Factors of a Number in C
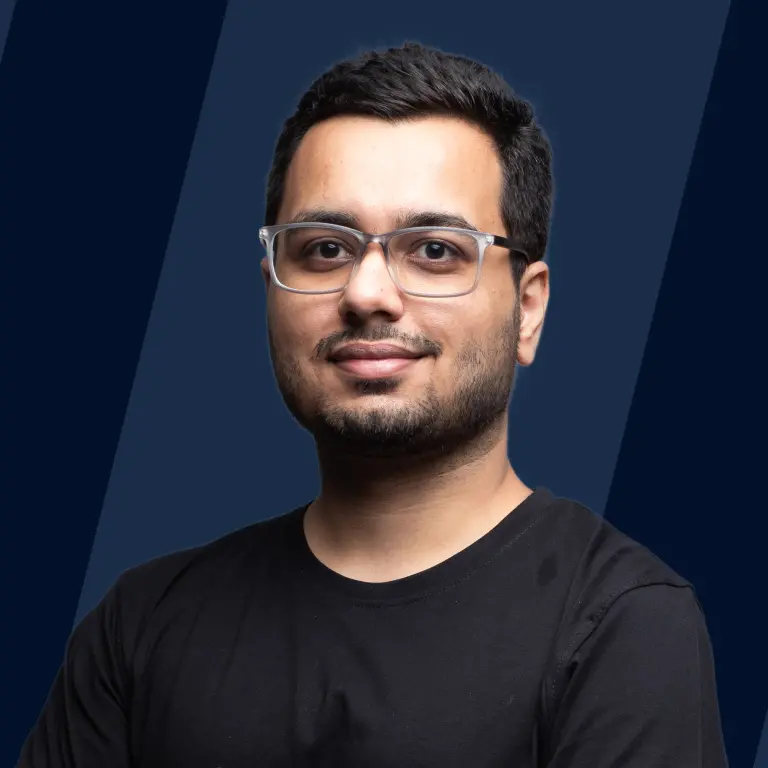
Overview
The factors of the number are the values that completely divide the given number i.e give the remainder zero. In this article, we will see how to find factors of a number in C programming language using various techniques.
Factors of a Positive Integer
The factors of given numbers are the numbers that perfectly divide the given number or we can say give zero remainders when the given number is divided by them.
For example, factors of number 20 are -
1, 2, 4, 5, 10, and 20 are the factors of the number 20. All these numbers perfectly divide 20 without giving any remainder.
C Program to Find Factors of a Number Using For Loop
The following coding example shows the way to find the factors of a number in c programming using for loop.
Code:
Output:
In the above code, we used the for loop to iterate from the 1 to the given input and we used the if statement inside the for block where we check whether the number perfectly divides the entered number or not.
For example, in the above code, we entered the 20 as input so in for loop iterates from 1 to 20, and for each number the if condition checks if the number perfectly divides the given number or not. If the number is a factor then it gets printed, otherwise, we move to the next number.
C Program to Find Factors of a Number Using While Loop
The following coding example shows the way to find the factors of a number in c programming using a while loop.
Code:
Output:
In the above code, we used the while loop to find the factors of a number. The logic remains the same we check each number from 1 to the given input and if it is perfectly divisible we print it otherwise we increment the number and again check for the factor until we reach the last iteration of the while loop.
C Program to Find Factors of a Number Using the do-while Loop
The following coding example shows the way to find the factors of a number in c programming using a do-while loop.
Code:
Output:
In the above code, we used the do-while loop to find the factors of a given number. When we use the do-while loop 1 iteration needs to perform strictly as we check the condition at the end of the loop, otherwise, it is based on the same logic where we find the factors in the loop by iterating from 1 to entered number.
C Program to Find Factors of a Number Using Functions
The following coding example shows the way to find the factors of a number in c programming using functions.
Code:
Output:
There are various ways and logic to find the factors using the function in c programming. In the above code, we wrote the function in which we implemented the logic using the for loop and called the printFactors function from the main. When the function runs it prints the numbers which perfectly divide the entered number as we did earlier in the above programs.
C Program to Find Factorial of a Number Using Pointers
The following coding example shows the way to find the factors of a number in c programming using pointers.
Code:
Output:
In the above code, we used the pointer ptr in which we stored the address of the number variable. We pass the pointer as an argument at the time of the function call. Then we use this reference of a number in the function printFactors. Here we used the for loop to iterate over the numbers and if condition to check whether the number perfectly divides the entered number or not.
C Program to Find Factors of a Number using recursion
The following coding example shows the way to find the factors of a number in c programming using recursion.
Code:
Output:
In the above code, we used the recursion technique to find the factors of a given number. A recursive function is a function that calls itself.
Here in the above example, we defined the printFactor function which works as a recursive function. We declared two parameters in the function one is the entered number and the other is the number that we are maintaining (i) for recursion which ranges from 0 to entered number.
With this logic here we end the recursion with the help of the base case when the maintained number (i) becomes greater than the entered number. Other than that in the function definition we check whether the i perfectly divides the entered number or not if it perfectly divides we print it, otherwise, we make a recursive call by incrementing the i.
Conclusion
- The numbers which perfectly divide the given value are known as the factors of that value.
- We can find the factors of a number in c programming using various techniques.
- In for loop, while loop, and do-while loop we iterate from 1 to a given number to find the factors of a given number.
- When we use a function, we pass the given number as an argument to find the factors of the given number, and the entire logic is present in the function definition.
- We can pass the reference of a number with the use of a pointer which we can then pass into the function to find the factors of a given number.
- Recursion is also one of the ways to find the factors of a given number.