Builtin MiddleWar in ExpressJS
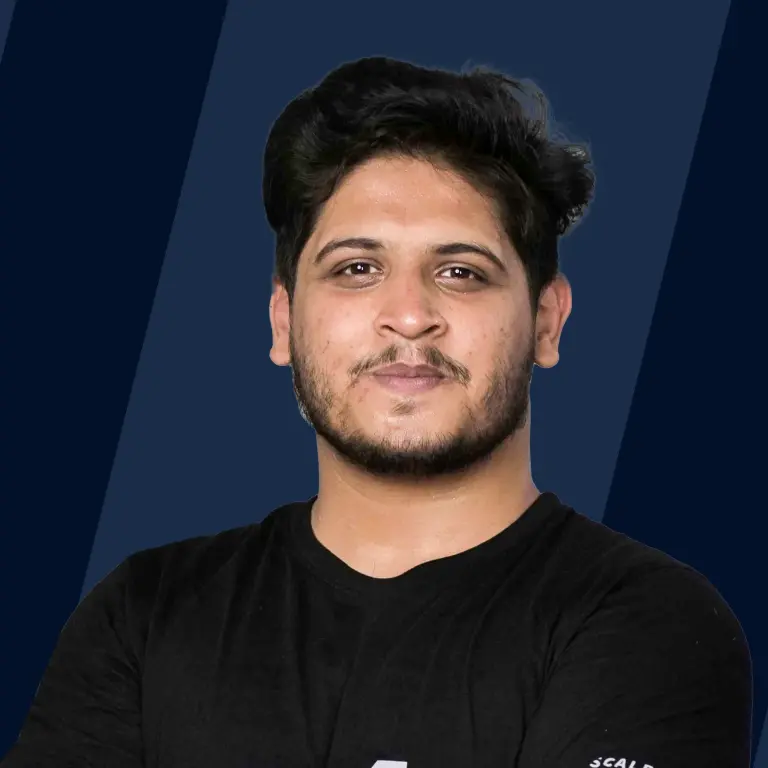
Overview
Imagine you're totally burned out after work and finally decide to go on that trip you've been planning with your friends for ages. Now, since it's a road trip you'd want to stop at multiple places along your journey. It might be a gas station to refuel your vehicle, a toll booth, or even a hotel to stay in for the night. Now, you can think of each stop as a middleware function that intercepts your journey and performs a specific task before you can go on to your next stop.
ExpressJS MiddleWare is a function that exists between the client and the server and is responsible for modifying the request or response objects, or performing some other action before the request is handled by the server or after the response is generated.
Introduction to UsiMiddlewareare
Express is a web framework for routing and middleware with only the following basic functionality: In essence, an Express program is a collection of middleware function calls. In the request-response cycle of an application, express middleware functions are functions that have access to the request object (req), the answer object (res), and the subsequent express middleware function. A variable with the name next is frequently used to identify the next middleware procedure.
The following activities can be carried out by express middleware functions:
- Put any code to use.
- Modify the objects representing the request and the response.
- Stop the loop of requests and responses* Invoke the middleware procedure that is next on the stack.
- The current express middleware function must execute next() to transfer control to the following middleware function if it does not stop the request-response cycle. If not, the proposal will be ignored.
An Express application can use the following types of middleware:
- Application-level middleware
- Router-level middleware
- Error-handling middleware
- Built-in middleware
- Third-party middleware
Application Level Middleware
To attach express middleware that operates at the application level to a specific app object instance, you can utilize the app.use() and app.METHOD() functions. The METHOD parameter should be in lowercase and correspond to the HTTP method (such as GET, PUT, or POST) that the middleware function handles.
Example 1
The express middleware method in this example doesn't have a mount path. Every time the app gets a request, the function is run.
Here, the app object represents a server, app.use() method is used to set up the middleware function
Using this line the middleware function logs in the current time to the console
What makes this middleware function intriguing is that it lacks a mount path, meaning that it's triggered by every HTTP request the server receives. This can be beneficial when implementing features that need to apply to all requests, like authentication or logging. However, it's essential to keep in mind that if needed, middleware functions can also be confined to a specific set of paths using a mount path.
Example 2
This example shows a middleware function mounted on the /user/:id path. which means that the express js middleware function will only be called for HTTP requests that match this route pattern
The middleware function logs the HTTP request method to the console using
This kind of scoped middleware function can help add features that are unique to particular routes, like input validation or authentication. You can make sure that the expressjs middleware function is only used when it is required and not for each HTTP request to the server by using a mount path to scope the function to particular routes.
Furthermore, you can alter code like this
This would show a route and its handler function (middleware system).
Example 3
Here is an illustration of how to use a mount path to install several express middleware functions at a mount point. It displays an example of a middleware sub-stack that outputs request information for any kind of HTTP request to the /user/:id path
Example 4
Route handlers allow you to define multiple routes for a path. The example below defines two routes for GET requests to the /user/:id path. The request-response loop is ended by the first route, so the second route won't cause any problems but will never be used.
It shows an express middleware sub-stack that handles GET requests to the /user/:id path
Call next('route') to transfer control to the next route in a router middleware stack to skip the remaining middleware operations.
NOTE: only middleware methods that were loaded using the app.METHOD() or router.METHOD() functions will support next('route').
Example 5
This example shows an array with a middleware sub-stack that handles GET requests to the /user/:id path
Router Level Middleware
Router-level middleware works similarly to application-level middleware, except being connected to an instance of express.Router()
Use the router to load middleware at the gateway level. the router.METHOD() and use() methods.
The accompanying sample code, which makes use of router-level middleware, simulates the middleware system shown above for application-level middleware:
next('router') can be used to pass control back out of the router instance to skip the rest of the router’s middleware functions
Example 1
This example shows a middleware sub-stack that handles GET requests to the /user/:id path
If the incoming request does not contain a x-auth header, the middleware function instantly transfers control to the following middleware function, which response with a 401 (Unauthorized) response.
The code then creates a route for the router that replies with a straightforward greeting to GET queries to /user/:id
The code then configures the application so that it will use the router for any requests that fit the /admin path. As a result, any requests for, say, /admin/user/123 will first go through the middleware code set up for the router. The request will go to the path designated for /user/:id and return the welcome message if the "x-auth" header is present. The middleware function will instantly transfer control to the final middleware function, which responds with a 401 (Unauthorized) response if the request does not contain a "x-auth" header
Error Handling Middleware
Expresjs middleware for managing errors always requires four arguments. For it to be recognized as an error-handling middleware method, you must supply four arguments. You must identify the next object even if you won't be using it to uphold the signature. If not, the subsequent object will be seen as standard middleware and will be unable to manage errors.
Error-handling functions should be defined with four arguments rather than three, using the signature (err, req, res, next), as with other middleware functions
The function takes four arguments:
- "err" represents the error object that was thrown or passed to "next" by another middleware function.
- "req" represents the incoming request object.
- "res" represents the response object that will be sent back to the client.
- "next" represents the next middleware function in the chain (although it is not used in this function).
When an error is thrown or passed to next, this expressjs middleware function will log the error stack to the console using console. error, and then send a 500 (Internal Server Error) response back to the client with the message "Something broke!"
Built In Middleware
Express includes the following software features by default:
- HTML files, images, and other static elements are served by express. static
- JSON payloads from inbound requests are parsed by express.json
- The URL-encoded payloads in inbound requests are parsed by express. urlencoded
We'll elaborate on these further in this article
Third-Party Middleware
Third-party middleware can be used in an Express application to add additional functionality or features that are not provided by the core framework
The installation and loading of the cookie-parsing middleware function cookie-parser are demonstrated in the sample below.
This example shows the working of third-party middleware, using which a cookie-parser package, a middleware for parsing cookies in Express.js applications, is installed via npm. Express is then imported, and an instance of an Express application is created.
Any incoming cookie headers are parsed by this middleware, which then adds key-value pairs representing the cookie name and value to the req.cookies object. Making it simpler for Express routes and middleware functions to read and manipulate cookies.
Built-In Middleware Functions
For common tasks in web applications, Express.js offers a set of integrated middleware functions. The following are some of the most popular built-in middleware features:
-
express.static: This middleware feature is used to deliver static files from a server directory, including images, CSS, and JavaScript files. It accepts as an argument the root directory from which to serve files.
-
express.json: JSON payloads in HTTP requests are parsed using this middleware function. It adds the parsed info to the req.body object.
-
express.urlencoded: The HTTP requests' inbound URL-encoded payloads are decoded using this middleware method. It adds the parsed info to the req.body object.
-
express.Router: Create modular routes that can be mounted to various paths in your program using this middleware function.
-
express.cookieParser: To process cookies in HTTP requests, middleware is used. The decoded cookies are added to the req.cookies object.
-
express.session: In your program, user sessions are created and managed using this middleware function.
Let's discuss some of them in detail
express.static
The express.static() function is a built-in middleware function in Express. It serves static files and is based on serve-static. Syntax:
Parameters: The root parameter describes the root directory from which to serve static assets.
Return Value: It returns an Object.
Explanation Files that clients receive directly from the server are known as static files. Make a new subfolder called public. By default, Express forbids serving inactive files. Use the following built-in middleware to activate it.
Note:
-
Since Express searches for files relative to the static directory, the URL does not contain the name of the static directory.
-
As your public directory is now the root route, all static files you load will consider public as the root. To confirm that everything is working as expected, add an image file (e.g., "testimage.jpg") to your new public directory and create a new view in your views. Then, include the image file in your view.
Multiple Static Directories
We can also set multiple static assets directories using the following program −
Virtual Path Prefix
To serve static files, we can also provide a path prefix. For instance, you must add the following code to your index.js file if you want to provide a route prefix like /static. −
Now, let's see an example Example Create a file named index.js and use the following code
The callback code is executed whenever a GET request is sent to the root endpoint ('/'). This function creates a view using the home.ejs template file and the res.render method. Data passed to the ejs template engine as an argument is used to create dynamic HTML content.
Now, create a home.ejs file in views folder with the following code:
express.json
express.json() is a built-in middleware function in Express. This method is used to parse the incoming requests with JSON payloads and is based on the body parser.
This technique provides middleware that only parses JSON and examines requests where the content-type header corresponds to the type option.
Syntax:
Parameters: The options parameter have various property like inflate, limit, type, etc.
Return Value: It returns an Object.
Now let's take a look at an example
Example 1:
Create a file named index.js
On port 3000 an express js server is created to parse the JSON payloads from HTTP POST requests using the express.json middleware method
The callback code is executed whenever a POST request is sent to the root endpoint ('/'). This function uses the console.log command to record the value of the name attribute of the parsed JSON data from the request body (req.body.name). The parsed JSON data from the request body is contained in the req.body object, which is filled out by the express.json middleware method.
Then you will see the following output on your console:
express.urlencoded
Express.js comes with a built-in wrapper called express.urlencoded(). This approach, which is built on the body parser, aims to parse incoming requests with urlencoded payloads.
The middleware that decodes all of the urlencoded bodies is returned by this function.
Syntax:
Parameter: The options parameter contains various properties like extended, inflate, limit, veri,fy etc.
Return Value: It returns an Object.
Let's see an example to understand better
Example 1:
Filename: index.js
On port 3000 an express js server is created to decode inbound URL-encoded form data from HTTP POST requests
The output from the console.log statement in your code would look like this:
{ name: 'ScalarTopics' }
Conclusion
- Express JS is a function that is responsible for modifying the request or response objects, or performing some other action before the request is handled by the server or after it's generated
- The current express middleware function must execute next() in order to transfer control to the following middleware function if it does not stop the request-response cycle. If not, the proposal will be ignored.
- A sub-stack of the expressjs middleware system can be built at a mount point by loading several middleware functions simultaneously
- Error-handling functions should be defined with four arguments rather than three, as with other middleware functions
- Third-party middleware can be used in an Express application to add additional functionality or features that are not provided by the core framework