What is Float in C++?
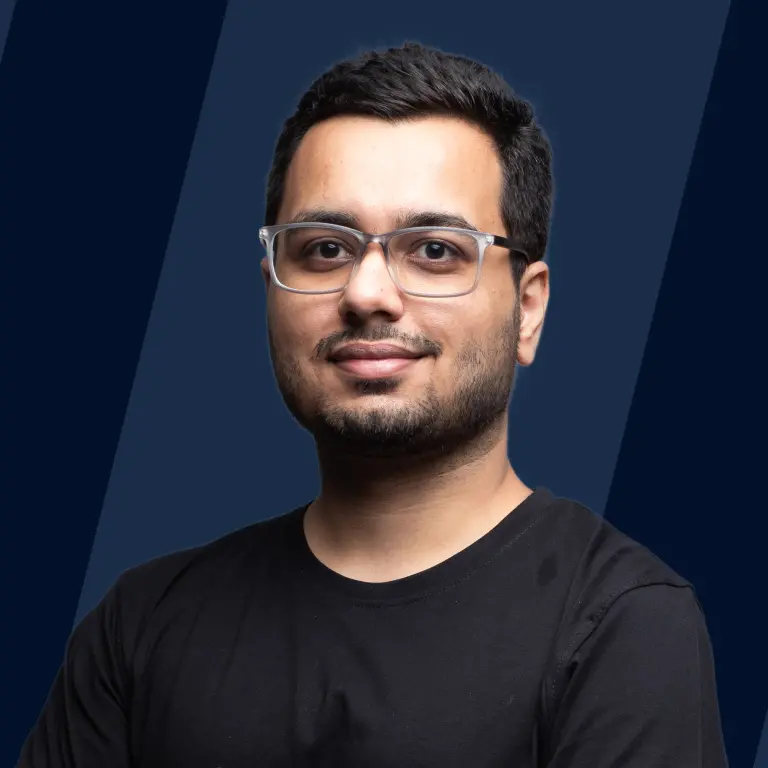
The float in C++ is the fundamental primitive built-in data type. Float is the term used for the numerical implementation of floating point numbers. The memory size of float in C++ is 32 bits(i.e. 4 bytes), and there is a variety of functions to manipulate and work with the floating-point numbers.
The float in C++ has a precision only up to the 7 digits, if we exceed its precision limit then it displays the garbage values after the precision limit. The range of float type in the C++ can represents values ranging from approximately to . If we want to store the number with higher precision values than the given range of float, then we can use the double which has a higher range than the float.
Uses of Float
The float values are better in performance as compared to the double values. Processing of float values is faster due to their internal implementation, float value consumes half of the memory bandwidth when compared with the double, due to this reason it is a good decision to use the float more often when the requirement is equal to or less than of 7 digit precision, over the double.
As graphic libraries require extremely high-demand processing power, float values are also preferred in the graphics library due to their performance advantage over double values.
Programmers also prefer to store the records of currencies in the float values due to their ability to define the number of decimal places with some additional parameters.
Float vs Double and Int
Float values and double values both serve the same purpose of storing the floating point values. The main difference between this two is their range and memory size. The size of double in C++ is 64 bits(i.e. 8 bytes) which is twice of float data type. If we want to store the values with a precision of 15 to 16 decimal numbers then double is the preferred choice over the float in C++.
The int is known as the built-in integer data type which is used to store the whole numbers. It serves a completely different purpose than the float. The size of int in C++ is 32 bits(i.e. 4 bytes).
Example 1: Float in C++
Consider the following example where the variable of type float is created and printed using C++.
Coding Example -
Output -
In the above program, we declared the variable with type float with the name numFloat and assigned the floating value 5.6937 to it. Next, we printed the value inside this numFloat variable. This code demonstrates the basic use of float in C++.
setprecision() Function
In C++, we can use the setprecision() function to specify the number of values with the decimal point in our floating point values to print with the cout. Below are examples of some floating point numbers with the precision number -
The setprecision() function is the part of the iomanip library in C++ that we need to import into our code. The iomanip stands for input-output manipulation.
Example 2: Using setprecision() for Floating-Point Numbers
Below is the coding example to show the use of the setprecision() function in the program to set the number of values with the decimal point in our program.
Code Example -
Output -
Consider the above coding example, where we used the setprecision() method from the iomanip library to set the number of values of a number to print it with the cout. As you can see in the above example, we assigned the variable numFloat with the value 15.6397. Here if we passed value 4 as an argument with the setprecision() function, then we get the output as 15.64 consists of 4 digits, the given value is generated by rounding off the number. If we passed the value as 3 as an argument then we get the output as 15.6 which is the value with 3 digits of precision as you can see in the output. So these are the ways we can use to set the precision for values of float in C++. Use this online Compiler to compile your C++ code.
Work with Exponential Numbers
We can use the float in C++ to represent the exponential numbers. For example, to represent 21000 (i.e. ) can be stored as follows.
This format of numbers is called scientific format where we use e+ to denote the exponential. The number before e+ is the base, whereas the number after e+ is the exponent of the given number.
This scientific format is also used to showcase the numbers which are out of precision range. As we saw earlier the precision range for float values is 7 digits so the numbers above this are stored in the scientific format. For example, the number 2100000 stored is displayed as the 2.1e+06. here the base value is 2.1 and the exponent value is 6.
Code Example -
Output -
As you can see in the above coding example, here the demonstration of the scientific format is given with two examples. First of all numFloat variable of type float is declared and assigned with the scientific format number which comes out to be 21000. In the second initialization, the same variable numFloat is assigned with a value greater than the range of float type, when printed we can see that it is stored in the scientific format.
Elevate your career prospects with our Free C++ certification course. Enroll today and acquire skills that will set you apart in the job market.
Conclusion
- Float is one of the fundamental in-built datatypes in C++ which is used the store the floating point values.
- The float in C++ ranges from approximately to the precision of the float values is 7 digits as we saw above.
- We can use the setprecision function from the iomanip library in C++ to set the precision of the number of digits, for a given value while printing with the cout.
- Exponential values can also be represented with the float in C++. Here the scientific format representation is used to indicate the base and the exponent value in the given number.