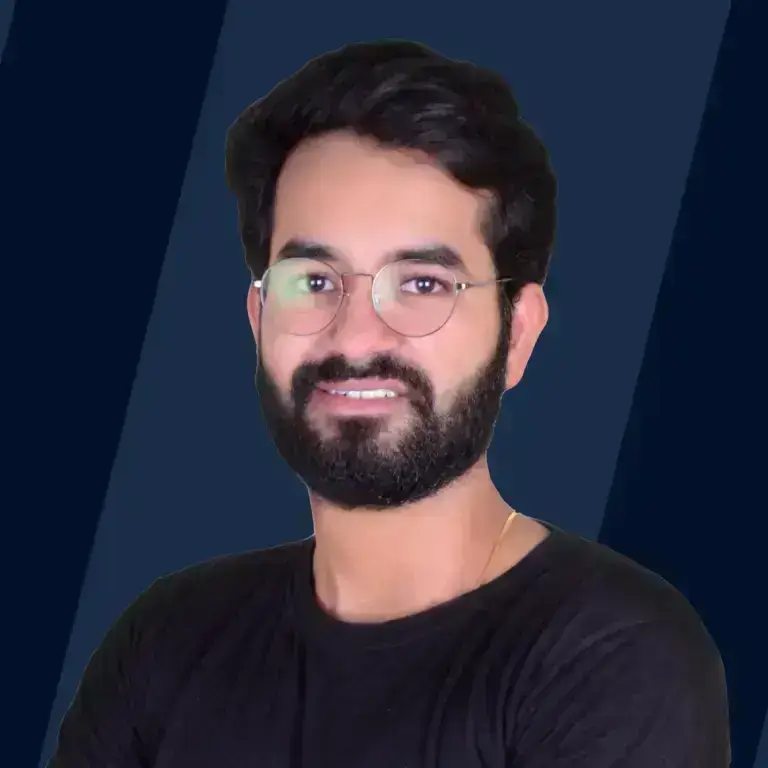
Even numbers are divisible by 2 with no remainder. It ends with 0, 2, 4, 6, or 8 (e.g., 74). where Odd numbers leave a remainder when divided by 2, and end in 1, 3, 5, 7, or 9 (e.g., 71). Zero is an even number. Now, let's code the even and odd programs in Python by using the divisibility logic.
Note: The number 0 is considered an even number. As 0 ends with one of the numbers (0, 2, 4, 6, 8).
Check Even or Odd Number in Python
Let us code the even and odd numbers in python program.
Even Odd Program in Python Using Division
We will divide the given number by 2. If the number gets completely divided by 2 then the number is an even number. Else, the number is not an even number (i.e. odd number).
The pseudoscope for the even off program in python can be:
Function Name: even_odd(number)
Note: We can use the modulo operator in python % to find the remainder. The modulo operator in python returns the remainder of a division between two numbers.
Code:
Output:
Check Even or Odd Numbers using recursion in Python
We can also use recursion in even-odd programs in python. We will call the recursive function until it is greater than 1, it become 0 then called even and called odd.
Output:
Even Odd Program in Python Using Bitwise Operator
We can also use Bitwise Operators in even-odd programs in python. Bitwise Operators are the operators that help us to perform bit (bitwise) operations. Bit operators are ~, << >>, &, ^, |.
Using the bitwise operator, we will check if the last bit of the number is set or not. If the last bit is set then the number must be odd. Else, the number will be an even number.
Code:
Output:
Conclusion
- Even numbers are the numbers that can be completely divided by 2 without leaving any remainder.
- On the other hand, odd numbers are the numbers that can not be completely divided by 2 (leaves a remainder when divided by 2).
- We can code the even-odd program in python using divisibility logic and bitwise operator.