JavaScript Array findIndex()
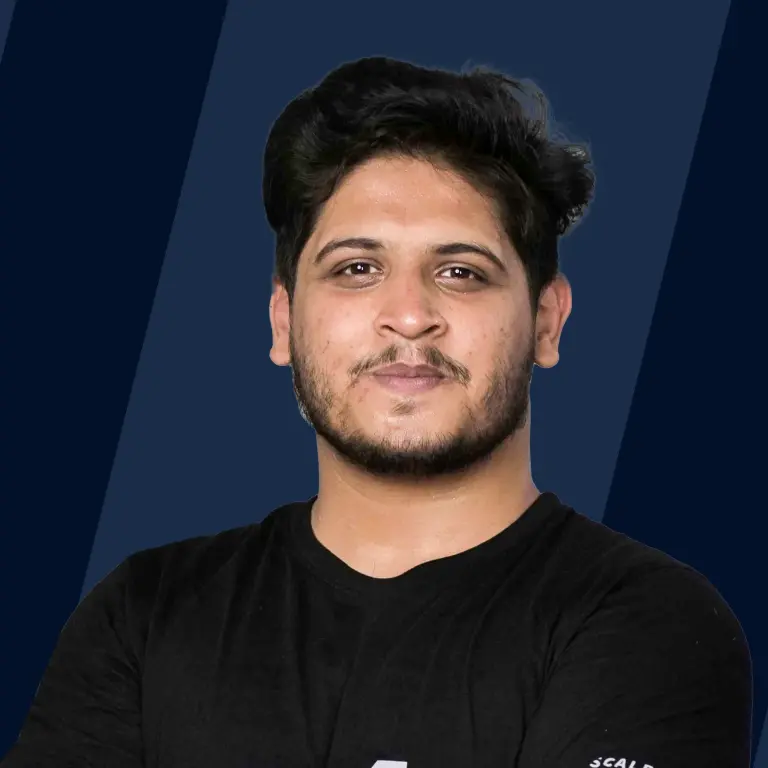
The array findindex() javascript method is used to act on objects. Using this method, we can find the first element in an array. However, to find this first array, the function should fulfil a given function for testing.
The array findindex() method in JavaScript was added to the array.prototype by the ES2015. It iterates an array and checks each element individually; that's why the time complexity always requires O(n) time. In this article, we will learn about the findindex() javascript method and see the implementation with examples and explanations of findindex javascript wherever necessary.
Syntax of findIndex() Method in JavaScript
The syntax of the array findindex() javascript method in JavaScript is as follows:
Parameters of findIndex() in JavaScript
The array findindex() javascript method parameters are as follows. Five parameters of the findindex javascript method are described below.
- function: This is the user's function in the method. This function is used to test a particular element.
- current value: This parameter is used to hold the element that is currently in the method. This is an optional parameter.
- index: This parameter is used to hold the index value of that particular current element.
- arr: This parameter holds the array object that belongs to that particular current element. It is optional.
- thisvalue: This parameter is the value passed to the function as the this value in the array findindex() javascript method. This parameter is also optional.
Return value of findIndex() Method in JavaScript
Return value: number The return value of the findindex() javascript method is as follows: This method returns an array's indexelement only if the value given to the function passes the condition. If it does not pass the test, it returns -1.
Exceptions of findIndex() in JavaScript
The following is the exception for the findindex() javascript method. This findindex Javascript method returns the index of the first element of an array, which is provided by the user only if the given array satisfies the condition given in the function.
Example of findIndex() Method in JavaScript
In the above sections, we have discussed the findindex() Javascript method, its syntax, and its parameters. Let us look at a simple and short example of how this function works. In this example, there is an array inside which some ranks are provided. We will use the findindex() Javascript method to return the occurrence of rank 7.
Output:
Explanation:
In the above example, we have declared an array named ranks inside which 6 different ranks are mentioned. We used the findindex() javascript function with rank using a dot (.) operator, and inside the method, we declared the condition (rank => rank === 7) for testing the function, and then we used the console.log function to print the output which is the index of the array where the rank 7 first occurs that is 2.
More Examples of findIndex() in JavaScript
Now, let us look at some more examples and the implementation of the findindex() method javascript using examples. All the examples are discussed with the output and explanation where ever necessary.
- Example 1. In this example of the findindex javascript method, we will use the findindex() method javascript method to get the index of the first occurrence of number 7 and also after index value 2.
Output:
Explanation: In the above example, we have declared an array named ranks inside which 6 different ranks are mentioned. We used the findindex() javascript function with rank using a dot (.) operator, and inside the method, we have declared the condition rank, index) => rank === 7 && index > 2 for testing the function. Then, we used the console.log function to print the output, which is the index of the array where the rank 7 first occurs, but that should be after the index 2. So the output is 5 as seen in the array.
- Example 2. In this example, we will use the findindex() javascript method to find the index of the first product. And the condition is that is the price of the product is greater than 1000.
Output:
Explanation: In the above example, we have declared an array of objects named products inside which there are different products with their price mentioned. Then we used the findindex() javascript function with product using a dot (.) operator, and inside the method, we declared the condition product => product.price > 1000which, which means we have to show the output for the product whose price is greater than 1000. for testing the function. Then, we used the console.log function to print the output, which is the index of the product whose price is greater than 1000.
- Example 3. In this example of the findindex javascript method, we will use the findindex() method javascript method to find all the index values that contain the odd numbers. If there is no odd number, then the return value will be -1.
Output:
Explanation: In the above example, we have declared a var array inside, in which 5 elements are mentioned. Then, we declared the testing function where we kept the condition that the element should be greater than 25 ( element > 25 ). After that, we have to use the document.write operator to print the output, which is all the index numbers of the elements greater than 25.
Supported Browsers
Almost all modern browsers support the javascript array findindex() method. The names of some popular browsers that support the array findindex() method are given below.
Browser Name | Supported on versions |
---|---|
Mozilla Firefox | 25.0 |
Microsoft Edge | 12.0 |
Google Chrome | 45 |
Safari | 7.1 |
Opera | 32.0 |
Conclusion
- The array findindex() javascript method is used to find the first element in an array.
- The return value of the findindex() method is the index of the element if the element satisfies the condition. If the condition does not satisfy any elements in the array, the findindex Javascript method returns the value -1. Various modern browsers, such as Edge and Chrome, support the findindex() Javascript method.