Executor Framework in Java
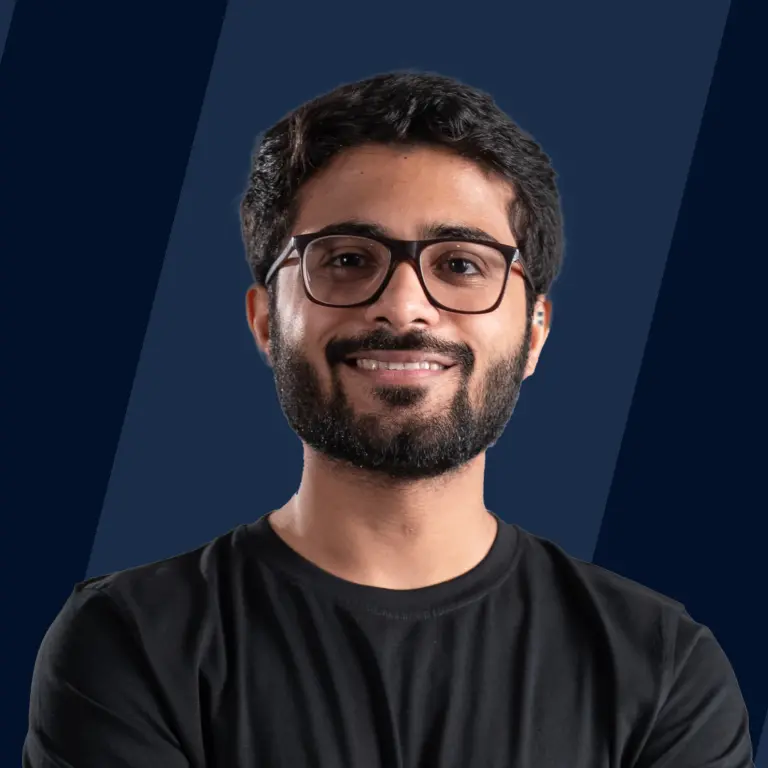
Overview
The Executor Framework in Java is a powerful and essential component for managing and controlling the execution of concurrent tasks within a Java application. The framework makes it easier to handle multiple tasks at the same time without needing to deal with the tricky details of managing them individually. It does this by providing a group of worker threads that can do these tasks all at once, which helps the application work better and use resources more effectively.
What is the Executor Framework in Java?
The Executor Framework in Java is a high-level abstraction for managing and controlling the execution of concurrent tasks within a Java application. It provides a structured way to handle multi-threading by abstracting away the complexities of thread creation, management, and task scheduling. The key components of the Executor Framework include:
-
Executor Interface:
This is at the core of the framework, defining a simple execute(Runnable) method that takes a task (typically implemented as a Runnable or Callable), which is then submitted for execution by a thread from a pool of worker threads.
-
ThreadPoolExecutor:
This is one of the most commonly used implementations of the Executor interface. It manages a pool of worker threads and provides fine-grained control over thread management, allowing you to specify parameters like the core pool size, maximum pool size, and various queueing strategies.
-
ScheduledExecutorService:
This extends the Executor framework to support scheduling tasks to run at specific times or with fixed time intervals. It's particularly useful for implementing tasks that need to be executed periodically, such as cleanup tasks or periodic data updates.
-
Executors Utility Class:
Java provides the Executors utility class, which offers factory methods for creating instances of Executor and ScheduledExecutorService. It simplifies the process of creating thread pools with various configurations.
Types of Executor Framework
Let's discuss about the types of Executor Framework in Java:
1. SingleThreadExecutor
The SingleThreadExecutor is one of the executor types provided by the Executor Framework in Java. It is designed to execute tasks sequentially, one at a time, on a single worker thread. Here's an explanation of the SingleThreadExecutor:
-
Sequential Execution:
SingleThreadExecutor ensures that tasks submitted to it are executed one after the other, in the order they were submitted. This is useful in scenarios where you want to guarantee sequential execution of tasks, ensuring that they don't run concurrently.
-
Worker Thread:
It maintains a single worker thread to execute tasks. This thread is created and managed by the executor. Tasks are executed in a first-in, first-out (FIFO) order on this dedicated thread.
-
No Parallelism:
Unlike other executor types that use multiple threads for concurrent execution, SingleThreadExecutor is single-threaded. This makes it suitable for tasks that require exclusive access to resources or tasks that must execute sequentially.
Syntax:
Explanation:
- newSingleThreadExecutor() creates a single-threaded executor.
- execute(...) submits a task for execution on a single thread.
- shutdown() is used to gracefully shut down the executor when done.
2. FixedThreadPool(n)
The FixedThreadPool(n) is another type of executor provided by the Executor Framework in Java. It creates a fixed-size thread pool with a specified number of worker threads. Here's an explanation of FixedThreadPool(n):
-
Fixed Number of Threads:
FixedThreadPool maintains a fixed number of worker threads in the pool, exactly as specified by the parameter 'n' when creating the executor. These threads are created at initialization and remain active throughout the lifetime of the executor.
-
Thread Reuse:
The threads in a FixedThreadPool are reused to execute tasks. When a task is submitted, it is assigned to one of the available threads for execution. If all threads are busy, additional tasks are queued until a thread becomes available.
-
Optimal for Controlled Concurrency:
This type of executor is ideal when you need to control the level of concurrency in your application. You can precisely specify how many threads are available for executing tasks, which can help manage resource usage and prevent resource contention.
-
Blocking Queue:
Tasks that are submitted to a FixedThreadPool and can't be immediately executed due to all threads being busy are placed in a blocking queue. They are executed as soon as a thread becomes available.
Syntax:
Explanation:
- newFixedThreadPool(n) creates a fixed-size thread pool with n threads.
- execute(...) submits a task for execution on one of the 'n' threads.
- shutdown() is used to gracefully shut down the executor when done.
3. CachedThreadPool
The CachedThreadPool is another type of executor provided by the Executor Framework in Java. It creates a thread pool that can dynamically adjust the number of worker threads based on the workload. Here's an explanation of CachedThreadPool:
-
Dynamic Thread Count:
CachedThreadPool doesn't have a fixed number of threads like FixedThreadPool. Instead, it dynamically adjusts the number of threads in the pool based on the number of tasks and the workload.
-
Thread Reuse:
Threads in a CachedThreadPool are reused to execute tasks. When a task is submitted, it is assigned to an available thread for execution. If no threads are available, a new thread is created. Threads that remain idle for a certain period are terminated and removed from the pool to save resources.
-
Suitable for Bursty Workloads:
This type of executor is well-suited for scenarios with bursty workloads or when the number of tasks varies widely. It can efficiently allocate threads as needed and shrink the thread pool during periods of low activity.
Syntax:
Explanation:
- newCachedThreadPool() creates a thread pool that dynamically adjusts the number of threads based on workload.
- execute(...) submits a task for execution on an available thread.
- shutdown() is used to gracefully shut down the executor when done.
4. ScheduledExecutor
The ScheduledExecutor is a type of executor provided by the Executor Framework in Java that is specifically designed for scheduling tasks to run at specified times or with fixed time intervals. Here's an explanation of ScheduledExecutor:
-
Task Scheduling:
The primary purpose of ScheduledExecutor is to schedule tasks to run at specific times in the future or at regular intervals. This is particularly useful for automating periodic tasks, such as data updates, backups, or sending out notifications.
-
Multiple Implementations:
Java provides several implementations of ScheduledExecutor, with the most common one being ScheduledThreadPoolExecutor. This allows you to choose the level of concurrency and scheduling flexibility that suits your application.
-
Delayed Execution:
You can schedule tasks to run after a certain delay from the current time or at an absolute time in the future. This flexibility enables you to precisely control when tasks should be executed.
-
Recurring Tasks:
ScheduledExecutor can also be used to schedule recurring tasks that run at fixed time intervals. For example, you can schedule a task to run every hour or every day.
Syntax (for scheduling a task to run after a delay):
Explanation:
- newScheduledThreadPool(1) creates a scheduled executor with one thread.
- schedule(...) schedules a task to run after a specified delay (5 seconds in this example).
- shutdown() is used to gracefully shut down the executor when done.
Creating a Simple Executor Framework in Java
Let's create a basic example using a FixedThreadPool with three threads. In this example, we'll submit three tasks to the Executor, and they will run concurrently.
Implementation
Output
Output Explanation
- We create a FixedThreadPool with 3 threads using Executors.newFixedThreadPool(3).
- We submit three tasks to the executor using executor.execute(...). Each task is represented by a Runnable and prints a message indicating its ID and the thread it's running on.
- The tasks are executed concurrently by the three threads in the thread pool.
- The output shows that each task is running on one of the three threads from the pool (pool-1-thread-1, pool-1-thread-2, and pool-1-thread-3).
- Finally, we shut down the executor using executor.shutdown() to gracefully terminate the thread pool when all tasks are completed.
FAQs
Q. What is the difference between submit() and execute() in the Executor Framework?
A. submit() returns a Future for task result tracking, while execute() is void and used for fire-and-forget tasks.
Q. Can you reuse an executor after it has been shut down?
A. No, once an executor is shut down using the shutdown() method, it cannot be reused. You would need to create a new executor instance if you want to continue submitting tasks.
Q. What is the benefit of using a CachedThreadPool over a FixedThreadPool?
A. A CachedThreadPool dynamically adjusts its thread count based on workload, potentially reducing resource usage during periods of low activity. In contrast, a FixedThreadPool maintains a constant number of threads, which might be less efficient for variable workloads.
Q. Is it necessary to explicitly shut down an executor when done using it?
A. It's considered a best practice to shut down an executor when you're done with it to release its resources. Failure to do so might lead to resource leaks and prevent the Java application from terminating properly.
Conclusion
- Concurrency Management: The Executor Framework simplifies concurrent programming in Java, providing abstractions for managing and controlling the execution of tasks concurrently.
- Thread Pooling: It offers thread pooling capabilities, allowing you to reuse threads for executing tasks, reducing the overhead of thread creation and destruction.
- Types of Executors: Java provides various executor types, including SingleThreadExecutor, FixedThreadPool, and CachedThreadPool, catering to different concurrency needs.
- Task Scheduling: The ScheduledExecutorService component enables scheduling tasks to run at specific times or with fixed intervals, making it useful for periodic tasks and time-based operations.
- Resource Efficiency: Executor Framework promotes efficient resource utilization by managing the number of threads and providing control over the thread lifecycle.
- Error Handling: It abstracts error handling and task cancellation, making it easier to deal with exceptions and failures in concurrent code.
- Cleaner Code: Using the framework leads to cleaner, more readable code by encapsulating low-level threading details.
- Shutdown Mechanism: Properly shutting down the executor is crucial to avoid resource leaks, and the framework provides methods like shutdown() and awaitTermination() for graceful termination.
- Scalability: It helps in building scalable applications by efficiently distributing tasks across available threads.
- Concurrency Control: Developers can control the level of concurrency, manage thread pools, and prioritize tasks using various configuration options.
- Enhanced Performance: Leveraging the Executor Framework can lead to enhanced application performance by utilizing available CPU resources effectively.
- Key Component of Java Concurrency: It is a fundamental component of Java's concurrency toolkit, essential for writing robust and high-performance multithreaded applications.