Fizz Buzz Program in Java
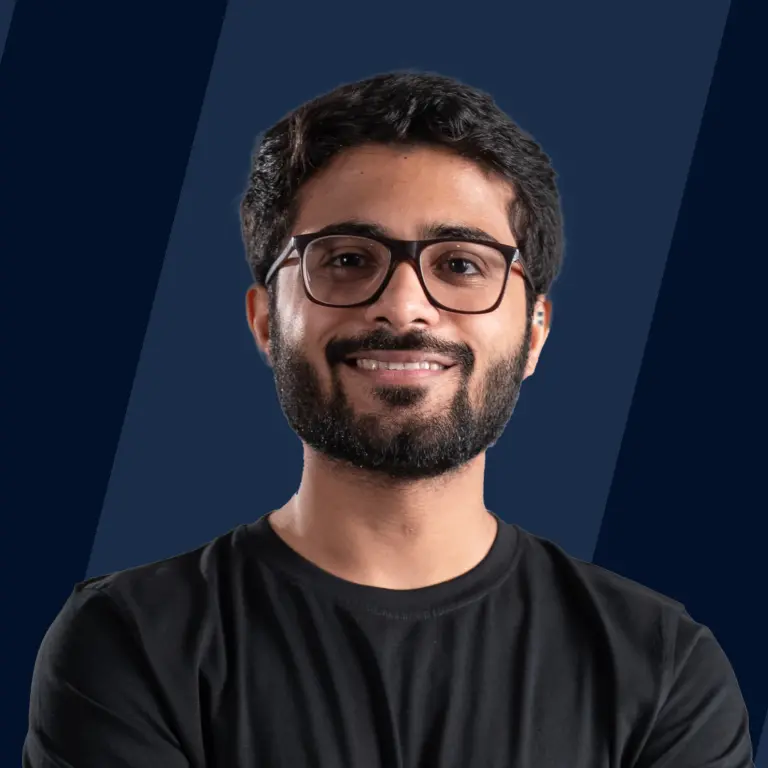
Overview
This fizz buzz program in Java is a very popular programming question asked in interviews to test the basic knowledge of the candidate. It is a beginner-level problem. It would be a good programming question to develop a coding sense in the beginners who are just starting with programming.
The Fizz Buzz program is a classic coding challenge that is often used as a fun and educational tool to assess basic programming skills. In this challenge, the program is given a number , and the objective is to iterate through the numbers from to and perform specific actions based on the value of each number. The actions include printing Fizz for multiples of , Buzz for multiples of , and FizzBuzz for multiples of both 3 and 5. This game provides an opportunity to practice looping, conditional statements, and basic arithmetic operations in a practical and engaging way.
Introduction to the FizzBuzz Program
The FizzBuzz program in Java is a fun game that is used to print certain outputs like "Fizz", "Buzz", or "FizzBuzz" based on some conditions.
In this program, we are given a set of numbers, say, 1 to 20. We are supposed to check each number between 1 to 20 whether it is divisible by 3 or 5 or by both. Based on the results of this divisibility operation, we will print "Fizz" if the number is divisible by 3, "Buzz" if it is divisible by 5, and "FizzBuzz" if it is divisible by both 3 and 5.
Moreover, if the number does not satisfy any of the three conditions specified above, then we will print the number itself.
Example:
Rules of the Game
The following rules should be followed while playing the fizz buzz game.
- If the number is divisible by both 3 and 5 then we will print "FizzBuzz" as the output.
- If the number is divisible by 3 then we will print "Fizz" as the output.
- If the number is divisible by 5 then we will print "Buzz" as the output.
- However, if none of the three conditions is satisfied then we will print the number itself as the output.
FizzBuzz Program in Java
There are several ways in which the FizzBuzz program in Java can be solved. Let us see them in detail.
Simple FizzBuzz Java Solution
In this program, we will run a loop from 1 to the number given by the user as the input, after which we will be checking the three conditions of the fizz buzz game on each number and print the result accordingly.
Let us see the code for a simple FizzBuzz program in Java.
Output: The output is given for n =
The time complexity of the above solution will be O(N) as we are running a single loop to traverse the numbers whereas the space complexity of the above solution would be constant O(1) as we are not using any extra space in our program.
- Time Complexity:
- Space Complexity:
Using Java 8
In this method, we will be using IntStream Interface provided by Java 8. The IntStream Interface provides two methods that we will be used for solving the fizz buzz program in Java.
The first method is the rangeClosed() method which takes two parameters, a starting index and an ending index and generates the numbers between the starting and the ending index by incrementing each number by . It is a static method.
Syntax:
The second method is the mapToObj() method which is discussed further.
Using mapToObj() Method
The second method is the mapToObj() method which returns an object-valued stream. This stream has the results obtained by applying the given conditions to the elements of this stream.
Syntax:
Now let us use these functions to solve our FizzBuzz program in Java.
Output: The output is given for n =
In the above program, we have used the rangeClosed() method of IntStream Interface to traverse the numbers and the mapToObj() method to check the respective conditions on the stream of elements.
However, using the ternary operator in place of the if-else conditions has made our code shorter as compared to the previous one.
- Time Complexity: O(N)
- Space Complexity: O(N)
Conclusion
- The FizzBuzz program is a simple game and a beginner-level problem to test the logic-building skills of the candidate.
- In this program, we need to check each number for its divisibility with 3, 5, or with both 3 and 5.
- If the given number would be divisible by 3, "Fizz" would be printed as the output, if the number is divisible by 5, "Buzz" would be printed.
- If the number is divisible by both 3 and 5, "FizzBuzz" would be printed. However, if none of the conditions is satisfied then the number itself is printed as the output.
- This problem can be solved using if-else and ternary operator.
- We can use the mapToObj() and rangeClosed() methods of the IntStream Interface of Java 8 for solving this problem.