What Values Does JavaScript Consider Falsy?
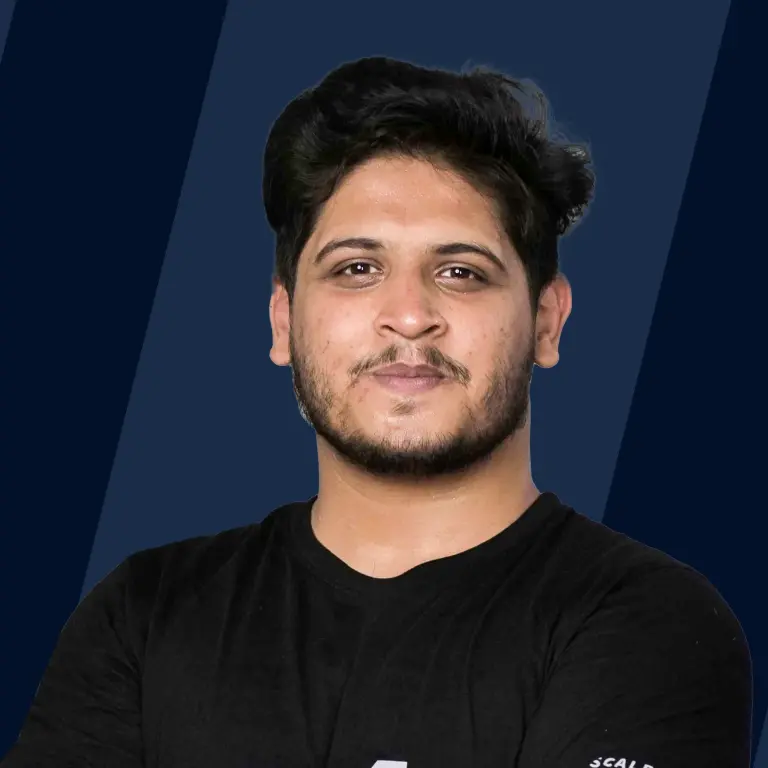
In addition to the boolean value false, javascript also considers the other five values as equivalent to FALSE and they are, undefined, NaN, null, 0, and ""(empty string).
The number 0 in any data type such as int(0), float(0.0), BigInt(0n), hexadecimal(0x00), negatives(-0) is considered as a falsy value.
BigInt is a built-in object that is used to represent numbers that are greater than . The BigInt numbers are represented by an n at the end of the number.
For example, 25n, 5n, 213123n, etc...
Description
Falsy values are values that are considered as false by the online javascript compiler. These false values are often used to evaluate different conditions in the program. These falsy values return false with the Boolean() constructor.
A Complete List of JavaScript Falsy Values
The following are the javascript falsy values :
Falsy Values | Description |
---|---|
false | The value of Boolean object |
0, 0,0,0x00 | Zero of Number object |
-0, -0.0, -0x00 | Zero value of Number object |
0n | Zero value of BigInt object |
"",'',`` | Empty String of String object |
null | The keyword for empty variables |
undefined | The keyword for undefined variables |
NaN | The keyword for variables that are not a number |
document.all | Falsy objects are inaccessible by the document.all method |
Code Example of Falsy Values
The Boolean object is used to represent the boolean data type and the parameter passed into the Boolean() constructor will be converted into an object of the boolean data type.
The following examples illustrate the different use cases of the javascript falsy values,
The false and true are keywords of the data type boolean that are mostly used as flags to check conditions in a program.
Output :
The numbers(0,-0), decimal(0.0), and hexadecimal number(0x0) are all falsy values and are equivalent to false.
According to the IEEE-754 standards of javascript, all these numbers are just different representations of the 64-bit floating point value.
Code :
Output :
The javascript falsy value, BigInt zero was introduced in the ES2020 script in 2020. BigInt is a flexible data type that takes arbitrary space required to store large numbers that are not accommodated by the Number data type.
Code :
Output :
The javascript falsy value, null is the representation of a variable with an empty value. A variable can be defined with an empty value with the help of the null keyword.
Code :
Output :
The undefined keyword in javascript is assigned to any variable that was just declared without assigning of value.
Code :
Output :
The NaN keyword is used to represent a number that is not a legal number. The Number.isNaN() method is used to check if a number is a NaN. The number NaN occurs when a number is divided by another data type.
Code :
Output :
Checking for Falsy Values on Variables
The variables can be checked for falsy values under if...else statements to control the flow of the program.
Let us consider the following example,
Example :
Let us see how the variables are loosely compared using the == operator. The following code illustrates how variables are compared using the == operator,
Code :
Output :
The following case illustrates a difference in comparison between the falsy values,
Code :
Output :
Explanation :
The result is not equal because the null variable is not equal to any values other than undefined according to the standards of javascript and hence proves the execution of the above example. This is also the same for the undefined keyword.
The == operator behaves differently in the presence of the ! operator. The following example illustrates this,
Code :
Output :
Explanation :
The output results in equal because the ! operator performs a casting to the boolean data type which is then evaluated using the expression.
The == operator follows the Abstract Equality Comparison Algorithm and by this algorithm, if any of the two variables to be compared are of type boolean, then the boolean value is converted to a number before comparison. The true value is converted to 1 and the false value is converted to +0. The null type is not equal to 1 or +0, but the +null will be equal to false(+0) and will return true.
The NaN value is not equal to another other value in comparison and is not even equal to itself. For example the following code prints not equal,
Code :
Output :
General Examples
The following example illustrates the use of such falsy values,
Code :
Explanation :
In the above example, the javascript falsy value is used as a condition in the while() loop, and the falsy value is inverted using the ! operator when a certain condition is met to end the loop. Many use cases of the falsy variable are to handle unseen situations in which we may encounter an empty value for a variable or zero or an empty string.
The javascript falsy values are also used to check if an object has a certain property. The following example illustrates this,
Example :
The presence of the property unavailable_property in the Array object is checked using the falsy values.
Output :
We can also find the same results using the following code,
Code :
Output :
Explanation :
Since length is a property that belongs to the Array object, it returns true. The method discussed before the current example is more suitable to check the presence of a method in an object because the current example can't be used to check a method or data member that has the value of 0 or "".
Based on the above concepts, the following code can be made to run on the console of a browser to find that if the browser has geolocation support,
A Note on Strict Equality
The strict equality operator or the === operator will not make any type casting to the values that are compared and will return false if the data types of the compared values are different.
The following example illustrates how the === operator works,
Code :
Output :
Explanation :
Since the data types of the values compared are different, the strict equality operator returns false, and therefore the else part of the code is executed.
Even using the strict equality operator it is important to note that NaN is not equal to NaN,
Examples with Arrays
The array object will never be a falsy value, even empty arrays will return true when passed as a parameter to the Boolean() function. The following example shows this,
Output :
The array object acts differently when compared with the false value and the following code illustrates this,
Code :
Output :
Explanation :
The reason for this is due to the Abstract Equality Algorithm followed by the == operator. By the algorithm, the following steps occur,
- The value false is converted to 0.
- By the rule, if the value is to be compared in our object and number, then the object is converted to primitive form. The empty array([]) is converted to an empty string('').
- Then the empty string is again converted to 0.
Hence, now both sides are equal and this results in the above output.
Caveat
The typeof operator is used to find the data type of the variable and can be used to check for undefined variables, as when checking for the availability of a property or data member of an object as numbers and strings may behave differently due to falsy values.
The following example illustrates this,
Code :
Output :
In the above example, even though the data member name is available, the condition couldn't evaluate it, because of its falsy value. The above error can be rectified by using the typeof operator,
Code :
Explanation :
The above code only checks the availability of the name data member and doesn't consider the falsy values.
The Logical AND Operator(&&)
The AND(&&) operator is used to evaluate two conditions at the same time. Falsy values in javascript can be used along with the AND operator to perform several functions. Let us consider the following example,
Code :
Explanation :
The above example produces no output as both test1 and test2 are fasly values. On using the AND operator, the test2 is not evaluated as the test1 is false. If the test1 is true, then the test2 is evaluated and if both are true, the if block is executed.
Conclusion
- Falsy values in javascript returns false on passing as a parameter to the Boolean() function.
- There are 6 falsy values in javascript and are used in if cases to control the flow of the program.
- These falsy values can be evaluated using loose and strict equality.
- All objects are not falsy objects as even empty objects are not undefined. Everything is defined at declaration and is never equal to false.
- The presence of a method or data member in an object can be evaluated using javascript falsy values.