How to Flatten List in Python?
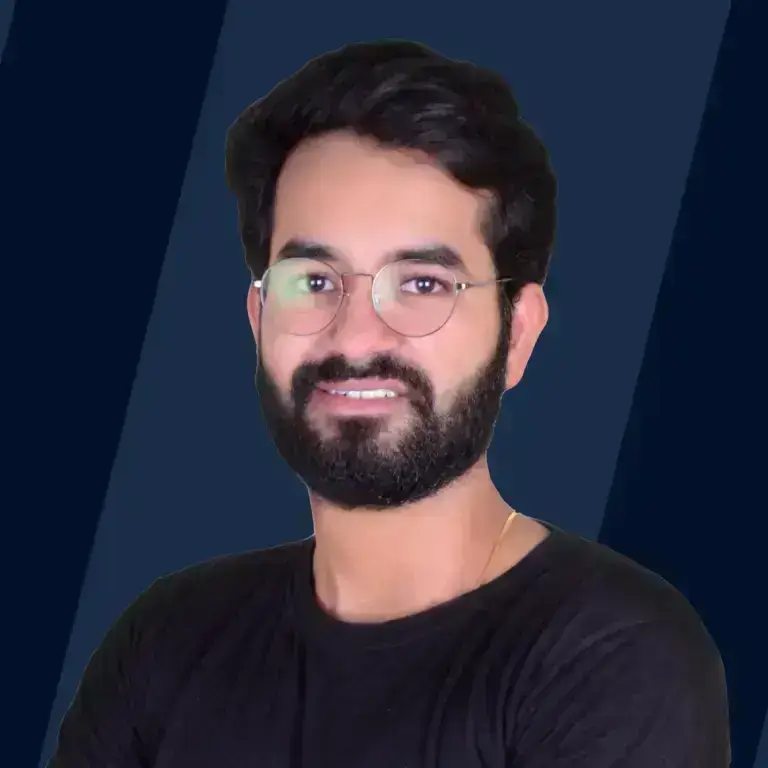
While dealing with Python, we often encounter nested lists. To perform operations on these lists, it's essential to flatten them. Flattening a list in Python means transforming a nested list into a one-dimensional array-like list.
This process is crucial when we work with lists that contain other lists in a cascaded format, similar to a two-dimensional array.
Unlike Java or C++, which have built-in methods like flatMap() or forEach() for this purpose, Python requires us to manually flatten the list to ensure all elements of sublists are included in a single, one-dimensional list.
For instance, consider the nested list [[1, 2, [3]], 4]. To flatten this list in Python, we aim to achieve the output [1, 2, 3, 4], where all elements, regardless of their depth in the original nested structure, are part of the final, flattened list.
Different Ways to Flatten List in Python
Now we shall be learning five ways in which we can Flatten a list in Python with its algorithm, syntax, and code example along with output and the elaborative explanation to under each concept better.
Using List Comprehension
List comprehension in Python allows us to create a new list based on values from an existing list. It involves iterating through each element of the list using a for loop, resulting in a flattened list. This syntax is concise and convenient.
Code:
Output:
Using Nested Loops
Intro: To flatten a list in Python using nested for loops, iterate through each element of the list and append it to a new, flattened list. This is the most intuitive approach to flattening a list in Python, as it involves picking each item from the sublist of the list and putting it in the new, empty flattened list.
Code:
Output:
Using Itertool Package
Intro: To flatten a list in Python using the itertools package, import the package and use its chain function to flatten the list. This works for regular and irregular lists, even if they contain nested sublists. The chain function treats each consecutive sequence as a single sequence, allowing you to iterate through the elements of the list sequentially and create a one-dimensional flattened list in Python.
Syntax: The syntax to use the itertools package is given below:
Parameters: The parameter that needs to be passed to start using the ietertools in Python is only the list that needs to flatten as in the syntax given above its the Original_list.
Return Value: The return value of implementing the itertools in Python is the flatten list that you get.
Quick Note: The chain() method from the itertools package returns each item of each list or sub-lists.
Code:
Output:
Using Sum() Function
Intro: You might now get surprised to know the magic of all the different ways we learned so far to flatten lists in Python. Python has this built-in function known as the sum() method which can also be used to create a flatten list in Python. What is more magical about this is, this is the easiest and quickest way to convert your super nested list into a flatten list in Python. It happens by simply passing the nested list as an argument in addition to the empty list. It then magically converts your nested list into a flatten list in Python.
Syntax: The syntax used for the sum() method to flatten lists in Python is as below:
Parameters: The parameter that needs to be passed to start using the sum() method in Python is the original list which needs to be flattened along with a square bracket to indicate an empty list where all the elements of the super nested original list will be set in one-dimensional returned as flatten list.
Return Value: The return value of implementing the sum() method in Python is the flattened list that you get.
Code:
Output:
Using Lambda and Reduce()
Intro: Lastly, we now shall be diving deep into how we can use the lambda and reduce() method to flatten a list in Python. We define a lambda method that has only one expression but can take any number of arguments. Also, the functools module offers functions that can work with other functions. Here we use or extend with the help of callable objects without the hassle of completely rewriting them.
To flatten the list in Python, we give the function itself with two arguments passed as the first argument to the reduce() method whereas the second argument is the original super nested list. Then the argument function gets applied cumulatively to all the items in the original list.
Syntax: The syntax use of the lambda and reduce() method to flatten lits in Python is as below:
Parameters: We pass the lambda function itself with two arguments as the first argument of the reduce() function from the functools package. And original super nested list as the second argument.
Return Value: The return value of implementing the lambda and reduce() method in Python is the flattened list that you get.
Code:
Output:
Using flatten() method
Intro: The flatten() method is often associated with libraries such as NumPy, which provide the capability to flatten arrays. This method is straightforward and efficient, especially for numerical data or when working with arrays.
Syntax: The syntax used for the flatten() method to flatten lists in Python is as below:
Parameters:
- numpy_array: The NumPy array that you want to flatten. It should be created from the nested list that needs to be flattened.
Return Value: The flatten() method returns a new one-dimensional array that contains all the elements of the input array, flattened.
Code:
Output:
Using chain() with isinstance()
Intro: The combination of itertools.chain() and isinstance() provides a dynamic approach to flatten lists, especially useful for irregularly nested lists. This method iterates through each element and checks if it's a list. If so, it flattens it; otherwise, it leaves the element as is. This ensures a thorough flattening process, accommodating various nesting levels.
Syntax: The syntax used for this method to flatten lists in Python is as below:
Parameters:
- nested_list: The nested list that you want to flatten. It can contain elements of various data types, including other lists.
Return Value: This method returns a new list where all elements from the nested list are flattened into a one-dimensional structure.
Code:
Output:
Using recursion
Intro: Recursion is a powerful technique for flattening deeply nested lists or lists with irregular nesting structures. This method involves defining a function that calls itself to process each element of the list. If an element is a list, the function recursively flattens it; otherwise, it yields the element directly.
Syntax: The syntax used for this method to flatten lists in Python is as below:
Parameters:
- nested_list: The deeply nested or irregularly nested list that you want to flatten.
Return Value: The recursive function yields a generator that can be converted to a list, containing all elements from the nested list flattened into a one-dimensional structure.
Code:
Output:
Conclusion
- We need to flatten list Python, to perform any operations in Python the list needs to be a one-dimensional array.
- The chain() method from the itertools package returns each item of each list or sub-lists.
- We have two types of nested lists:
- Irregular nested list: A list consisting of elements that are not of the same datatype. For example, a list containing integers as well as strings.
- Regular nested list: A list consisting of elements that are having the same data type. For example, a list containing all integers or all strings.
Related Programs in Python
Some of the cool projects in Python which can help you understand concepts of Python a lot deeper along with having fun programming in Python are given below: