Math exp() Method in Java with Example
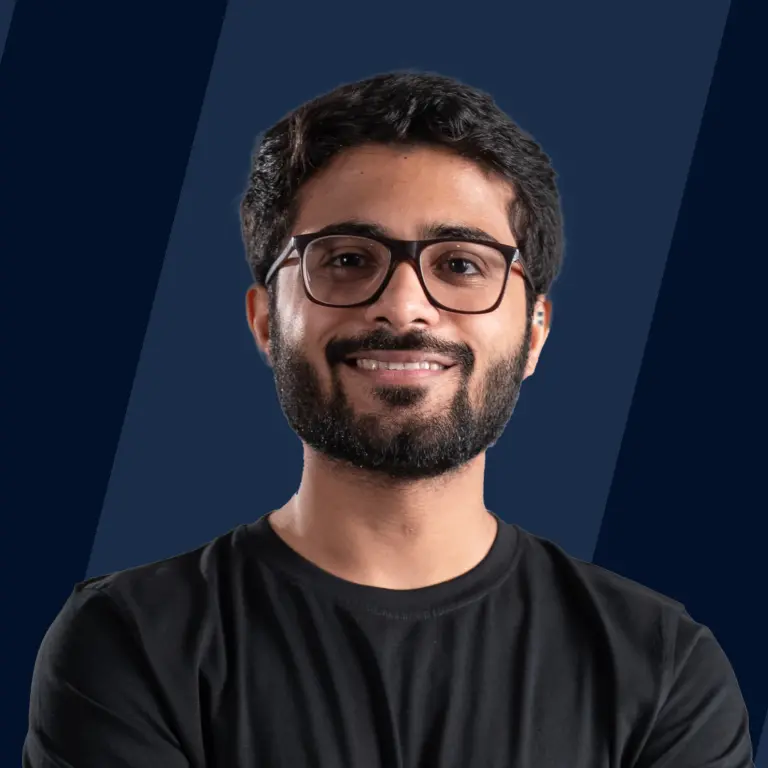
Overview
In the field of programming, mathematics is essential for resolving complex problems. Java, being a flexible programming language, provides a wide range of mathematical functions through its Math class. One such function is the exp() in Java which enables you to determine the exponential value of a given number. In this article, we'll examine the exp() method in Java, comprehend how it works, and give examples to demonstrate its features.
The java.Lang.Math.exp() Method in Java
The exp() method of the Math class is static in Java. It determines the exponential value of the double-precision floating-point number that is supplied. The exponential function is represented by e^x which denotes the the value of Euler's number 'e' raised to the power of 'x'. It is present in the java.lang package of Java.
In the above image, x is the exponential value that is raised to the power of e.
Syntax
The syntax for the exp() in Java of the Math class is as follows:
In the above syntax, x is the argument passed to the exp() method which has a data type of double.
Now, let us see an example of how can we use Math.exp() in Java in a program.
In the above example, we have used the Math.exp() method with an argument x passed to it. The variable x has a value of 5, therefore the value of e^5 will be stored in the variable a.
Parameters
The method Math.exp() in Java takes only one parameter
- x - which will be raised to the power of e.
Return Value
The return value of the method Math.exp() in Java would be e^x where x is the argument passed to the method. The return type of the method would be a double value.
Note: The Euler's number e has the value of 2.71828.
Examples
Now, let us understand the Math.exp() method in Java with the help of examples.
Output:
As you can see in the above code example, the statement at line 6 calculates the value of e^x. We have given the variable x a value of 3. After which we have given this value of x to the Math.exp() method as an argument which calculates and prints the value of e^3. Therefore, the method Math.exp() calculates the exponentiation of a number given to it as an argument.
The time complexity for calculating the exponential value of x would be constant, that is, O(1) which makes it efficient for calculating exponentials of a number.
Note: However, you could also achieve this without the help of this method. By using the Math.pow() method of the Math class, we could also find the value of e^x.
The Math.pow() method in Java can be used to calculate exponentiation, allowing you to raise a base number to a specified exponent. It takes two arguments: the base and the exponent, and returns the result of the base raised to the power of the exponent. On the other hand, the Math.exp() method takes only one argument, that is, the exponent value.
Below are some key differences between the two methods in Java.
exp() | pow() |
---|---|
It takes only one argument that is the number to which you want to apply the exponential function. | It requires two arguments that is the base and the exponent. |
It calculates the exponential function, raising e (approximately 2.71828) to the power of the given number. | It calculates exponentiation, raising the base to the specified power. |
It returns a double value that is the result of the exponential function. | It returns a numeric value that is the result of the exponentiation. |
FAQs
Q. What does the Math.exp() method do?
A. The Math.exp() method calculates the value of 'e' (Euler's number) raised to the power of the given argument 'x', where 'x' is a double value. The result is returned as a double value.
Q. How is Math.exp() different from the Math.pow() method?
A. The Math.exp() method specifically calculates 'e' raised to the power of the given argument, while the Math.pow() method can be used to calculate the value of any arbitrary base raised to any arbitrary exponent value.
Q. What is the return type of the Math.exp() method?
A. The Math.exp() method returns a double value, which denotes the result of 'e' raised to the power of the given argument, that is, the exponential value of the given argument.
Conclusion
- The exp() in Java is a part of the Math class in Java which is used to calculate the value of e raised to the power of a number.
- It takes a single argument for which the exponential value of the number is to be calculated. The method returns a double value as a result of the exponential value of a number.
- The exponential value of a number can also be calculated using the pow() method of the Math class.
- The time complexity for calculating the exponential value of a number using the exp() method is O(1).