First and Last Digit of a Number in Java
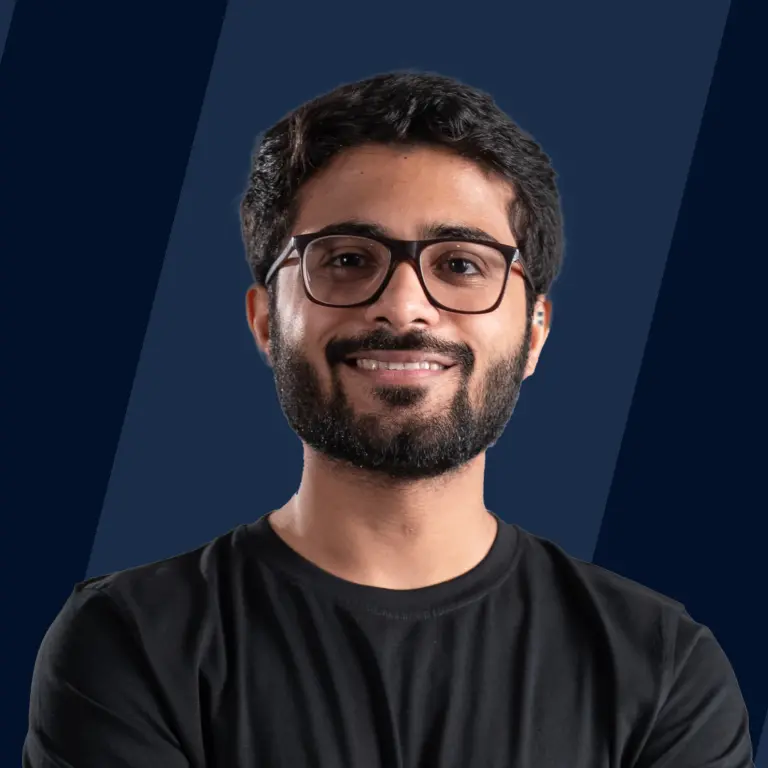
Overview
The last digit of a number can be calculated using the modulo operator only. We can use different approaches to find the first digit, such as dividing by where numDigits is the count of digits in the input number. We can also use the while loop with the modulo operator to find the first digit.
In this article, we will dive deep into various Java programs to find the first and last digits of a number. We will use the while loop, log(), and pow()methods to do this.
Introduction
In this article, we shall explore three approaches to finding a number's first and last digits in Java. We shall cover the concepts of while loop, log() and pow() methods to do the same. The theoretical knowledge we studied in the Java language will be put to use while we execute the source code for writing a program.
Before discussing three methods for printing a number's first and last digits, let's understand the algorithm we will follow.
Step 1: We can either take the input number from the user or give it in the code itself.
Step 2: Build two variables, firstDigit and lastDigit, and initialize both to 0.
Step 3: We will find the' lastDigit' Using the modulo operator(%).
Step 4: Using the while loop, Math.log(), and Math.pow() methods (discussed separately below), we can calculate the firstDigit.
Step 5: Print the values of firstDigit and lastDigit variables.
Now we have understood the flow that will be followed in each of these programs, let us dive into each program to do the same.
Example: Using while loop
Output:
Explanation:
- Here, we stored the input in the number variable, whose first and last digits need to be calculated.
- Using the modulo operator, we calculated the number lastDigit. We then used the while loop and modulo operator again to get the first digit of the number.
- The firstDigit variable will store the result when the while loop terminates. It updates each time and contains the last digit until the number has any digits left.
Example: Using log() and pow() methods
Output:
Explanation:
- Here, we start by directly providing the input in the number variable. Then, we use the modulo operator for the last digit of the number.
- To calculate the first digit, we used built-in methods Math.log() and Math.pow() belonging to the Math class.
- We used the log() method to determine the number of digits in the input number. We have also used the Math.max() method to ensure that the number of digits is at least one.
- After this, we have used the pow() method to get the divisor value. This divisor value was used to get the first digit of the number subsequently.
Example: Using while loop and pow() method
Output:
Explanation:
-
- Here, we start by directly providing the input in the number variable. Then, we use the modulo operator for the last digit of the number.
- Then, we used the while loop instead of the log() method (as in the above example ) to calculate the number of digits, which is stored in the count variable.
- We have used the Math.max() method to ensure that the number of digits is atleast one.
- For the first digit of the number, we calculate the number of digits using the while loop and then obtain our divisor value using the pow() method.
- This divisor value was used to get the first digit of the number subsequently.
Conclusion
- The first and last digits of a number can be calculated in three different ways:
- Using while loop
- Using log() and pow() methods
- Using while loop and pow() method
- The trick to finding the last digit is simply taking number modulo 10. The first digit can be found in various ways, as discussed.
- To handle negative numbers, either take the absolute value of the input or first and last digits.
- To handle the special case of zero, use the Math.max() method wherever suitable.