What is Expression in Java?
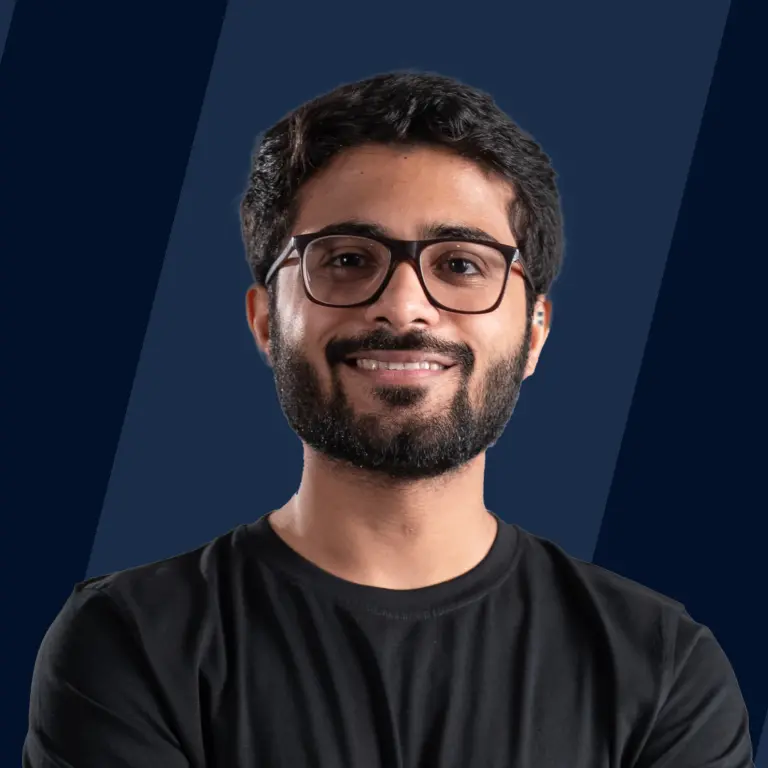
An expression in Java is a construct that evaluates to a single value. This value can be a number, a string, an object, or any other data type depending on the components of the expression. Expressions can include variables, literals, method calls, and operators.
For example:
In the above code, the assignment marks = 90 itself is an expression. Specifically, it's an assignment expression, and it evaluates to the value on the right-hand side, which is 90.
Another example:
In this example, a + b - 3.4 is an arithmetic expression that evaluates to the sum of a and b minus 3.4.
Simple Expressions
A simple expression in Java can be a literal, a variable name, or a method call without any operations involved. For instance:
Compound Expressions
Compound expressions in Java involve the use of operators and typically combine one or more simple expressions to form a more complex expression. By leveraging operators, these expressions integrate their constituent parts into a cohesive whole.
To understand this better, let's consider an example:
In the above example, a + b - 3.4 is a compound expression that combines the simple expressions a, b, and 3.4 using the addition and subtraction operators.
How to Evaluate Expression in Java?
Types of Operators
-
Additive Operators These operators are used to manipulate numeric values through addition or subtraction:
- Addition - Syntax: operand1 + operand2. This returns the sum of two operands. For example, 3 + 2 = 5.
- Subtraction - Syntax: operand1 - operand2. This returns the result of subtracting the second operand from the first. For example, 5 - 3 = 2.
- Post-decrement - Syntax: variable--. This subtracts 1 from the variable's value and returns the original value before decrementing.
- Post-increment - Syntax: variable++. This adds 1 to the variable's value and returns the original value before incrementing.
- Pre-decrement - Syntax: --variable. This subtracts 1 from the variable's value and then returns the decremented value.
- Pre-increment - Syntax: ++variable. This adds 1 to the variable's value and then returns the incremented value.
- String Concatenation - Syntax: operand1 + operand2. When used with strings, the + operator concatenates the two strings.
-
Array index operator This operator is used for accessing the elements of an array through its index. Syntax: variable[index]
-
Assignment operator It is used for assigning the value of the expression to a variable. Example: i=3 The compound assignment operators like, *(+=, -=, =, /=, %=, &=, |=, ^=, <<=, >>=, >>>=) also helps in evaluating the expression.
-
Bitwise Operator These operators perform operations on the individual bits of integer types.
- Bitwise AND - Syntax: operand1 & operand2. Resultant bit is set to 1 only if both corresponding operand bits are 1.
- Bitwise Complement - Syntax: ~operand. It inverts the bits of its operand. If a bit is 1, it becomes 0, and vice-versa.
- Bitwise Exclusive OR (XOR) - Syntax: operand1 ^ operand2. Resultant bit is set to 1 if the corresponding bits of the operands are different.
- Bitwise Inclusive OR - Syntax: operand1 | operand2. The resultant bit is set to 1 if either (or both) of the operand bits is 1.
-
Cast Operator Denoted as (type), it's used to convert one primitive type to another. For example, to convert a float 3.0 to a 32-bit integer 3, you would use (int)3.0.
-
Conditional Operator These operators are used for conditional evaluations based on boolean expressions.
-
Ternary Conditional - Syntax: condition ? valueIfTrue : valueIfFalse. If the condition is true, the operator returns valueIfTrue, otherwise it returns valueIfFalse. Example: boolean status = true; int statusInt = status ? 1 : 0;.
-
Conditional AND (Logical AND) - Syntax: operand1 && operand2. Returns true only if both operands are true. Short-circuits if operand1 is false. Example: false && true returns false.
-
Conditional OR (Logical OR) - Syntax: operand1 || operand2. Returns true if at least one of the operands is true. Short-circuits if operand1 is true. Example: true || false returns true.
-
-
Equality Operator Used to compare two operands for equality or inequality.
-
Equality - Syntax: operand1 == operand2. Returns true if the operands are equal. Example: 'a' == 'b' returns false.
-
Inequality - Syntax: operand1 != operand2. Returns true if the operands are not equal. Example: 'a' != 'b' returns true.
-
-
Logical operator These are the boolean equivalent of the bitwise operator. The following are the types of logical operators:
- Logical AND- syntax: operand1 & operand2, here each operand is of type boolean. It returns true if both the operands are true, else false. For example, true and false= false.
- Logical complement- syntax: !operand, it flips the value of operand, i.e if the value of the operand is true then the output is false, and vice-versa.
-
Member access operator It is denoted by “.” and is used for accessing a class or its members. For instance, a name is a type of string and the length of the string is accessed by using the method, name.length() on that string. This length() method is used for accessing the members of the object.
-
Method call operator It is denoted as “()” and it signifies that a method is called. It identifies the order, number, and type of expression that is passed to the method. For instance, System.out.println(“Scaler”) denotes that the method println is called and is a member of the system class.
-
Multiplicative operator It is mostly used for increasing or decreasing a numeric value by multiple addition or subtraction. The operators include:
- Multiplication - syntax: operand1 * operand2. It returns the output as a multiplication of two values. Example, "3*4=12".
- Division - syntax: operand1 / operand2. It divides operand1 by operand2. For example, 15/5=3.
- Remainder - syntax: operand1 % operand2. It divides operand1 by operand2 leaving behind the remainder. For example, 14%3= 2.
-
Object Creation Operator:
- The object creation operator, also known as "new", is used for creating an object from a class or an array. Syntax: new identifier (argument list) This calls the constructor and allocates memory for an object. For instance: String s = new String("Scaler");.
-
Relational Operator:
- Used for comparing operands. Operators include:
- Greater than: operand1 > operand2 returns true if operand1 is greater than operand2.
- Greater than or equal to: operand1 >= operand2 returns true if operand1 is greater than or equal to operand2.
- Less than: operand1 < operand2 returns true if operand1 is less than operand2.
- Less than or equal to: operand1 <= operand2 returns true if operand1 is less than or equal to operand2.
- Type checking: operand1 instanceof operand2 returns true if operand1 is an instance of the class represented by operand2.
- Used for comparing operands. Operators include:
-
Shift Operator:
- Used to shift the bits of integers.
- Left shift: operand1 << operand2 shifts the bits of operand1 to the left. For int (32-bit), the five low-order bits of operand2 are used, and for long (64-bit), six low-order bits are used.
- Right shift:
- Signed: operand1 >> operand2 shifts the bits of operand1 to the right, preserving the sign.
- Unsigned: operand1 >>> operand2 shifts the bits of operand1 to the right and fills with zero bits on the left.
- Used to shift the bits of integers.
-
Unary Minus/Plus Operator:
- Java supports unary (+) and unary (-) operators. The unary minus operator negates its operand; for instance, -(-3) returns 3. The unary plus operator returns an unchanged operand; for instance, +3 returns 3, and +-3 returns -3.
Operator Precedence
It is used for determining the order in which the operators in the expression are evaluated. For example:
Here two operand shares a command operand which is 4 in this case. The operator with the highest precedence is executed first (which in this case is *) or the expression is evaluated from left to right.
Associativity Rules
It specifies the order in which the expression is executed which is generally from left to right. There are 3 types of associativity:
- Left-associative: operators are accessed from left to right.
- Right-associative: operators are accessed from right to left.
- No associativity: operators are executed in random order.
Types of Expressions in Java
Infix Expression
It is an expression in which the operators are used between operands.
Postfix Expression
It is an expression in which operators are used after operands.
Prefix Expression
It is an expression in which operators are used before an operand.
Examples
Expressions That Produce a Value
Expressions That Assign a Variable
Explanation
In the "Expressions That Assign a Variable" section:
- The assignment operator (=) is used to assign values to variables.
- The value on the right side of the = operator is an expression that gets evaluated, and its result is assigned to the variable on the left side.
- The corrected program calculates the number of seconds in a day and, if calculateWeek is true, calculates and displays the number of seconds in a week.
Java Expression with No Results
Let's say you have the following method:
If you were to call this method:
This is an expression that produces no usable result. The method returns void, meaning it doesn't produce a value that you can assign to a variable, use in an arithmetic operation, or do anything else with.
Explanation:
In the above example, the displayMessage() method call is an expression that produces no result. It performs an action (printing a message) but doesn't give back any value that can be stored or further manipulated.
Java Statements
A statement in java is a command that is to be executed and can include one or more expressions. Types of statements in java:
- Expression statement that changes the value of variables, method calls, and objects.
- Declaration statement is used for declaring variables.
- Control flow statements are used for determining the order in which the statements are executed. Example:
Difference between Statements and Expressions in Java
Statements | Expressions |
---|---|
It is used for performing actions. | Is it used for evaluating a value. |
It cannot be made up by using other statements. | It can be made using various expressions. |
Statements are of type void in most programming languages. | There are types of expression in every programming language. |
Statements are pre-defined in most programming languages. | These are usually defined by the users. |
These are evaluated based on the order or sequence of statements. | These are evaluated based on rules of operations. |
Learn More
Conclusion
-
Expressions in Java:
- An expression in Java is a combination of variables, operators, and method calls constructed according to the language's syntax, which evaluates to a single value.
- Simple expressions in Java have a type, such as 43 being a literal of the int type, "java" representing a String literal, and 32L signifying a long literal.
- Compound expressions combine multiple simple expressions, typically using operators.
-
Types of Operators:
- Operators in Java play a crucial role in forming compound expressions. Common operators include additive, bitwise, conditional, logical, multiplicative, and relational operators, among others.
-
Operator Precedence and Associativity:
- Operator precedence defines the order in which different operators are evaluated in an expression. For instance, multiplication takes precedence over addition.
- Associativity rules specify how operators with the same precedence level are grouped in the absence of parentheses, with most operators having left-to-right associativity.
-
Java Statements:
- A statement in Java represents a command or set of commands for the JVM to execute. Statements can include expressions and span constructs like assignments, method calls, and control flow directives.
- Examples of Java statements include declaration statements (e.g., int number;), expression statements (e.g., number = 4;), and control flow statements (e.g., an if statement).
-
Difference between Statements and Expressions in Java:
- While expressions evaluate to a single value, statements perform actions and don't necessarily produce a value.
- Expressions can be combined to form larger expressions, but statements in Java are discrete units of execution.
- Methods in Java that don't return a value have a return type of void, but it's a misconception to say that all statements are of type void.