FileInputStream in Java
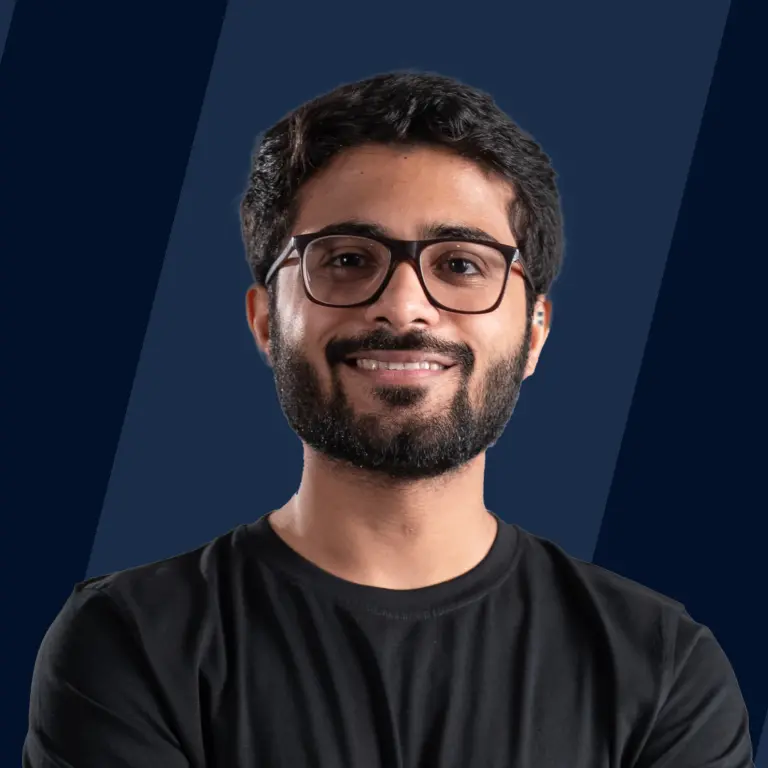
Overview
A file's input bytes are obtained through the Java FileInputStream class. It is used to read byte-oriented data, which includes image, audio, and video data. Character-stream data is also readable. However, the FileReader class is advised for reading character streams. FileInputStream class offers various method and constructor that allows reading from file. Some commonly used methods are read() is used to read characters from a file, skip() which is used to skip over, and available() to find how many bytes are remaining in the file to read.
What is FileInputStream in Java?
FileInputStream class is useful to read data from a file in the form of sequence of bytes. A file's input bytes are obtained through the Java FileInputStream class. It is used to read byte-oriented data, which includes image, audio, and video data. Character-stream data is also readable. However, the FileReader class is advised for reading character streams.
Constructors of FileInputStream in Java
1.FileInputStream(File file): Creates a file stream for input that will be used to read from the provided File object.
2. FileInputStream(FileDescriptor fdobj)
3. FileInputStream(String name): A file with the supplied name is read from by creating an input file stream.
Methods of FileInputStream in Java
Methods | Action | Syntax |
---|---|---|
available() | Estimates the number of bytes in this input stream that can still be read (or skipped over). | new FileInputStream("file1.txt").available() |
close() | Closes fileInput Stream, and any system resources connected to the stream are released. | new FileInputStream("file1.txt").close() |
finalize() | This method makes sure that when there are no more references to the file input stream, the close method is called. | new FileInputStream("file1.txt").finalize() |
getChannel() | the unique FileChannel object connected to this file input stream is returned. | new FileInputStream("file1.txt").getChannel() |
getFD() | provides the link to the actual file in the file system that this FileInputStream is using, represented by the FileDescriptor object. | new FileInputStream("file1.txt").getFD() |
read() | Reads a byte of data from this input stream | new FileInputStream("file1.txt").read() |
read(byte[] b) | This method receives data from this input stream into an array of bytes, up to b.length bytes in total. | new FileInputStream("file1.txt").read(byte[] b) |
read(byte[] b, int off, int len) | This method receives data from this input stream into an array of bytes, up to b.length bytes in total. | new FileInputStream("file1.txt").read(byte[] b,int off, int len) |
skip() | skips n bytes of data from the input stream and discards them. | new FileInputStream("file1.txt").skip() |
Basic Steps to be Followed to use FileInputStream Class in Java for Reading Data from a File.
The ultimate purpose of FileInputClass is to read data from files using FileInputStream, thus whenever we use these methods, we generically follow these steps to accomplish so.
Step1
As illustrated below, by attaching a file to a FileInputStream, we may read data from the file and do the following:
Step2
Now, as indicated below, we should read data from the FileInputStream using read() method in order to read data from the file:
Step3
There can be two possible condition after calling read() method
- When there is no more data available to read further, the read() method returns -1;
- If read() doesnt return -1 then we can do what we want with data like printing data
Example of FileInput Stream
In this example we are going to use methods and constructor that we have discussed above.
Output:
Explanation:
In the above code, we have First created an Object of FileInputStream and attached input_file.txt to FileInputStream. Then printed how many bytes are available to read from a file that is 100 and printed FileChannel and FileDescriptor object. After that, we skipped 10 bytes and now available bytes are 90 bytes. Then to print all characters of the file we have used and while loop that will run until we haven't reached the end of the file and will print each character.
Conclusion
- A file's input bytes are obtained through the Java FileInputStream class.
- It is used to read byte-oriented data, which includes image, audio, and video data. Character-stream data is also readable.
- However, the FileReader class is advised for reading character streams.
- FileInputStream class offeres various method and constructor that allows to read from file.
- Some of commonly use method are read() that is used to read character from file,skip() that is used to skip over and available() to find how many bytes are remaining in file to read.