Exception Handling in PL/SQL
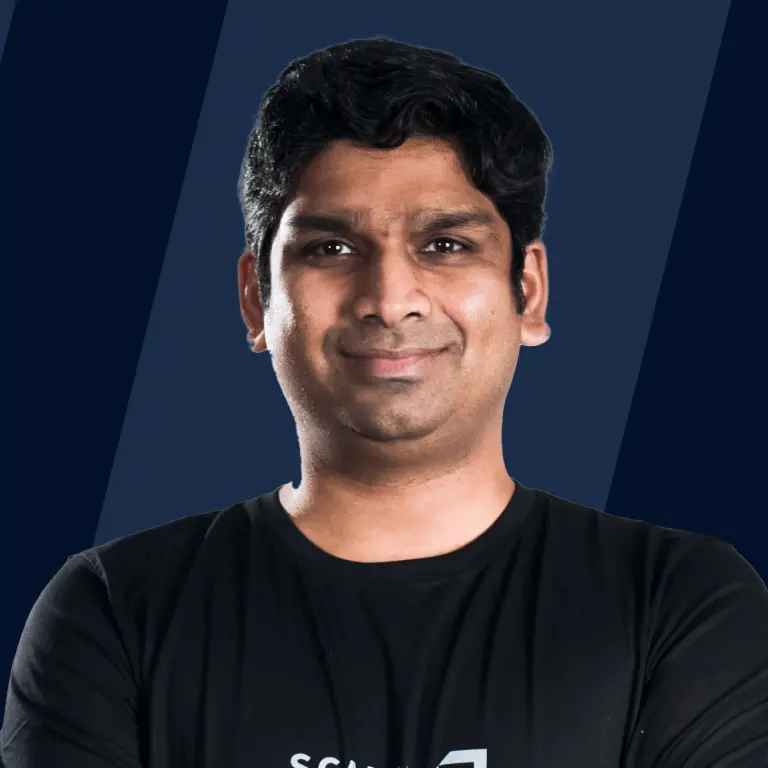
Overview
The topic of Exceptions in PL/SQL will be covered in this article. A program execution error circumstance is an exception. Using the EXCEPTION block in the program, PL/SQL enables programmers to catch certain conditions, and the required action is then taken to address the error condition. Two types of exceptions exist.
What is Exception Handling in PL/SQL?
In PL/SQL, an error that happens while the program runs is an exception.
Programmers can capture these occurrences using exception blocks in PL/SQL, and the error condition is handled appropriately.
Two types of exceptions exist:
- System-defined Exceptions
- User-defined Exceptions
Syntax for exception handling
Below is the syntax for exception handling in pl sql
Note: When other keyword should only be used at the conclusion of the exception handling block because when the control executes the when others, the block will be closed, and no subsequent exception handling code will be executed.
The syntax represents the basic structure of an exception-handling block in PL/SQL. It is used to handle exceptions that may occur during the execution of PL/SQL code. Here's an explanation of each part:
- DECLARE: This section is used to declare variables, cursors, and other PL/SQL constructs used within the block's executable section.
- BEGIN: This is the beginning of the executable section. It contains the actual PL/SQL statements and commands you want to execute. If an exception occurs during the execution of these statements, the control will transfer to the appropriate exception handler.
- EXCEPTION: This keyword marks the beginning of the exception handling section. It is followed by one or more exception handlers.
- WHEN exception1 THEN statement1: This is an exception handler. It specifies a particular exception (exception1) and the action to be taken (statement1) if that exception is raised during the execution of the executable section.
- WHEN exception2 THEN statement2: Similarly, you can have multiple exception handlers to handle different exceptions with specific actions.
- [WHEN others THEN]: This is an optional part. If none of the specific exception handlers match the raised exception, the control will transfer to the WHEN OTHERS handler (if it is defined). The WHEN OTHERS handler can handle generic or default exceptions.
This structure allows you to define how your PL/SQL code should respond to different exceptions. You can have specific handlers for specific exceptions and a generic handler for any other unhandled exceptions. This helps in gracefully handling errors and exceptions in your PL/SQL programs.
Example of exception handling
In this example:
- We declare two variables, num1 and num2, and initialize num2 with a value of 0.
- Inside the BEGIN block, we attempt to divide num1 by num2, which will cause a "division by zero" exception.
- We then have an exception handling section:
- WHEN ZERO_DIVIDE THEN is a specific exception handler for the "division by zero" exception.
- WHEN OTHERS THEN is a generic handler for any other unhandled exceptions.
- If a "division by zero" exception occurs, the first handler will be triggered, printing an error message. If any other unexpected exception occurs, the generic handler will be triggered, printing a different error message.
- Using exception handling like this allows you to gracefully handle errors and exceptions in your PL/SQL code, ensuring that your application doesn't terminate unexpectedly due to errors.
Raising Exceptions
When an internal database issue occurs, the server automatically raises exceptions, but programmers can also directly raise exceptions using the command RAISE. The simple syntax for raising an exception is as follows:
The previously mentioned syntax can be used to raise either an Oracle standard exception or a user-defined exception. The following section will provide an example of raising a user-defined exception. The Oracle standard exceptions can also be raised in a similar manner.
User-defined Exceptions
You can create your exceptions in PL/SQL based on the requirements of your program. The function DBMS_STANDARD.RAISE_APPLICATION_ERROR or a RAISE statement must be used to specify and raise a user-defined exception expressly.
User-defined exceptions in PL/SQL allow you to define and handle custom exception conditions in your code. Here's an example of how to define and use a user-defined exception:
In this example:
- We declare a user-defined exception named insufficient_balance.
- We initialize account_balance to 1000 and withdrawal_amount to 2000.
- Inside the BEGIN block, we check if there's enough balance for the withdrawal. If not, we raise the insufficient_balance exception.
- We have an exception handling section:
- WHEN insufficient_balance THEN is a specific handler for our user-defined exception. It prints an error message.
- WHEN OTHERS THEN is a generic handler for any other unhandled exceptions.
- If the withdrawal amount exceeds the account balance, the insufficient_balance exception is raised, and the corresponding error message is printed. Otherwise, the withdrawal is processed, and the new balance is displayed.
User-defined exceptions allow you to create custom error conditions specific to your application's logic and provide a way to handle them gracefully.
Pre-defined Exceptions
Many pre-defined exceptions are available in PL/SQL and are used if a program breaks a database rule. For instance, when a SELECT INTO operation returns no rows, the predefined exception NO_DATA_FOUND is raised. A handful of the significant pre-defined exceptions are listed in the following table:
Exception Name | Oracle Error | SQLCODE | Description |
---|---|---|---|
ACCESS_INTO_NULL | 06530 | -6530 | Occurs when a null object is automatically assigned a value. |
CASE_NOT_FOUND | 06592 | -6592 | Raised when no choice in a CASE statement matches, and no ELSE clause is provided. |
COLLECTION_IS_NULL | 06531 | -6531 | Raised when collection methods are applied to an uninitialized nested table or varray. |
DUP_VAL_ON_INDEX | 00001 | -1 | Raised when duplicate values are inserted into a column with a unique index. |
INVALID_CURSOR | 01001 | -1001 | Occurs when an invalid cursor operation is attempted, such as closing an unopened cursor. |
INVALID_NUMBER | 01722 | -1722 | Raised when a character string cannot be converted into a valid number. |
LOGIN_DENIED | 01017 | -1017 | Occurs when invalid credentials are used while attempting to log in to the database. |
NO_DATA_FOUND | 01403 | +100 | Raised when a SELECT INTO statement returns no rows. |
NOT_LOGGED_ON | 01012 | -1012 | Occurs when a database call is issued without an active connection to the database. |
PROGRAM_ERROR | 06501 | -6501 | Raised when there is an internal problem in PL/SQL. |
ROWTYPE_MISMATCH | 06504 | -6504 | Occurs when there is a data type mismatch during a cursor fetch operation. |
SELF_IS_NULL | 30625 | -30625 | Raised when a member method is invoked on an uninitialized object instance. |
STORAGE_ERROR | 06500 | -6500 | Raised when PL/SQL runs out of memory or memory corruption is detected. |
TOO_MANY_ROWS | 01422 | -1422 | Occurs when a SELECT INTO statement returns more than one row. |
VALUE_ERROR | 06502 | -6502 | Raised when arithmetic, conversion, truncation, or size constraint errors occur. |
ZERO_DIVIDE | 01476 | 1476 | Raised when an attempt is made to divide a number by zero. |
Conclusion
- In conclusion, exception handling in PL/SQL is critical for managing errors and unexpected situations in your database programs. It allows you to:
- Catch and Handle Errors: You can define and specify how your program should respond to specific error conditions using exception handlers. This prevents your program from terminating abruptly when errors occur.
- Predefined and User-Defined Exceptions: PL/SQL provides both predefined exceptions (such as NO_DATA_FOUND or VALUE_ERROR) and the capability to define custom, user-defined exceptions to suit your application's needs.
- Robust Error Reporting: Exception handling provides a structured way to report and log errors, making diagnosing and resolving issues in your applications easier.
- Control Flow: By handling exceptions, you can gracefully control the flow of your program, allowing it to recover from errors and continue executing other parts of the code.
- Nested Exception Handling: PL/SQL supports nested exception handling, allowing you to handle exceptions at different levels of your program, improving code modularity and readability.
- Exception Propagation: Exceptions can be propagated up the call stack, providing a mechanism for higher-level code to handle errors detected in lower-level routines.
- In summary, exception handling is essential to robust PL/SQL programming. It enables you to create applications that can handle errors gracefully, maintain data integrity, and provide meaningful error messages to users or developers, ultimately enhancing the reliability and maintainability of your database programs.