FileOutputStream in Java
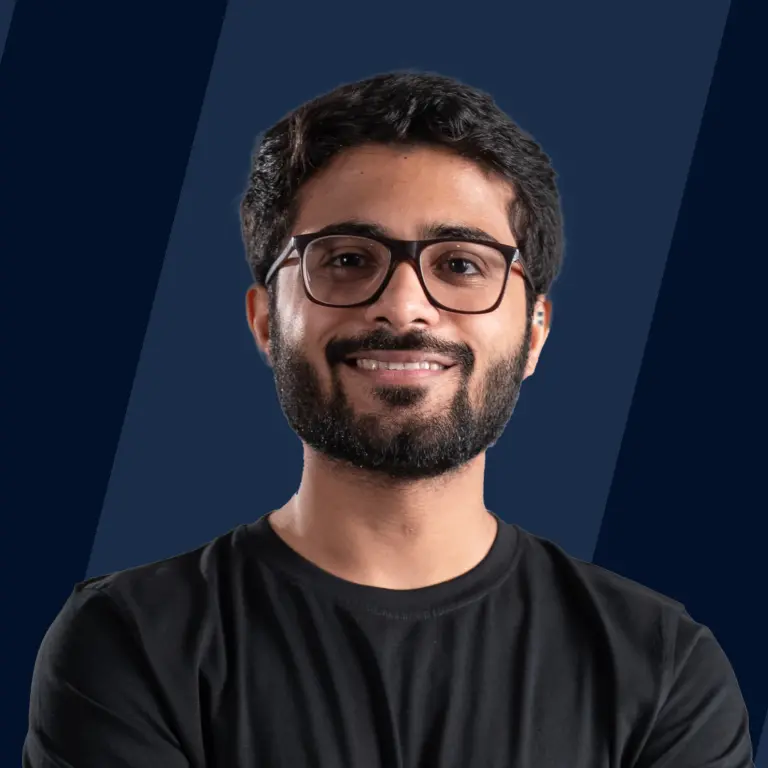
FileOutputStream, a subclass of OutputStream, facilitates writing raw byte streams to files or storing data. Primarily used for writing primitive values, it supports both byte-oriented and character-oriented data. However, FileWriter is generally preferred for character-oriented data in Java. FileOutputStream is integral for efficiently writing various types of data to files in Java programming. Learn more about files in Java here
How to Store Data in Files
The above-mentioned graphic demonstrates how data is saved in RAM before to execution of the Java program. Let's say we wish to access the variable data that is currently being kept in RAM and transfer it to a file on our hard drive. So, we'll make an OutputStream object in RAM that points to a file referencing a hard drive.
The information from the RAM variable data file will now be transmitted to the referenced file (an object of the output stream), where it will then be saved in the hard disk's file.
Hierarchy of FileOutputStream
You may manipulate binary files by using the FileInputStream and FileOutputStream classes by following the instructions in this Java File IO tutorial.
FileInputStream and FileOutputStream in Java are byte streams in the Java programming language that read and write data in binary format, which consists of exactly 8-bit bytes. They are descendants of the super types of all byte streams, the abstract classes InputStream and OutputStream.
You can better comprehend the byte stream classes' API structure by looking at the class diagram below:
As you can see, these classes support the AutoCloseable interface, allowing us to use the try-with-resources structure to automatically close the streams.
Constructors of FileOutputStream in java
FileOutputStream(File file)
Creates a file output stream to write to the file represented by the specified File object.
FileOutputStream( File file, boolean append)
A file output stream object is created and represented by the given file object.
FileOutputStream(FileDescripter fdobj)
It establishes a connection to the supplied file descriptor, which stands in for an already-existing connection with the actual file in the file system, to create a file output stream for writing to it
FileOutputStream(String name)
It creates a file output stream object to write to the specified file with the given name
FileOutputStream(String name, boolean append)
It creates a file output object with the supplied name to write to the file
Methods of FileOutputStream in Java
Let's now look at some methods of FileOutputStream in Java
Method | Description |
---|---|
protected void finalize() | It is used to clean up the connection with the file output stream. |
void write(byte[] ary) | It is used to write ary.length bytes from the byte array to the file output stream. |
void write(byte[] ary, int off, int len) | It is used to write len bytes from the byte array starting at offset off to the file output stream. |
void write(int b) | It is used to write the specified byte to the file output stream. |
FileChannel getChannel() | It is used to return the file channel object associated with the file output stream. |
FileDescriptor getFD() | It is used to return the file descriptor associated with the stream. |
void close() | It is used to close the file output stream. |
Let's discuss these methods briefly
1. protected void finalize(): When there are no more references to this file output stream, the java.io.FileOutputStream.finalize() function ensures that the close method is invoked and closes the connection to the file
Before an object is discarded or destroyed by the garbage collector, all resources required by it must be released using Java's finalise function. In Java, finalise() is a method, not a reserved word. Once the finalise() method has completed its cleanup task, the garbage collector promptly destroys the Java object. Only one call to the finalise() method is allowed per object by the Java Virtual Machine (JVM)
A flag indicating that an object has been finalized and won't be finalized again is set in the object header by JVM once it has been finalized. JVM ignores attempts by a user to use the finalise() function twice for the same object.
Learn more about finalize() here
Following is the declaration for the java.io.FileOutputStream.finalize() method
Example
Let's see an implementation of this method in Java
Suppose we have the following text in a file called c:/test.txt The input for our example application will be this file.
ABCDE
Let's compile and run the aforementioned program, which will result in the output shown below.
Stream is closed successfully
2. write() Method
- write() - This method writes the single byte to the file output stream
- write(byte[] array) - It writes the bytes from the specified array to the output stream
- write(byte[] array, int start, int length) - It writes the number of bytes equal to length to the output stream from an array starting from the position start
Following is the declaration for the java.io.FileOutputStream.write(byte[] buffer, int offset, int len) method in Java
Here in the parameters,
- buffer − The source buffer.
- offset − The start offset in the data.
- len − The number of bytes we want to write.
Let's now see an example of FileOutputStream to write data to a File in Java
We create a file output stream named as output The file output stream is evidently linked with the file output.txt
For writing data to the file, we've used the write() method here in this example
Here, the moment we run the program, the output.txt file is filled with the following content
Tcontent inside the text file displayed here
==getBytes() method used in the program converts a string into an array of bytes==
3. FileOutputStream.getChannel() The java.io.FileOutputStream.getChannel() method returns the unique FileChannel object associated with this file output stream
Following is the declaration for the java.io.FileOutputStream.getChannel() method
The following example shows the usage of the java.io.FileOutputStream.getChannel() method
Output:
4. FileOutputStream.getFD(): The java.io.FileOutputStream.getFD() method returns the file descriptor associated with this stream.
declaration for java.io.FileOutputStream.getFD() method in Java is provided
Let's see an implementation of java.io.FileOutputStream.getFD() method in Java
Output:
5. FileOutputStream.close(): The java.io.FileOutputStream.close() closes this file output stream and releases any system resources associated with this stream.
Let's see how can we declare the java.io.FileOutputStream.close() method
Let's see an example that implements the java.io.FileOutputStream.close() method.
Say we have a text file c:/test.txt which will be used as input for our example program
Output
While we're at it let's also briefly discuss some Methods declared in the OutputStream class
Method | Description |
---|---|
flush() | This method forces any data present in the output stream to be written to the target (disk) |
nullOutputStream() | This method returns a new OutputStream discarding all bytes. The returned stream is initially open. |
Basic Steps to be Followed While Writing Data to a File Using the FileOutputStream in Java.
Steps to writing data to a file using FileOutputStream:
- First, attach a file path to a FileOutputStream as shown here:
This will enable us to write data to the file
- Then, to write data to the file, we should write data using the FileOutputStream as,
- Then we should call the close() method to close the fout file.
Let's discuss these methods of FileOutputStream to in detail to understand better
The FileOutputStream class provides implementations for different methods present in the OutputStream class
Examples of FileInputStream in Java
Example 1: Using FileOutputStream Object for Writing Data
We need to import the java.io package to use the FileOutputStream class.
The data (i.e the string TATA will be transferred to file)
Output:
myfile.txt file is created and the text TATA is saved in the file
Example 2: Java FileOutputStream Specifying Encoding
The fact that the FileWriter class uses the default encoding and prevents us from explicitly defining the encoding is a big disadvantage of this Java convenience class for writing character files. If we need to set the encoding, use OutputStreamWriter and FileOutputStream
Using an OutputStreamWriter, we write text to a file. The charset to be used is the second parameter
Output
Example 3: Java FileOutputStream Append to File
FileOutputStream facilitates in appending data to a file Logging is another use of this method
In the above program in Java, we append data to a file using FileOutputStream
The purpose of the FileOutputStream is to write streams of unprocessed bytes, such as image data. Consider using FileWriter to write streams of characters
The following code in Java helps to append text to a file in the above example:
The second parameter of FileOutputStream indicates appending to the file
The FileOutputStream constructor here in the above example, accepts a boolean that is supposed to be set to true to mark that we want to append data to an existing file.
Conclusion
- FileOutputStream is an outputstream for writing data/streams of raw bytes to file or storing data to file.
- write() - This method writes a single byte to the file output stream
- The java.io.FileOutputStream.close() closes this file output stream and releases any system resources associated with this stream.
- The FileOutputStream class provides implementations for different methods present in the OutputStream class
- An identified space that can be utilized to store relevant data is called a file.