JavaScript Array find() Method
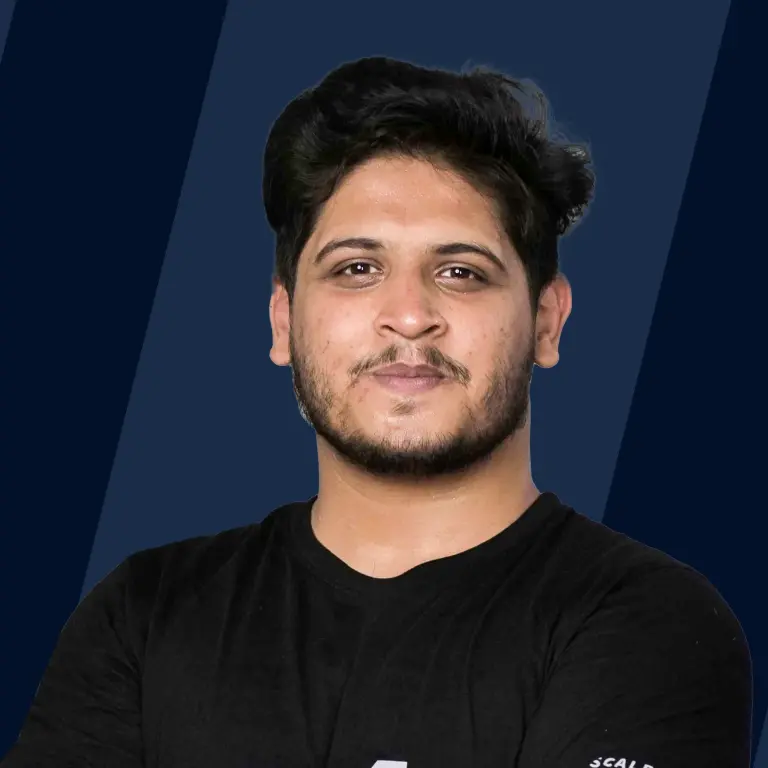
The find method in JavaScript calls the function parameter for each index of the array. If the element satisfies the test function then the find method stops its execution and returns the element. Otherwise, if no element of the array satisfies the test function then the method returns undefined. Since the function is called for each index of the array, so the function will be called even for the unassigned values.
Syntax of array.find in javascript
Using Arrow Function
Using Inline Function
Parameters of find method in javascript
Function: This is the function that will be executed for each index of the array. This function is executed with the following arguments,
Current Value: This is the current element of the Array.
Index: This is the index of the current element of the Array.
Arr: This is the array on which the find() method was called.
ThisValue: This object is used as this keyword inside the function parameter. This is an optional parameter and by default, it is set as undefined.
Return Value of array.find in javascript
Returns the first element that meets the provided test function. If no element meets the test function, undefined is returned by the find method in JavaScript.
Example
Let's have an example to find the first number in the array greater than 0.
Since we have to check whether the element is greater than 0, there is no need for the index and arr parameters.
Output:
find in JavaScript returns undefined if none of the Array elements meets the test function. Let's have an example for this case,
Output:
Note: The find in JavaScript does not alter the array, but the function passed can alter the array.
More Examples
Find an Object in an Array by One of Its Properties
Let's have an example of finding an object in the array using one of its properties. Our array will store the student details, such as name and roll number, and we have to find the object with the given roll number.
Output:
Find a Prime Number in an Array
Before moving to the program, let's see how we can check whether a number is prime. A prime number is a number that is divisible by 1 and the number itself; hence, we will loop over the numbers [2,element-1], and if any of the numbers in the range completely divide the element, we will return false.
Output:
Conclusion
- The find in JavaScript returns the first element in the specified array that meets the testing condition.
- If no element meets the test function undefined is returned by find in JavaScript.
- find method in JavaScript accepts 5 parameters, function, currentValue, index, arr, thisValue.
- The find in JavaScript does not alter the array, but the function passed can alter the array.
See Also
- JavaScript Array findIndex() method
- JavaScript Array values() method
- JavaScript Array filter() method